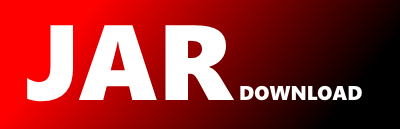
com.pulumi.aws.dms.ReplicationSubnetGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dms;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.dms.ReplicationSubnetGroupArgs;
import com.pulumi.aws.dms.inputs.ReplicationSubnetGroupState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a DMS (Data Migration Service) replication subnet group resource. DMS replication subnet groups can be created, updated, deleted, and imported.
*
* > **Note:** AWS requires a special IAM role called `dms-vpc-role` when using this resource. See the example below to create it as part of your configuration.
*
* ## Example Usage
*
* ### Basic
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.dms.ReplicationSubnetGroup;
* import com.pulumi.aws.dms.ReplicationSubnetGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // Create a new replication subnet group
* var example = new ReplicationSubnetGroup("example", ReplicationSubnetGroupArgs.builder()
* .replicationSubnetGroupDescription("Example replication subnet group")
* .replicationSubnetGroupId("example-dms-replication-subnet-group-tf")
* .subnetIds(
* "subnet-12345678",
* "subnet-12345679")
* .tags(Map.of("Name", "example"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Creating special IAM role
*
* If your account does not already include the `dms-vpc-role` IAM role, you will need to create it to allow DMS to manage subnets in the VPC.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.dms.ReplicationSubnetGroup;
* import com.pulumi.aws.dms.ReplicationSubnetGroupArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dms_vpc_role = new Role("dms-vpc-role", RoleArgs.builder()
* .name("dms-vpc-role")
* .description("Allows DMS to manage VPC")
* .assumeRolePolicy(serializeJson(
* jsonObject(
* jsonProperty("Version", "2012-10-17"),
* jsonProperty("Statement", jsonArray(jsonObject(
* jsonProperty("Effect", "Allow"),
* jsonProperty("Principal", jsonObject(
* jsonProperty("Service", "dms.amazonaws.com")
* )),
* jsonProperty("Action", "sts:AssumeRole")
* )))
* )))
* .build());
*
* var example = new RolePolicyAttachment("example", RolePolicyAttachmentArgs.builder()
* .role(dms_vpc_role.name())
* .policyArn("arn:aws:iam::aws:policy/service-role/AmazonDMSVPCManagementRole")
* .build());
*
* var exampleReplicationSubnetGroup = new ReplicationSubnetGroup("exampleReplicationSubnetGroup", ReplicationSubnetGroupArgs.builder()
* .replicationSubnetGroupDescription("Example")
* .replicationSubnetGroupId("example-id")
* .subnetIds(
* "subnet-12345678",
* "subnet-12345679")
* .tags(Map.of("Name", "example-id"))
* .build(), CustomResourceOptions.builder()
* .dependsOn(example)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import replication subnet groups using the `replication_subnet_group_id`. For example:
*
* ```sh
* $ pulumi import aws:dms/replicationSubnetGroup:ReplicationSubnetGroup test test-dms-replication-subnet-group-tf
* ```
*
*/
@ResourceType(type="aws:dms/replicationSubnetGroup:ReplicationSubnetGroup")
public class ReplicationSubnetGroup extends com.pulumi.resources.CustomResource {
@Export(name="replicationSubnetGroupArn", refs={String.class}, tree="[0]")
private Output replicationSubnetGroupArn;
public Output replicationSubnetGroupArn() {
return this.replicationSubnetGroupArn;
}
/**
* Description for the subnet group.
*
*/
@Export(name="replicationSubnetGroupDescription", refs={String.class}, tree="[0]")
private Output replicationSubnetGroupDescription;
/**
* @return Description for the subnet group.
*
*/
public Output replicationSubnetGroupDescription() {
return this.replicationSubnetGroupDescription;
}
/**
* Name for the replication subnet group. This value is stored as a lowercase string. It must contain no more than 255 alphanumeric characters, periods, spaces, underscores, or hyphens and cannot be `default`.
*
*/
@Export(name="replicationSubnetGroupId", refs={String.class}, tree="[0]")
private Output replicationSubnetGroupId;
/**
* @return Name for the replication subnet group. This value is stored as a lowercase string. It must contain no more than 255 alphanumeric characters, periods, spaces, underscores, or hyphens and cannot be `default`.
*
*/
public Output replicationSubnetGroupId() {
return this.replicationSubnetGroupId;
}
/**
* List of at least 2 EC2 subnet IDs for the subnet group. The subnets must cover at least 2 availability zones.
*
*/
@Export(name="subnetIds", refs={List.class,String.class}, tree="[0,1]")
private Output> subnetIds;
/**
* @return List of at least 2 EC2 subnet IDs for the subnet group. The subnets must cover at least 2 availability zones.
*
*/
public Output> subnetIds() {
return this.subnetIds;
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy