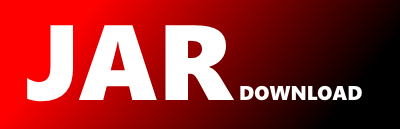
com.pulumi.aws.ec2.SecurityGroupRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.SecurityGroupRuleArgs;
import com.pulumi.aws.ec2.inputs.SecurityGroupRuleState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a security group rule resource. Represents a single `ingress` or `egress` group rule, which can be added to external Security Groups.
*
* > **NOTE:** Avoid using the `aws.ec2.SecurityGroupRule` resource, as it struggles with managing multiple CIDR blocks, and, due to the historical lack of unique IDs, tags and descriptions. To avoid these problems, use the current best practice of the `aws.vpc.SecurityGroupEgressRule` and `aws.vpc.SecurityGroupIngressRule` resources with one CIDR block per rule.
*
* !> **WARNING:** You should not use the `aws.ec2.SecurityGroupRule` resource in conjunction with `aws.vpc.SecurityGroupEgressRule` and `aws.vpc.SecurityGroupIngressRule` resources or with an `aws.ec2.SecurityGroup` resource that has in-line rules. Doing so may cause rule conflicts, perpetual differences, and result in rules being overwritten.
*
* > **NOTE:** Setting `protocol = "all"` or `protocol = -1` with `from_port` and `to_port` will result in the EC2 API creating a security group rule with all ports open. This API behavior cannot be controlled by this provider and may generate warnings in the future.
*
* > **NOTE:** Referencing Security Groups across VPC peering has certain restrictions. More information is available in the [VPC Peering User Guide](https://docs.aws.amazon.com/vpc/latest/peering/vpc-peering-security-groups.html).
*
* ## Example Usage
*
* Basic usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.SecurityGroupRule;
* import com.pulumi.aws.ec2.SecurityGroupRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new SecurityGroupRule("example", SecurityGroupRuleArgs.builder()
* .type("ingress")
* .fromPort(0)
* .toPort(65535)
* .protocol("tcp")
* .cidrBlocks(exampleAwsVpc.cidrBlock())
* .ipv6CidrBlocks(exampleAwsVpc.ipv6CidrBlock())
* .securityGroupId("sg-123456")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Usage With Prefix List IDs
*
* Prefix Lists are either managed by AWS internally, or created by the customer using a
* Managed Prefix List resource. Prefix Lists provided by
* AWS are associated with a prefix list name, or service name, that is linked to a specific region.
*
* Prefix list IDs are exported on VPC Endpoints, so you can use this format:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.VpcEndpoint;
* import com.pulumi.aws.ec2.SecurityGroupRule;
* import com.pulumi.aws.ec2.SecurityGroupRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // ...
* var myEndpoint = new VpcEndpoint("myEndpoint");
*
* var allowAll = new SecurityGroupRule("allowAll", SecurityGroupRuleArgs.builder()
* .type("egress")
* .toPort(0)
* .protocol("-1")
* .prefixListIds(myEndpoint.prefixListId())
* .fromPort(0)
* .securityGroupId("sg-123456")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* You can also find a specific Prefix List using the `aws.ec2.getPrefixList`
* or `ec2_managed_prefix_list` data sources:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.ec2.Ec2Functions;
* import com.pulumi.aws.ec2.inputs.GetPrefixListArgs;
* import com.pulumi.aws.ec2.SecurityGroupRule;
* import com.pulumi.aws.ec2.SecurityGroupRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getRegion();
*
* final var s3 = Ec2Functions.getPrefixList(GetPrefixListArgs.builder()
* .name(String.format("com.amazonaws.%s.s3", current.applyValue(getRegionResult -> getRegionResult.name())))
* .build());
*
* var s3GatewayEgress = new SecurityGroupRule("s3GatewayEgress", SecurityGroupRuleArgs.builder()
* .description("S3 Gateway Egress")
* .type("egress")
* .securityGroupId("sg-123456")
* .fromPort(443)
* .toPort(443)
* .protocol("tcp")
* .prefixListIds(s3.applyValue(getPrefixListResult -> getPrefixListResult.id()))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Import a rule with various IPv4 and IPv6 source CIDR blocks:
*
* Import a rule, applicable to all ports, with a protocol other than TCP/UDP/ICMP/ICMPV6/ALL, e.g., Multicast Transport Protocol (MTP), using the IANA protocol number. For example: 92.
*
* Import a default any/any egress rule to 0.0.0.0/0:
*
* Import an egress rule with a prefix list ID destination:
*
* Import a rule applicable to all protocols and ports with a security group source:
*
* Import a rule that has itself and an IPv6 CIDR block as sources:
*
* __Using `pulumi import` to import__ Security Group Rules using the `security_group_id`, `type`, `protocol`, `from_port`, `to_port`, and source(s)/destination(s) (such as a `cidr_block`) separated by underscores (`_`). All parts are required. For example:
*
* __NOTE:__ Not all rule permissions (e.g., not all of a rule's CIDR blocks) need to be imported for this provider to manage rule permissions. However, importing some of a rule's permissions but not others, and then making changes to the rule will result in the creation of an additional rule to capture the updated permissions. Rule permissions that were not imported are left intact in the original rule.
*
* Import an ingress rule in security group `sg-6e616f6d69` for TCP port 8000 with an IPv4 destination CIDR of `10.0.3.0/24`:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule ingress sg-6e616f6d69_ingress_tcp_8000_8000_10.0.3.0/24
* ```
* Import a rule with various IPv4 and IPv6 source CIDR blocks:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule ingress sg-4973616163_ingress_tcp_100_121_10.1.0.0/16_2001:db8::/48_10.2.0.0/16_2002:db8::/48
* ```
* Import a rule, applicable to all ports, with a protocol other than TCP/UDP/ICMP/ICMPV6/ALL, e.g., Multicast Transport Protocol (MTP), using the IANA protocol number. For example: 92.
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule ingress sg-6777656e646f6c796e_ingress_92_0_65536_10.0.3.0/24_10.0.4.0/24
* ```
* Import a default any/any egress rule to 0.0.0.0/0:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule default_egress sg-6777656e646f6c796e_egress_all_0_0_0.0.0.0/0
* ```
* Import an egress rule with a prefix list ID destination:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule egress sg-62726f6479_egress_tcp_8000_8000_pl-6469726b
* ```
* Import a rule applicable to all protocols and ports with a security group source:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule ingress_rule sg-7472697374616e_ingress_all_0_65536_sg-6176657279
* ```
* Import a rule that has itself and an IPv6 CIDR block as sources:
*
* ```sh
* $ pulumi import aws:ec2/securityGroupRule:SecurityGroupRule rule_name sg-656c65616e6f72_ingress_tcp_80_80_self_2001:db8::/48
* ```
*
*/
@ResourceType(type="aws:ec2/securityGroupRule:SecurityGroupRule")
public class SecurityGroupRule extends com.pulumi.resources.CustomResource {
/**
* List of CIDR blocks. Cannot be specified with `source_security_group_id` or `self`.
*
*/
@Export(name="cidrBlocks", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> cidrBlocks;
/**
* @return List of CIDR blocks. Cannot be specified with `source_security_group_id` or `self`.
*
*/
public Output>> cidrBlocks() {
return Codegen.optional(this.cidrBlocks);
}
/**
* Description of the rule.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the rule.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Start port (or ICMP type number if protocol is "icmp" or "icmpv6").
*
*/
@Export(name="fromPort", refs={Integer.class}, tree="[0]")
private Output fromPort;
/**
* @return Start port (or ICMP type number if protocol is "icmp" or "icmpv6").
*
*/
public Output fromPort() {
return this.fromPort;
}
/**
* List of IPv6 CIDR blocks. Cannot be specified with `source_security_group_id` or `self`.
*
*/
@Export(name="ipv6CidrBlocks", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> ipv6CidrBlocks;
/**
* @return List of IPv6 CIDR blocks. Cannot be specified with `source_security_group_id` or `self`.
*
*/
public Output>> ipv6CidrBlocks() {
return Codegen.optional(this.ipv6CidrBlocks);
}
/**
* List of Prefix List IDs.
*
*/
@Export(name="prefixListIds", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> prefixListIds;
/**
* @return List of Prefix List IDs.
*
*/
public Output>> prefixListIds() {
return Codegen.optional(this.prefixListIds);
}
/**
* Protocol. If not icmp, icmpv6, tcp, udp, or all use the [protocol number](https://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml)
*
*/
@Export(name="protocol", refs={String.class}, tree="[0]")
private Output protocol;
/**
* @return Protocol. If not icmp, icmpv6, tcp, udp, or all use the [protocol number](https://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml)
*
*/
public Output protocol() {
return this.protocol;
}
/**
* Security group to apply this rule to.
*
*/
@Export(name="securityGroupId", refs={String.class}, tree="[0]")
private Output securityGroupId;
/**
* @return Security group to apply this rule to.
*
*/
public Output securityGroupId() {
return this.securityGroupId;
}
/**
* If the `aws.ec2.SecurityGroupRule` resource has a single source or destination then this is the AWS Security Group Rule resource ID. Otherwise it is empty.
*
*/
@Export(name="securityGroupRuleId", refs={String.class}, tree="[0]")
private Output securityGroupRuleId;
/**
* @return If the `aws.ec2.SecurityGroupRule` resource has a single source or destination then this is the AWS Security Group Rule resource ID. Otherwise it is empty.
*
*/
public Output securityGroupRuleId() {
return this.securityGroupRuleId;
}
/**
* Whether the security group itself will be added as a source to this ingress rule. Cannot be specified with `cidr_blocks`, `ipv6_cidr_blocks`, or `source_security_group_id`.
*
*/
@Export(name="self", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> self;
/**
* @return Whether the security group itself will be added as a source to this ingress rule. Cannot be specified with `cidr_blocks`, `ipv6_cidr_blocks`, or `source_security_group_id`.
*
*/
public Output> self() {
return Codegen.optional(this.self);
}
/**
* Security group id to allow access to/from, depending on the `type`. Cannot be specified with `cidr_blocks`, `ipv6_cidr_blocks`, or `self`.
*
*/
@Export(name="sourceSecurityGroupId", refs={String.class}, tree="[0]")
private Output sourceSecurityGroupId;
/**
* @return Security group id to allow access to/from, depending on the `type`. Cannot be specified with `cidr_blocks`, `ipv6_cidr_blocks`, or `self`.
*
*/
public Output sourceSecurityGroupId() {
return this.sourceSecurityGroupId;
}
/**
* End port (or ICMP code if protocol is "icmp").
*
*/
@Export(name="toPort", refs={Integer.class}, tree="[0]")
private Output toPort;
/**
* @return End port (or ICMP code if protocol is "icmp").
*
*/
public Output toPort() {
return this.toPort;
}
/**
* Type of rule being created. Valid options are `ingress` (inbound)
* or `egress` (outbound).
*
* The following arguments are optional:
*
* > **Note** Although `cidr_blocks`, `ipv6_cidr_blocks`, `prefix_list_ids`, and `source_security_group_id` are all marked as optional, you _must_ provide one of them in order to configure the source of the traffic.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Type of rule being created. Valid options are `ingress` (inbound)
* or `egress` (outbound).
*
* The following arguments are optional:
*
* > **Note** Although `cidr_blocks`, `ipv6_cidr_blocks`, `prefix_list_ids`, and `source_security_group_id` are all marked as optional, you _must_ provide one of them in order to configure the source of the traffic.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SecurityGroupRule(java.lang.String name) {
this(name, SecurityGroupRuleArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SecurityGroupRule(java.lang.String name, SecurityGroupRuleArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SecurityGroupRule(java.lang.String name, SecurityGroupRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2/securityGroupRule:SecurityGroupRule", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SecurityGroupRule(java.lang.String name, Output id, @Nullable SecurityGroupRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2/securityGroupRule:SecurityGroupRule", name, state, makeResourceOptions(options, id), false);
}
private static SecurityGroupRuleArgs makeArgs(SecurityGroupRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SecurityGroupRuleArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SecurityGroupRule get(java.lang.String name, Output id, @Nullable SecurityGroupRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SecurityGroupRule(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy