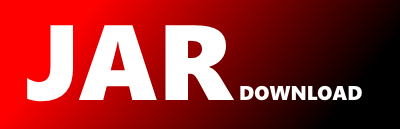
com.pulumi.aws.ec2.inputs.SpotFleetRequestLaunchSpecificationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.SpotFleetRequestLaunchSpecificationEbsBlockDeviceArgs;
import com.pulumi.aws.ec2.inputs.SpotFleetRequestLaunchSpecificationEphemeralBlockDeviceArgs;
import com.pulumi.aws.ec2.inputs.SpotFleetRequestLaunchSpecificationRootBlockDeviceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SpotFleetRequestLaunchSpecificationArgs extends com.pulumi.resources.ResourceArgs {
public static final SpotFleetRequestLaunchSpecificationArgs Empty = new SpotFleetRequestLaunchSpecificationArgs();
@Import(name="ami", required=true)
private Output ami;
public Output ami() {
return this.ami;
}
@Import(name="associatePublicIpAddress")
private @Nullable Output associatePublicIpAddress;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy