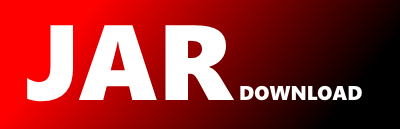
com.pulumi.aws.ec2.outputs.GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute {
private String destinationCidr;
private String destinationPrefixListId;
private String egressOnlyInternetGatewayId;
private String gatewayId;
private String instanceId;
private String natGatewayId;
private String networkInterfaceId;
private String origin;
private String transitGatewayId;
private String vpcPeeringConnectionId;
private GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute() {}
public String destinationCidr() {
return this.destinationCidr;
}
public String destinationPrefixListId() {
return this.destinationPrefixListId;
}
public String egressOnlyInternetGatewayId() {
return this.egressOnlyInternetGatewayId;
}
public String gatewayId() {
return this.gatewayId;
}
public String instanceId() {
return this.instanceId;
}
public String natGatewayId() {
return this.natGatewayId;
}
public String networkInterfaceId() {
return this.networkInterfaceId;
}
public String origin() {
return this.origin;
}
public String transitGatewayId() {
return this.transitGatewayId;
}
public String vpcPeeringConnectionId() {
return this.vpcPeeringConnectionId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String destinationCidr;
private String destinationPrefixListId;
private String egressOnlyInternetGatewayId;
private String gatewayId;
private String instanceId;
private String natGatewayId;
private String networkInterfaceId;
private String origin;
private String transitGatewayId;
private String vpcPeeringConnectionId;
public Builder() {}
public Builder(GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute defaults) {
Objects.requireNonNull(defaults);
this.destinationCidr = defaults.destinationCidr;
this.destinationPrefixListId = defaults.destinationPrefixListId;
this.egressOnlyInternetGatewayId = defaults.egressOnlyInternetGatewayId;
this.gatewayId = defaults.gatewayId;
this.instanceId = defaults.instanceId;
this.natGatewayId = defaults.natGatewayId;
this.networkInterfaceId = defaults.networkInterfaceId;
this.origin = defaults.origin;
this.transitGatewayId = defaults.transitGatewayId;
this.vpcPeeringConnectionId = defaults.vpcPeeringConnectionId;
}
@CustomType.Setter
public Builder destinationCidr(String destinationCidr) {
if (destinationCidr == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "destinationCidr");
}
this.destinationCidr = destinationCidr;
return this;
}
@CustomType.Setter
public Builder destinationPrefixListId(String destinationPrefixListId) {
if (destinationPrefixListId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "destinationPrefixListId");
}
this.destinationPrefixListId = destinationPrefixListId;
return this;
}
@CustomType.Setter
public Builder egressOnlyInternetGatewayId(String egressOnlyInternetGatewayId) {
if (egressOnlyInternetGatewayId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "egressOnlyInternetGatewayId");
}
this.egressOnlyInternetGatewayId = egressOnlyInternetGatewayId;
return this;
}
@CustomType.Setter
public Builder gatewayId(String gatewayId) {
if (gatewayId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "gatewayId");
}
this.gatewayId = gatewayId;
return this;
}
@CustomType.Setter
public Builder instanceId(String instanceId) {
if (instanceId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "instanceId");
}
this.instanceId = instanceId;
return this;
}
@CustomType.Setter
public Builder natGatewayId(String natGatewayId) {
if (natGatewayId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "natGatewayId");
}
this.natGatewayId = natGatewayId;
return this;
}
@CustomType.Setter
public Builder networkInterfaceId(String networkInterfaceId) {
if (networkInterfaceId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "networkInterfaceId");
}
this.networkInterfaceId = networkInterfaceId;
return this;
}
@CustomType.Setter
public Builder origin(String origin) {
if (origin == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "origin");
}
this.origin = origin;
return this;
}
@CustomType.Setter
public Builder transitGatewayId(String transitGatewayId) {
if (transitGatewayId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "transitGatewayId");
}
this.transitGatewayId = transitGatewayId;
return this;
}
@CustomType.Setter
public Builder vpcPeeringConnectionId(String vpcPeeringConnectionId) {
if (vpcPeeringConnectionId == null) {
throw new MissingRequiredPropertyException("GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute", "vpcPeeringConnectionId");
}
this.vpcPeeringConnectionId = vpcPeeringConnectionId;
return this;
}
public GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute build() {
final var _resultValue = new GetNetworkInsightsAnalysisForwardPathComponentRouteTableRoute();
_resultValue.destinationCidr = destinationCidr;
_resultValue.destinationPrefixListId = destinationPrefixListId;
_resultValue.egressOnlyInternetGatewayId = egressOnlyInternetGatewayId;
_resultValue.gatewayId = gatewayId;
_resultValue.instanceId = instanceId;
_resultValue.natGatewayId = natGatewayId;
_resultValue.networkInterfaceId = networkInterfaceId;
_resultValue.origin = origin;
_resultValue.transitGatewayId = transitGatewayId;
_resultValue.vpcPeeringConnectionId = vpcPeeringConnectionId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy