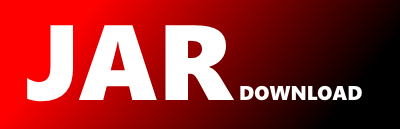
com.pulumi.aws.ec2.VpcIpam Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.VpcIpamArgs;
import com.pulumi.aws.ec2.inputs.VpcIpamState;
import com.pulumi.aws.ec2.outputs.VpcIpamOperatingRegion;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an IPAM resource.
*
* ## Example Usage
*
* Basic usage:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.ec2.VpcIpam;
* import com.pulumi.aws.ec2.VpcIpamArgs;
* import com.pulumi.aws.ec2.inputs.VpcIpamOperatingRegionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getRegion();
*
* var main = new VpcIpam("main", VpcIpamArgs.builder()
* .description("My IPAM")
* .operatingRegions(VpcIpamOperatingRegionArgs.builder()
* .regionName(current.applyValue(getRegionResult -> getRegionResult.name()))
* .build())
* .tags(Map.of("Test", "Main"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Shared with multiple operating_regions:
*
* ## Import
*
* Using `pulumi import`, import IPAMs using the IPAM `id`. For example:
*
* ```sh
* $ pulumi import aws:ec2/vpcIpam:VpcIpam example ipam-0178368ad2146a492
* ```
*
*/
@ResourceType(type="aws:ec2/vpcIpam:VpcIpam")
public class VpcIpam extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name (ARN) of IPAM
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) of IPAM
*
*/
public Output arn() {
return this.arn;
}
/**
* Enables you to quickly delete an IPAM, private scopes, pools in private scopes, and any allocations in the pools in private scopes.
*
*/
@Export(name="cascade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> cascade;
/**
* @return Enables you to quickly delete an IPAM, private scopes, pools in private scopes, and any allocations in the pools in private scopes.
*
*/
public Output> cascade() {
return Codegen.optional(this.cascade);
}
/**
* The IPAM's default resource discovery association ID.
*
*/
@Export(name="defaultResourceDiscoveryAssociationId", refs={String.class}, tree="[0]")
private Output defaultResourceDiscoveryAssociationId;
/**
* @return The IPAM's default resource discovery association ID.
*
*/
public Output defaultResourceDiscoveryAssociationId() {
return this.defaultResourceDiscoveryAssociationId;
}
/**
* The IPAM's default resource discovery ID.
*
*/
@Export(name="defaultResourceDiscoveryId", refs={String.class}, tree="[0]")
private Output defaultResourceDiscoveryId;
/**
* @return The IPAM's default resource discovery ID.
*
*/
public Output defaultResourceDiscoveryId() {
return this.defaultResourceDiscoveryId;
}
/**
* A description for the IPAM.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return A description for the IPAM.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Determines which locales can be chosen when you create pools. Locale is the Region where you want to make an IPAM pool available for allocations. You can only create pools with locales that match the operating Regions of the IPAM. You can only create VPCs from a pool whose locale matches the VPC's Region. You specify a region using the region_name parameter. You **must** set your provider block region as an operating_region.
*
*/
@Export(name="operatingRegions", refs={List.class,VpcIpamOperatingRegion.class}, tree="[0,1]")
private Output> operatingRegions;
/**
* @return Determines which locales can be chosen when you create pools. Locale is the Region where you want to make an IPAM pool available for allocations. You can only create pools with locales that match the operating Regions of the IPAM. You can only create VPCs from a pool whose locale matches the VPC's Region. You specify a region using the region_name parameter. You **must** set your provider block region as an operating_region.
*
*/
public Output> operatingRegions() {
return this.operatingRegions;
}
/**
* The ID of the IPAM's private scope. A scope is a top-level container in IPAM. Each scope represents an IP-independent network. Scopes enable you to represent networks where you have overlapping IP space. When you create an IPAM, IPAM automatically creates two scopes: public and private. The private scope is intended for private IP space. The public scope is intended for all internet-routable IP space.
*
*/
@Export(name="privateDefaultScopeId", refs={String.class}, tree="[0]")
private Output privateDefaultScopeId;
/**
* @return The ID of the IPAM's private scope. A scope is a top-level container in IPAM. Each scope represents an IP-independent network. Scopes enable you to represent networks where you have overlapping IP space. When you create an IPAM, IPAM automatically creates two scopes: public and private. The private scope is intended for private IP space. The public scope is intended for all internet-routable IP space.
*
*/
public Output privateDefaultScopeId() {
return this.privateDefaultScopeId;
}
/**
* The ID of the IPAM's public scope. A scope is a top-level container in IPAM. Each scope represents an IP-independent network. Scopes enable you to represent networks where you have overlapping IP space. When you create an IPAM, IPAM automatically creates two scopes: public and private. The private scope is intended for private
* IP space. The public scope is intended for all internet-routable IP space.
*
*/
@Export(name="publicDefaultScopeId", refs={String.class}, tree="[0]")
private Output publicDefaultScopeId;
/**
* @return The ID of the IPAM's public scope. A scope is a top-level container in IPAM. Each scope represents an IP-independent network. Scopes enable you to represent networks where you have overlapping IP space. When you create an IPAM, IPAM automatically creates two scopes: public and private. The private scope is intended for private
* IP space. The public scope is intended for all internet-routable IP space.
*
*/
public Output publicDefaultScopeId() {
return this.publicDefaultScopeId;
}
/**
* The number of scopes in the IPAM.
*
*/
@Export(name="scopeCount", refs={Integer.class}, tree="[0]")
private Output scopeCount;
/**
* @return The number of scopes in the IPAM.
*
*/
public Output scopeCount() {
return this.scopeCount;
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy