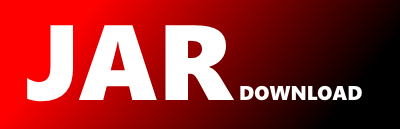
com.pulumi.aws.directconnect.BgpPeerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directconnect;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BgpPeerArgs extends com.pulumi.resources.ResourceArgs {
public static final BgpPeerArgs Empty = new BgpPeerArgs();
/**
* The address family for the BGP peer. ` ipv4 ` or `ipv6`.
*
*/
@Import(name="addressFamily", required=true)
private Output addressFamily;
/**
* @return The address family for the BGP peer. ` ipv4 ` or `ipv6`.
*
*/
public Output addressFamily() {
return this.addressFamily;
}
/**
* The IPv4 CIDR address to use to send traffic to Amazon.
* Required for IPv4 BGP peers on public virtual interfaces.
*
*/
@Import(name="amazonAddress")
private @Nullable Output amazonAddress;
/**
* @return The IPv4 CIDR address to use to send traffic to Amazon.
* Required for IPv4 BGP peers on public virtual interfaces.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy