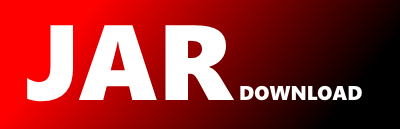
com.pulumi.aws.datazone.inputs.EnvironmentState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.datazone.inputs;
import com.pulumi.aws.datazone.inputs.EnvironmentLastDeploymentArgs;
import com.pulumi.aws.datazone.inputs.EnvironmentProvisionedResourceArgs;
import com.pulumi.aws.datazone.inputs.EnvironmentTimeoutsArgs;
import com.pulumi.aws.datazone.inputs.EnvironmentUserParameterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EnvironmentState extends com.pulumi.resources.ResourceArgs {
public static final EnvironmentState Empty = new EnvironmentState();
/**
* The ID of the Amazon Web Services account where the environment exists
*
*/
@Import(name="accountIdentifier")
private @Nullable Output accountIdentifier;
/**
* @return The ID of the Amazon Web Services account where the environment exists
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy