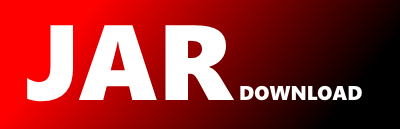
com.pulumi.aws.dynamodb.outputs.TableGlobalSecondaryIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dynamodb.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class TableGlobalSecondaryIndex {
/**
* @return Name of the hash key in the index; must be defined as an attribute in the resource.
*
*/
private String hashKey;
/**
* @return Name of the index.
*
*/
private String name;
/**
* @return Only required with `INCLUDE` as a projection type; a list of attributes to project into the index. These do not need to be defined as attributes on the table.
*
*/
private @Nullable List nonKeyAttributes;
/**
* @return One of `ALL`, `INCLUDE` or `KEYS_ONLY` where `ALL` projects every attribute into the index, `KEYS_ONLY` projects into the index only the table and index hash_key and sort_key attributes , `INCLUDE` projects into the index all of the attributes that are defined in `non_key_attributes` in addition to the attributes that that`KEYS_ONLY` project.
*
*/
private String projectionType;
/**
* @return Name of the range key; must be defined
*
*/
private @Nullable String rangeKey;
/**
* @return Number of read units for this index. Must be set if billing_mode is set to PROVISIONED.
*
*/
private @Nullable Integer readCapacity;
/**
* @return Number of write units for this index. Must be set if billing_mode is set to PROVISIONED.
*
*/
private @Nullable Integer writeCapacity;
private TableGlobalSecondaryIndex() {}
/**
* @return Name of the hash key in the index; must be defined as an attribute in the resource.
*
*/
public String hashKey() {
return this.hashKey;
}
/**
* @return Name of the index.
*
*/
public String name() {
return this.name;
}
/**
* @return Only required with `INCLUDE` as a projection type; a list of attributes to project into the index. These do not need to be defined as attributes on the table.
*
*/
public List nonKeyAttributes() {
return this.nonKeyAttributes == null ? List.of() : this.nonKeyAttributes;
}
/**
* @return One of `ALL`, `INCLUDE` or `KEYS_ONLY` where `ALL` projects every attribute into the index, `KEYS_ONLY` projects into the index only the table and index hash_key and sort_key attributes , `INCLUDE` projects into the index all of the attributes that are defined in `non_key_attributes` in addition to the attributes that that`KEYS_ONLY` project.
*
*/
public String projectionType() {
return this.projectionType;
}
/**
* @return Name of the range key; must be defined
*
*/
public Optional rangeKey() {
return Optional.ofNullable(this.rangeKey);
}
/**
* @return Number of read units for this index. Must be set if billing_mode is set to PROVISIONED.
*
*/
public Optional readCapacity() {
return Optional.ofNullable(this.readCapacity);
}
/**
* @return Number of write units for this index. Must be set if billing_mode is set to PROVISIONED.
*
*/
public Optional writeCapacity() {
return Optional.ofNullable(this.writeCapacity);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TableGlobalSecondaryIndex defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String hashKey;
private String name;
private @Nullable List nonKeyAttributes;
private String projectionType;
private @Nullable String rangeKey;
private @Nullable Integer readCapacity;
private @Nullable Integer writeCapacity;
public Builder() {}
public Builder(TableGlobalSecondaryIndex defaults) {
Objects.requireNonNull(defaults);
this.hashKey = defaults.hashKey;
this.name = defaults.name;
this.nonKeyAttributes = defaults.nonKeyAttributes;
this.projectionType = defaults.projectionType;
this.rangeKey = defaults.rangeKey;
this.readCapacity = defaults.readCapacity;
this.writeCapacity = defaults.writeCapacity;
}
@CustomType.Setter
public Builder hashKey(String hashKey) {
if (hashKey == null) {
throw new MissingRequiredPropertyException("TableGlobalSecondaryIndex", "hashKey");
}
this.hashKey = hashKey;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("TableGlobalSecondaryIndex", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nonKeyAttributes(@Nullable List nonKeyAttributes) {
this.nonKeyAttributes = nonKeyAttributes;
return this;
}
public Builder nonKeyAttributes(String... nonKeyAttributes) {
return nonKeyAttributes(List.of(nonKeyAttributes));
}
@CustomType.Setter
public Builder projectionType(String projectionType) {
if (projectionType == null) {
throw new MissingRequiredPropertyException("TableGlobalSecondaryIndex", "projectionType");
}
this.projectionType = projectionType;
return this;
}
@CustomType.Setter
public Builder rangeKey(@Nullable String rangeKey) {
this.rangeKey = rangeKey;
return this;
}
@CustomType.Setter
public Builder readCapacity(@Nullable Integer readCapacity) {
this.readCapacity = readCapacity;
return this;
}
@CustomType.Setter
public Builder writeCapacity(@Nullable Integer writeCapacity) {
this.writeCapacity = writeCapacity;
return this;
}
public TableGlobalSecondaryIndex build() {
final var _resultValue = new TableGlobalSecondaryIndex();
_resultValue.hashKey = hashKey;
_resultValue.name = name;
_resultValue.nonKeyAttributes = nonKeyAttributes;
_resultValue.projectionType = projectionType;
_resultValue.rangeKey = rangeKey;
_resultValue.readCapacity = readCapacity;
_resultValue.writeCapacity = writeCapacity;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy