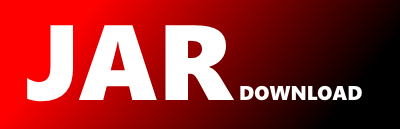
com.pulumi.aws.cognito.outputs.GetUserPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cognito.outputs;
import com.pulumi.aws.cognito.outputs.GetUserPoolAccountRecoverySetting;
import com.pulumi.aws.cognito.outputs.GetUserPoolAdminCreateUserConfig;
import com.pulumi.aws.cognito.outputs.GetUserPoolDeviceConfiguration;
import com.pulumi.aws.cognito.outputs.GetUserPoolEmailConfiguration;
import com.pulumi.aws.cognito.outputs.GetUserPoolLambdaConfig;
import com.pulumi.aws.cognito.outputs.GetUserPoolSchemaAttribute;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetUserPoolResult {
private List accountRecoverySettings;
private List adminCreateUserConfigs;
/**
* @return ARN of the User Pool.
* * account_recovery_setting - The available verified method a user can use to recover their password when they call ForgotPassword. You can use this setting to define a preferred method when a user has more than one method available. With this setting, SMS doesn't qualify for a valid password recovery mechanism if the user also has SMS multi-factor authentication (MFA) activated. In the absence of this setting, Amazon Cognito uses the legacy behavior to determine the recovery method where SMS is preferred through email.
* * admin_create_user_config - The configuration for AdminCreateUser requests.
*
*/
private String arn;
/**
* @return The attributes that are auto-verified in a user pool.
*
*/
private List autoVerifiedAttributes;
/**
* @return The date and time, in ISO 8601 format, when the item was created.
*
*/
private String creationDate;
/**
* @return A custom domain name that you provide to Amazon Cognito. This parameter applies only if you use a custom domain to host the sign-up and sign-in pages for your application. An example of a custom domain name might be auth.example.com.
*
*/
private String customDomain;
/**
* @return When active, DeletionProtection prevents accidental deletion of your user pool. Before you can delete a user pool that you have protected against deletion, you must deactivate this feature.
* * device_configuration - The device-remembering configuration for a user pool. A null value indicates that you have deactivated device remembering in your user pool.
*
*/
private String deletionProtection;
private List deviceConfigurations;
/**
* @return The domain prefix, if the user pool has a domain associated with it.
* * email_configuration - The email configuration of your user pool. The email configuration type sets your preferred sending method, AWS Region, and sender for messages from your user pool.
*
*/
private String domain;
private List emailConfigurations;
/**
* @return A number estimating the size of the user pool.
* * lambda_config - The AWS Lambda triggers associated with the user pool.
*
*/
private Integer estimatedNumberOfUsers;
private String id;
private List lambdaConfigs;
/**
* @return The date and time, in ISO 8601 format, when the item was modified.
*
*/
private String lastModifiedDate;
/**
* @return Can be one of the following values: `OFF` | `ON` | `OPTIONAL`
*
*/
private String mfaConfiguration;
/**
* @return - Name of the attribute.
*
*/
private String name;
private List schemaAttributes;
/**
* @return The contents of the SMS authentication message.
*
*/
private String smsAuthenticationMessage;
/**
* @return The reason why the SMS configuration can't send the messages to your users.
*
*/
private String smsConfigurationFailure;
/**
* @return The contents of the SMS authentication message.
*
*/
private String smsVerificationMessage;
/**
* @return Map of tags assigned to the resource.
*
*/
private Map tags;
private String userPoolId;
/**
* @return (Deprecated) Map of tags assigned to the resource.
*
* @deprecated
* Use the attribute "tags" instead
*
*/
@Deprecated /* Use the attribute ""tags"" instead */
private Map userPoolTags;
/**
* @return Specifies whether a user can use an email address or phone number as a username when they sign up.
*
*/
private List usernameAttributes;
private GetUserPoolResult() {}
public List accountRecoverySettings() {
return this.accountRecoverySettings;
}
public List adminCreateUserConfigs() {
return this.adminCreateUserConfigs;
}
/**
* @return ARN of the User Pool.
* * account_recovery_setting - The available verified method a user can use to recover their password when they call ForgotPassword. You can use this setting to define a preferred method when a user has more than one method available. With this setting, SMS doesn't qualify for a valid password recovery mechanism if the user also has SMS multi-factor authentication (MFA) activated. In the absence of this setting, Amazon Cognito uses the legacy behavior to determine the recovery method where SMS is preferred through email.
* * admin_create_user_config - The configuration for AdminCreateUser requests.
*
*/
public String arn() {
return this.arn;
}
/**
* @return The attributes that are auto-verified in a user pool.
*
*/
public List autoVerifiedAttributes() {
return this.autoVerifiedAttributes;
}
/**
* @return The date and time, in ISO 8601 format, when the item was created.
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return A custom domain name that you provide to Amazon Cognito. This parameter applies only if you use a custom domain to host the sign-up and sign-in pages for your application. An example of a custom domain name might be auth.example.com.
*
*/
public String customDomain() {
return this.customDomain;
}
/**
* @return When active, DeletionProtection prevents accidental deletion of your user pool. Before you can delete a user pool that you have protected against deletion, you must deactivate this feature.
* * device_configuration - The device-remembering configuration for a user pool. A null value indicates that you have deactivated device remembering in your user pool.
*
*/
public String deletionProtection() {
return this.deletionProtection;
}
public List deviceConfigurations() {
return this.deviceConfigurations;
}
/**
* @return The domain prefix, if the user pool has a domain associated with it.
* * email_configuration - The email configuration of your user pool. The email configuration type sets your preferred sending method, AWS Region, and sender for messages from your user pool.
*
*/
public String domain() {
return this.domain;
}
public List emailConfigurations() {
return this.emailConfigurations;
}
/**
* @return A number estimating the size of the user pool.
* * lambda_config - The AWS Lambda triggers associated with the user pool.
*
*/
public Integer estimatedNumberOfUsers() {
return this.estimatedNumberOfUsers;
}
public String id() {
return this.id;
}
public List lambdaConfigs() {
return this.lambdaConfigs;
}
/**
* @return The date and time, in ISO 8601 format, when the item was modified.
*
*/
public String lastModifiedDate() {
return this.lastModifiedDate;
}
/**
* @return Can be one of the following values: `OFF` | `ON` | `OPTIONAL`
*
*/
public String mfaConfiguration() {
return this.mfaConfiguration;
}
/**
* @return - Name of the attribute.
*
*/
public String name() {
return this.name;
}
public List schemaAttributes() {
return this.schemaAttributes;
}
/**
* @return The contents of the SMS authentication message.
*
*/
public String smsAuthenticationMessage() {
return this.smsAuthenticationMessage;
}
/**
* @return The reason why the SMS configuration can't send the messages to your users.
*
*/
public String smsConfigurationFailure() {
return this.smsConfigurationFailure;
}
/**
* @return The contents of the SMS authentication message.
*
*/
public String smsVerificationMessage() {
return this.smsVerificationMessage;
}
/**
* @return Map of tags assigned to the resource.
*
*/
public Map tags() {
return this.tags;
}
public String userPoolId() {
return this.userPoolId;
}
/**
* @return (Deprecated) Map of tags assigned to the resource.
*
* @deprecated
* Use the attribute "tags" instead
*
*/
@Deprecated /* Use the attribute ""tags"" instead */
public Map userPoolTags() {
return this.userPoolTags;
}
/**
* @return Specifies whether a user can use an email address or phone number as a username when they sign up.
*
*/
public List usernameAttributes() {
return this.usernameAttributes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetUserPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List accountRecoverySettings;
private List adminCreateUserConfigs;
private String arn;
private List autoVerifiedAttributes;
private String creationDate;
private String customDomain;
private String deletionProtection;
private List deviceConfigurations;
private String domain;
private List emailConfigurations;
private Integer estimatedNumberOfUsers;
private String id;
private List lambdaConfigs;
private String lastModifiedDate;
private String mfaConfiguration;
private String name;
private List schemaAttributes;
private String smsAuthenticationMessage;
private String smsConfigurationFailure;
private String smsVerificationMessage;
private Map tags;
private String userPoolId;
private Map userPoolTags;
private List usernameAttributes;
public Builder() {}
public Builder(GetUserPoolResult defaults) {
Objects.requireNonNull(defaults);
this.accountRecoverySettings = defaults.accountRecoverySettings;
this.adminCreateUserConfigs = defaults.adminCreateUserConfigs;
this.arn = defaults.arn;
this.autoVerifiedAttributes = defaults.autoVerifiedAttributes;
this.creationDate = defaults.creationDate;
this.customDomain = defaults.customDomain;
this.deletionProtection = defaults.deletionProtection;
this.deviceConfigurations = defaults.deviceConfigurations;
this.domain = defaults.domain;
this.emailConfigurations = defaults.emailConfigurations;
this.estimatedNumberOfUsers = defaults.estimatedNumberOfUsers;
this.id = defaults.id;
this.lambdaConfigs = defaults.lambdaConfigs;
this.lastModifiedDate = defaults.lastModifiedDate;
this.mfaConfiguration = defaults.mfaConfiguration;
this.name = defaults.name;
this.schemaAttributes = defaults.schemaAttributes;
this.smsAuthenticationMessage = defaults.smsAuthenticationMessage;
this.smsConfigurationFailure = defaults.smsConfigurationFailure;
this.smsVerificationMessage = defaults.smsVerificationMessage;
this.tags = defaults.tags;
this.userPoolId = defaults.userPoolId;
this.userPoolTags = defaults.userPoolTags;
this.usernameAttributes = defaults.usernameAttributes;
}
@CustomType.Setter
public Builder accountRecoverySettings(List accountRecoverySettings) {
if (accountRecoverySettings == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "accountRecoverySettings");
}
this.accountRecoverySettings = accountRecoverySettings;
return this;
}
public Builder accountRecoverySettings(GetUserPoolAccountRecoverySetting... accountRecoverySettings) {
return accountRecoverySettings(List.of(accountRecoverySettings));
}
@CustomType.Setter
public Builder adminCreateUserConfigs(List adminCreateUserConfigs) {
if (adminCreateUserConfigs == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "adminCreateUserConfigs");
}
this.adminCreateUserConfigs = adminCreateUserConfigs;
return this;
}
public Builder adminCreateUserConfigs(GetUserPoolAdminCreateUserConfig... adminCreateUserConfigs) {
return adminCreateUserConfigs(List.of(adminCreateUserConfigs));
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder autoVerifiedAttributes(List autoVerifiedAttributes) {
if (autoVerifiedAttributes == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "autoVerifiedAttributes");
}
this.autoVerifiedAttributes = autoVerifiedAttributes;
return this;
}
public Builder autoVerifiedAttributes(String... autoVerifiedAttributes) {
return autoVerifiedAttributes(List.of(autoVerifiedAttributes));
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder customDomain(String customDomain) {
if (customDomain == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "customDomain");
}
this.customDomain = customDomain;
return this;
}
@CustomType.Setter
public Builder deletionProtection(String deletionProtection) {
if (deletionProtection == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "deletionProtection");
}
this.deletionProtection = deletionProtection;
return this;
}
@CustomType.Setter
public Builder deviceConfigurations(List deviceConfigurations) {
if (deviceConfigurations == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "deviceConfigurations");
}
this.deviceConfigurations = deviceConfigurations;
return this;
}
public Builder deviceConfigurations(GetUserPoolDeviceConfiguration... deviceConfigurations) {
return deviceConfigurations(List.of(deviceConfigurations));
}
@CustomType.Setter
public Builder domain(String domain) {
if (domain == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "domain");
}
this.domain = domain;
return this;
}
@CustomType.Setter
public Builder emailConfigurations(List emailConfigurations) {
if (emailConfigurations == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "emailConfigurations");
}
this.emailConfigurations = emailConfigurations;
return this;
}
public Builder emailConfigurations(GetUserPoolEmailConfiguration... emailConfigurations) {
return emailConfigurations(List.of(emailConfigurations));
}
@CustomType.Setter
public Builder estimatedNumberOfUsers(Integer estimatedNumberOfUsers) {
if (estimatedNumberOfUsers == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "estimatedNumberOfUsers");
}
this.estimatedNumberOfUsers = estimatedNumberOfUsers;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lambdaConfigs(List lambdaConfigs) {
if (lambdaConfigs == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "lambdaConfigs");
}
this.lambdaConfigs = lambdaConfigs;
return this;
}
public Builder lambdaConfigs(GetUserPoolLambdaConfig... lambdaConfigs) {
return lambdaConfigs(List.of(lambdaConfigs));
}
@CustomType.Setter
public Builder lastModifiedDate(String lastModifiedDate) {
if (lastModifiedDate == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "lastModifiedDate");
}
this.lastModifiedDate = lastModifiedDate;
return this;
}
@CustomType.Setter
public Builder mfaConfiguration(String mfaConfiguration) {
if (mfaConfiguration == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "mfaConfiguration");
}
this.mfaConfiguration = mfaConfiguration;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder schemaAttributes(List schemaAttributes) {
if (schemaAttributes == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "schemaAttributes");
}
this.schemaAttributes = schemaAttributes;
return this;
}
public Builder schemaAttributes(GetUserPoolSchemaAttribute... schemaAttributes) {
return schemaAttributes(List.of(schemaAttributes));
}
@CustomType.Setter
public Builder smsAuthenticationMessage(String smsAuthenticationMessage) {
if (smsAuthenticationMessage == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "smsAuthenticationMessage");
}
this.smsAuthenticationMessage = smsAuthenticationMessage;
return this;
}
@CustomType.Setter
public Builder smsConfigurationFailure(String smsConfigurationFailure) {
if (smsConfigurationFailure == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "smsConfigurationFailure");
}
this.smsConfigurationFailure = smsConfigurationFailure;
return this;
}
@CustomType.Setter
public Builder smsVerificationMessage(String smsVerificationMessage) {
if (smsVerificationMessage == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "smsVerificationMessage");
}
this.smsVerificationMessage = smsVerificationMessage;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder userPoolId(String userPoolId) {
if (userPoolId == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "userPoolId");
}
this.userPoolId = userPoolId;
return this;
}
@CustomType.Setter
public Builder userPoolTags(Map userPoolTags) {
if (userPoolTags == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "userPoolTags");
}
this.userPoolTags = userPoolTags;
return this;
}
@CustomType.Setter
public Builder usernameAttributes(List usernameAttributes) {
if (usernameAttributes == null) {
throw new MissingRequiredPropertyException("GetUserPoolResult", "usernameAttributes");
}
this.usernameAttributes = usernameAttributes;
return this;
}
public Builder usernameAttributes(String... usernameAttributes) {
return usernameAttributes(List.of(usernameAttributes));
}
public GetUserPoolResult build() {
final var _resultValue = new GetUserPoolResult();
_resultValue.accountRecoverySettings = accountRecoverySettings;
_resultValue.adminCreateUserConfigs = adminCreateUserConfigs;
_resultValue.arn = arn;
_resultValue.autoVerifiedAttributes = autoVerifiedAttributes;
_resultValue.creationDate = creationDate;
_resultValue.customDomain = customDomain;
_resultValue.deletionProtection = deletionProtection;
_resultValue.deviceConfigurations = deviceConfigurations;
_resultValue.domain = domain;
_resultValue.emailConfigurations = emailConfigurations;
_resultValue.estimatedNumberOfUsers = estimatedNumberOfUsers;
_resultValue.id = id;
_resultValue.lambdaConfigs = lambdaConfigs;
_resultValue.lastModifiedDate = lastModifiedDate;
_resultValue.mfaConfiguration = mfaConfiguration;
_resultValue.name = name;
_resultValue.schemaAttributes = schemaAttributes;
_resultValue.smsAuthenticationMessage = smsAuthenticationMessage;
_resultValue.smsConfigurationFailure = smsConfigurationFailure;
_resultValue.smsVerificationMessage = smsVerificationMessage;
_resultValue.tags = tags;
_resultValue.userPoolId = userPoolId;
_resultValue.userPoolTags = userPoolTags;
_resultValue.usernameAttributes = usernameAttributes;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy