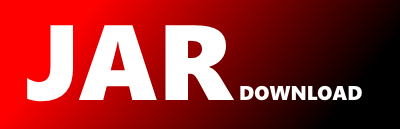
com.pulumi.aws.ec2.inputs.GetInstanceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.GetInstanceFilterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetInstanceArgs extends com.pulumi.resources.InvokeArgs {
public static final GetInstanceArgs Empty = new GetInstanceArgs();
/**
* One or more name/value pairs to use as filters. There are
* several valid keys, for a full reference, check out
* [describe-instances in the AWS CLI reference][1].
*
*/
@Import(name="filters")
private @Nullable Output> filters;
/**
* @return One or more name/value pairs to use as filters. There are
* several valid keys, for a full reference, check out
* [describe-instances in the AWS CLI reference][1].
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy