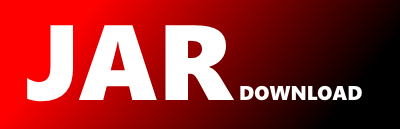
com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dlm.inputs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleCrossRegionCopyRuleArgs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleDeprecateRuleArgs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleFastRestoreRuleArgs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleRetainRuleArgs;
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleShareRuleArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LifecyclePolicyPolicyDetailsScheduleArgs extends com.pulumi.resources.ResourceArgs {
public static final LifecyclePolicyPolicyDetailsScheduleArgs Empty = new LifecyclePolicyPolicyDetailsScheduleArgs();
@Import(name="copyTags")
private @Nullable Output copyTags;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy