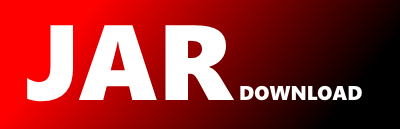
com.pulumi.aws.ec2.inputs.InternetGatewayState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InternetGatewayState extends com.pulumi.resources.ResourceArgs {
public static final InternetGatewayState Empty = new InternetGatewayState();
/**
* The ARN of the Internet Gateway.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return The ARN of the Internet Gateway.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy