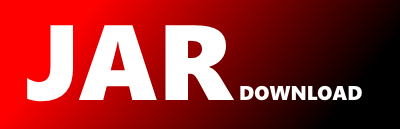
com.pulumi.aws.ecr.Repository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecr;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ecr.RepositoryArgs;
import com.pulumi.aws.ecr.inputs.RepositoryState;
import com.pulumi.aws.ecr.outputs.RepositoryEncryptionConfiguration;
import com.pulumi.aws.ecr.outputs.RepositoryImageScanningConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Elastic Container Registry Repository.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ecr.Repository;
* import com.pulumi.aws.ecr.RepositoryArgs;
* import com.pulumi.aws.ecr.inputs.RepositoryImageScanningConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foo = new Repository("foo", RepositoryArgs.builder()
* .name("bar")
* .imageTagMutability("MUTABLE")
* .imageScanningConfiguration(RepositoryImageScanningConfigurationArgs.builder()
* .scanOnPush(true)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import ECR Repositories using the `name`. For example:
*
* ```sh
* $ pulumi import aws:ecr/repository:Repository service test-service
* ```
*
*/
@ResourceType(type="aws:ecr/repository:Repository")
public class Repository extends com.pulumi.resources.CustomResource {
/**
* Full ARN of the repository.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Full ARN of the repository.
*
*/
public Output arn() {
return this.arn;
}
/**
* Encryption configuration for the repository. See below for schema.
*
*/
@Export(name="encryptionConfigurations", refs={List.class,RepositoryEncryptionConfiguration.class}, tree="[0,1]")
private Output* @Nullable */ List> encryptionConfigurations;
/**
* @return Encryption configuration for the repository. See below for schema.
*
*/
public Output>> encryptionConfigurations() {
return Codegen.optional(this.encryptionConfigurations);
}
/**
* If `true`, will delete the repository even if it contains images.
* Defaults to `false`.
*
*/
@Export(name="forceDelete", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceDelete;
/**
* @return If `true`, will delete the repository even if it contains images.
* Defaults to `false`.
*
*/
public Output> forceDelete() {
return Codegen.optional(this.forceDelete);
}
/**
* Configuration block that defines image scanning configuration for the repository. By default, image scanning must be manually triggered. See the [ECR User Guide](https://docs.aws.amazon.com/AmazonECR/latest/userguide/image-scanning.html) for more information about image scanning.
*
*/
@Export(name="imageScanningConfiguration", refs={RepositoryImageScanningConfiguration.class}, tree="[0]")
private Output* @Nullable */ RepositoryImageScanningConfiguration> imageScanningConfiguration;
/**
* @return Configuration block that defines image scanning configuration for the repository. By default, image scanning must be manually triggered. See the [ECR User Guide](https://docs.aws.amazon.com/AmazonECR/latest/userguide/image-scanning.html) for more information about image scanning.
*
*/
public Output> imageScanningConfiguration() {
return Codegen.optional(this.imageScanningConfiguration);
}
/**
* The tag mutability setting for the repository. Must be one of: `MUTABLE` or `IMMUTABLE`. Defaults to `MUTABLE`.
*
*/
@Export(name="imageTagMutability", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> imageTagMutability;
/**
* @return The tag mutability setting for the repository. Must be one of: `MUTABLE` or `IMMUTABLE`. Defaults to `MUTABLE`.
*
*/
public Output> imageTagMutability() {
return Codegen.optional(this.imageTagMutability);
}
/**
* Name of the repository.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the repository.
*
*/
public Output name() {
return this.name;
}
/**
* The registry ID where the repository was created.
*
*/
@Export(name="registryId", refs={String.class}, tree="[0]")
private Output registryId;
/**
* @return The registry ID where the repository was created.
*
*/
public Output registryId() {
return this.registryId;
}
/**
* The URL of the repository (in the form `aws_account_id.dkr.ecr.region.amazonaws.com/repositoryName`).
*
*/
@Export(name="repositoryUrl", refs={String.class}, tree="[0]")
private Output repositoryUrl;
/**
* @return The URL of the repository (in the form `aws_account_id.dkr.ecr.region.amazonaws.com/repositoryName`).
*
*/
public Output repositoryUrl() {
return this.repositoryUrl;
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy