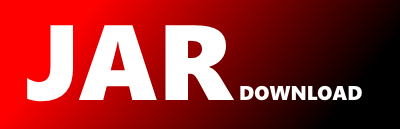
com.pulumi.aws.ec2.outputs.GetLaunchTemplateNetworkInterface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLaunchTemplateNetworkInterface {
private String associateCarrierIpAddress;
private @Nullable Boolean associatePublicIpAddress;
private @Nullable Boolean deleteOnTermination;
private String description;
private Integer deviceIndex;
private String interfaceType;
private Integer ipv4AddressCount;
private List ipv4Addresses;
private Integer ipv4PrefixCount;
private List ipv4Prefixes;
private Integer ipv6AddressCount;
private List ipv6Addresses;
private Integer ipv6PrefixCount;
private List ipv6Prefixes;
private Integer networkCardIndex;
private String networkInterfaceId;
private String primaryIpv6;
private String privateIpAddress;
private List securityGroups;
private String subnetId;
private GetLaunchTemplateNetworkInterface() {}
public String associateCarrierIpAddress() {
return this.associateCarrierIpAddress;
}
public Optional associatePublicIpAddress() {
return Optional.ofNullable(this.associatePublicIpAddress);
}
public Optional deleteOnTermination() {
return Optional.ofNullable(this.deleteOnTermination);
}
public String description() {
return this.description;
}
public Integer deviceIndex() {
return this.deviceIndex;
}
public String interfaceType() {
return this.interfaceType;
}
public Integer ipv4AddressCount() {
return this.ipv4AddressCount;
}
public List ipv4Addresses() {
return this.ipv4Addresses;
}
public Integer ipv4PrefixCount() {
return this.ipv4PrefixCount;
}
public List ipv4Prefixes() {
return this.ipv4Prefixes;
}
public Integer ipv6AddressCount() {
return this.ipv6AddressCount;
}
public List ipv6Addresses() {
return this.ipv6Addresses;
}
public Integer ipv6PrefixCount() {
return this.ipv6PrefixCount;
}
public List ipv6Prefixes() {
return this.ipv6Prefixes;
}
public Integer networkCardIndex() {
return this.networkCardIndex;
}
public String networkInterfaceId() {
return this.networkInterfaceId;
}
public String primaryIpv6() {
return this.primaryIpv6;
}
public String privateIpAddress() {
return this.privateIpAddress;
}
public List securityGroups() {
return this.securityGroups;
}
public String subnetId() {
return this.subnetId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLaunchTemplateNetworkInterface defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String associateCarrierIpAddress;
private @Nullable Boolean associatePublicIpAddress;
private @Nullable Boolean deleteOnTermination;
private String description;
private Integer deviceIndex;
private String interfaceType;
private Integer ipv4AddressCount;
private List ipv4Addresses;
private Integer ipv4PrefixCount;
private List ipv4Prefixes;
private Integer ipv6AddressCount;
private List ipv6Addresses;
private Integer ipv6PrefixCount;
private List ipv6Prefixes;
private Integer networkCardIndex;
private String networkInterfaceId;
private String primaryIpv6;
private String privateIpAddress;
private List securityGroups;
private String subnetId;
public Builder() {}
public Builder(GetLaunchTemplateNetworkInterface defaults) {
Objects.requireNonNull(defaults);
this.associateCarrierIpAddress = defaults.associateCarrierIpAddress;
this.associatePublicIpAddress = defaults.associatePublicIpAddress;
this.deleteOnTermination = defaults.deleteOnTermination;
this.description = defaults.description;
this.deviceIndex = defaults.deviceIndex;
this.interfaceType = defaults.interfaceType;
this.ipv4AddressCount = defaults.ipv4AddressCount;
this.ipv4Addresses = defaults.ipv4Addresses;
this.ipv4PrefixCount = defaults.ipv4PrefixCount;
this.ipv4Prefixes = defaults.ipv4Prefixes;
this.ipv6AddressCount = defaults.ipv6AddressCount;
this.ipv6Addresses = defaults.ipv6Addresses;
this.ipv6PrefixCount = defaults.ipv6PrefixCount;
this.ipv6Prefixes = defaults.ipv6Prefixes;
this.networkCardIndex = defaults.networkCardIndex;
this.networkInterfaceId = defaults.networkInterfaceId;
this.primaryIpv6 = defaults.primaryIpv6;
this.privateIpAddress = defaults.privateIpAddress;
this.securityGroups = defaults.securityGroups;
this.subnetId = defaults.subnetId;
}
@CustomType.Setter
public Builder associateCarrierIpAddress(String associateCarrierIpAddress) {
if (associateCarrierIpAddress == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "associateCarrierIpAddress");
}
this.associateCarrierIpAddress = associateCarrierIpAddress;
return this;
}
@CustomType.Setter
public Builder associatePublicIpAddress(@Nullable Boolean associatePublicIpAddress) {
this.associatePublicIpAddress = associatePublicIpAddress;
return this;
}
@CustomType.Setter
public Builder deleteOnTermination(@Nullable Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder deviceIndex(Integer deviceIndex) {
if (deviceIndex == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "deviceIndex");
}
this.deviceIndex = deviceIndex;
return this;
}
@CustomType.Setter
public Builder interfaceType(String interfaceType) {
if (interfaceType == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "interfaceType");
}
this.interfaceType = interfaceType;
return this;
}
@CustomType.Setter
public Builder ipv4AddressCount(Integer ipv4AddressCount) {
if (ipv4AddressCount == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv4AddressCount");
}
this.ipv4AddressCount = ipv4AddressCount;
return this;
}
@CustomType.Setter
public Builder ipv4Addresses(List ipv4Addresses) {
if (ipv4Addresses == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv4Addresses");
}
this.ipv4Addresses = ipv4Addresses;
return this;
}
public Builder ipv4Addresses(String... ipv4Addresses) {
return ipv4Addresses(List.of(ipv4Addresses));
}
@CustomType.Setter
public Builder ipv4PrefixCount(Integer ipv4PrefixCount) {
if (ipv4PrefixCount == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv4PrefixCount");
}
this.ipv4PrefixCount = ipv4PrefixCount;
return this;
}
@CustomType.Setter
public Builder ipv4Prefixes(List ipv4Prefixes) {
if (ipv4Prefixes == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv4Prefixes");
}
this.ipv4Prefixes = ipv4Prefixes;
return this;
}
public Builder ipv4Prefixes(String... ipv4Prefixes) {
return ipv4Prefixes(List.of(ipv4Prefixes));
}
@CustomType.Setter
public Builder ipv6AddressCount(Integer ipv6AddressCount) {
if (ipv6AddressCount == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv6AddressCount");
}
this.ipv6AddressCount = ipv6AddressCount;
return this;
}
@CustomType.Setter
public Builder ipv6Addresses(List ipv6Addresses) {
if (ipv6Addresses == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv6Addresses");
}
this.ipv6Addresses = ipv6Addresses;
return this;
}
public Builder ipv6Addresses(String... ipv6Addresses) {
return ipv6Addresses(List.of(ipv6Addresses));
}
@CustomType.Setter
public Builder ipv6PrefixCount(Integer ipv6PrefixCount) {
if (ipv6PrefixCount == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv6PrefixCount");
}
this.ipv6PrefixCount = ipv6PrefixCount;
return this;
}
@CustomType.Setter
public Builder ipv6Prefixes(List ipv6Prefixes) {
if (ipv6Prefixes == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "ipv6Prefixes");
}
this.ipv6Prefixes = ipv6Prefixes;
return this;
}
public Builder ipv6Prefixes(String... ipv6Prefixes) {
return ipv6Prefixes(List.of(ipv6Prefixes));
}
@CustomType.Setter
public Builder networkCardIndex(Integer networkCardIndex) {
if (networkCardIndex == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "networkCardIndex");
}
this.networkCardIndex = networkCardIndex;
return this;
}
@CustomType.Setter
public Builder networkInterfaceId(String networkInterfaceId) {
if (networkInterfaceId == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "networkInterfaceId");
}
this.networkInterfaceId = networkInterfaceId;
return this;
}
@CustomType.Setter
public Builder primaryIpv6(String primaryIpv6) {
if (primaryIpv6 == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "primaryIpv6");
}
this.primaryIpv6 = primaryIpv6;
return this;
}
@CustomType.Setter
public Builder privateIpAddress(String privateIpAddress) {
if (privateIpAddress == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "privateIpAddress");
}
this.privateIpAddress = privateIpAddress;
return this;
}
@CustomType.Setter
public Builder securityGroups(List securityGroups) {
if (securityGroups == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "securityGroups");
}
this.securityGroups = securityGroups;
return this;
}
public Builder securityGroups(String... securityGroups) {
return securityGroups(List.of(securityGroups));
}
@CustomType.Setter
public Builder subnetId(String subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetLaunchTemplateNetworkInterface", "subnetId");
}
this.subnetId = subnetId;
return this;
}
public GetLaunchTemplateNetworkInterface build() {
final var _resultValue = new GetLaunchTemplateNetworkInterface();
_resultValue.associateCarrierIpAddress = associateCarrierIpAddress;
_resultValue.associatePublicIpAddress = associatePublicIpAddress;
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.description = description;
_resultValue.deviceIndex = deviceIndex;
_resultValue.interfaceType = interfaceType;
_resultValue.ipv4AddressCount = ipv4AddressCount;
_resultValue.ipv4Addresses = ipv4Addresses;
_resultValue.ipv4PrefixCount = ipv4PrefixCount;
_resultValue.ipv4Prefixes = ipv4Prefixes;
_resultValue.ipv6AddressCount = ipv6AddressCount;
_resultValue.ipv6Addresses = ipv6Addresses;
_resultValue.ipv6PrefixCount = ipv6PrefixCount;
_resultValue.ipv6Prefixes = ipv6Prefixes;
_resultValue.networkCardIndex = networkCardIndex;
_resultValue.networkInterfaceId = networkInterfaceId;
_resultValue.primaryIpv6 = primaryIpv6;
_resultValue.privateIpAddress = privateIpAddress;
_resultValue.securityGroups = securityGroups;
_resultValue.subnetId = subnetId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy