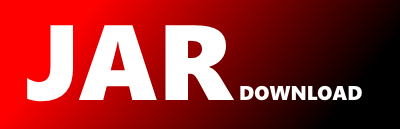
com.pulumi.aws.ec2.outputs.AmiEbsBlockDevice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AmiEbsBlockDevice {
/**
* @return Boolean controlling whether the EBS volumes created to
* support each created instance will be deleted once that instance is terminated.
*
*/
private @Nullable Boolean deleteOnTermination;
/**
* @return Path at which the device is exposed to created instances.
*
*/
private String deviceName;
/**
* @return Boolean controlling whether the created EBS volumes will be encrypted. Can't be used with `snapshot_id`.
*
*/
private @Nullable Boolean encrypted;
/**
* @return Number of I/O operations per second the
* created volumes will support.
*
*/
private @Nullable Integer iops;
/**
* @return ARN of the Outpost on which the snapshot is stored.
*
* > **Note:** You can specify `encrypted` or `snapshot_id` but not both.
*
*/
private @Nullable String outpostArn;
/**
* @return ID of an EBS snapshot that will be used to initialize the created
* EBS volumes. If set, the `volume_size` attribute must be at least as large as the referenced
* snapshot.
*
*/
private @Nullable String snapshotId;
/**
* @return Throughput that the EBS volume supports, in MiB/s. Only valid for `volume_type` of `gp3`.
*
*/
private @Nullable Integer throughput;
/**
* @return Size of created volumes in GiB.
* If `snapshot_id` is set and `volume_size` is omitted then the volume will have the same size
* as the selected snapshot.
*
*/
private @Nullable Integer volumeSize;
/**
* @return Type of EBS volume to create. Can be `standard`, `gp2`, `gp3`, `io1`, `io2`, `sc1` or `st1` (Default: `standard`).
*
*/
private @Nullable String volumeType;
private AmiEbsBlockDevice() {}
/**
* @return Boolean controlling whether the EBS volumes created to
* support each created instance will be deleted once that instance is terminated.
*
*/
public Optional deleteOnTermination() {
return Optional.ofNullable(this.deleteOnTermination);
}
/**
* @return Path at which the device is exposed to created instances.
*
*/
public String deviceName() {
return this.deviceName;
}
/**
* @return Boolean controlling whether the created EBS volumes will be encrypted. Can't be used with `snapshot_id`.
*
*/
public Optional encrypted() {
return Optional.ofNullable(this.encrypted);
}
/**
* @return Number of I/O operations per second the
* created volumes will support.
*
*/
public Optional iops() {
return Optional.ofNullable(this.iops);
}
/**
* @return ARN of the Outpost on which the snapshot is stored.
*
* > **Note:** You can specify `encrypted` or `snapshot_id` but not both.
*
*/
public Optional outpostArn() {
return Optional.ofNullable(this.outpostArn);
}
/**
* @return ID of an EBS snapshot that will be used to initialize the created
* EBS volumes. If set, the `volume_size` attribute must be at least as large as the referenced
* snapshot.
*
*/
public Optional snapshotId() {
return Optional.ofNullable(this.snapshotId);
}
/**
* @return Throughput that the EBS volume supports, in MiB/s. Only valid for `volume_type` of `gp3`.
*
*/
public Optional throughput() {
return Optional.ofNullable(this.throughput);
}
/**
* @return Size of created volumes in GiB.
* If `snapshot_id` is set and `volume_size` is omitted then the volume will have the same size
* as the selected snapshot.
*
*/
public Optional volumeSize() {
return Optional.ofNullable(this.volumeSize);
}
/**
* @return Type of EBS volume to create. Can be `standard`, `gp2`, `gp3`, `io1`, `io2`, `sc1` or `st1` (Default: `standard`).
*
*/
public Optional volumeType() {
return Optional.ofNullable(this.volumeType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AmiEbsBlockDevice defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean deleteOnTermination;
private String deviceName;
private @Nullable Boolean encrypted;
private @Nullable Integer iops;
private @Nullable String outpostArn;
private @Nullable String snapshotId;
private @Nullable Integer throughput;
private @Nullable Integer volumeSize;
private @Nullable String volumeType;
public Builder() {}
public Builder(AmiEbsBlockDevice defaults) {
Objects.requireNonNull(defaults);
this.deleteOnTermination = defaults.deleteOnTermination;
this.deviceName = defaults.deviceName;
this.encrypted = defaults.encrypted;
this.iops = defaults.iops;
this.outpostArn = defaults.outpostArn;
this.snapshotId = defaults.snapshotId;
this.throughput = defaults.throughput;
this.volumeSize = defaults.volumeSize;
this.volumeType = defaults.volumeType;
}
@CustomType.Setter
public Builder deleteOnTermination(@Nullable Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder deviceName(String deviceName) {
if (deviceName == null) {
throw new MissingRequiredPropertyException("AmiEbsBlockDevice", "deviceName");
}
this.deviceName = deviceName;
return this;
}
@CustomType.Setter
public Builder encrypted(@Nullable Boolean encrypted) {
this.encrypted = encrypted;
return this;
}
@CustomType.Setter
public Builder iops(@Nullable Integer iops) {
this.iops = iops;
return this;
}
@CustomType.Setter
public Builder outpostArn(@Nullable String outpostArn) {
this.outpostArn = outpostArn;
return this;
}
@CustomType.Setter
public Builder snapshotId(@Nullable String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
@CustomType.Setter
public Builder throughput(@Nullable Integer throughput) {
this.throughput = throughput;
return this;
}
@CustomType.Setter
public Builder volumeSize(@Nullable Integer volumeSize) {
this.volumeSize = volumeSize;
return this;
}
@CustomType.Setter
public Builder volumeType(@Nullable String volumeType) {
this.volumeType = volumeType;
return this;
}
public AmiEbsBlockDevice build() {
final var _resultValue = new AmiEbsBlockDevice();
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.deviceName = deviceName;
_resultValue.encrypted = encrypted;
_resultValue.iops = iops;
_resultValue.outpostArn = outpostArn;
_resultValue.snapshotId = snapshotId;
_resultValue.throughput = throughput;
_resultValue.volumeSize = volumeSize;
_resultValue.volumeType = volumeType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy