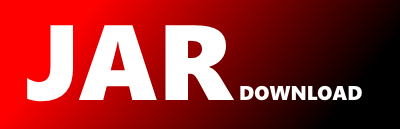
com.pulumi.aws.efs.FileSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.efs;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.efs.FileSystemArgs;
import com.pulumi.aws.efs.inputs.FileSystemState;
import com.pulumi.aws.efs.outputs.FileSystemLifecyclePolicy;
import com.pulumi.aws.efs.outputs.FileSystemProtection;
import com.pulumi.aws.efs.outputs.FileSystemSizeInByte;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Elastic File System (EFS) File System resource.
*
* ## Example Usage
*
* ### EFS File System w/ tags
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.efs.FileSystem;
* import com.pulumi.aws.efs.FileSystemArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foo = new FileSystem("foo", FileSystemArgs.builder()
* .creationToken("my-product")
* .tags(Map.of("Name", "MyProduct"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Using lifecycle policy
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.efs.FileSystem;
* import com.pulumi.aws.efs.FileSystemArgs;
* import com.pulumi.aws.efs.inputs.FileSystemLifecyclePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fooWithLifecylePolicy = new FileSystem("fooWithLifecylePolicy", FileSystemArgs.builder()
* .creationToken("my-product")
* .lifecyclePolicies(FileSystemLifecyclePolicyArgs.builder()
* .transitionToIa("AFTER_30_DAYS")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import the EFS file systems using the `id`. For example:
*
* ```sh
* $ pulumi import aws:efs/fileSystem:FileSystem foo fs-6fa144c6
* ```
*
*/
@ResourceType(type="aws:efs/fileSystem:FileSystem")
public class FileSystem extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name of the file system.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name of the file system.
*
*/
public Output arn() {
return this.arn;
}
/**
* The identifier of the Availability Zone in which the file system's One Zone storage classes exist.
*
*/
@Export(name="availabilityZoneId", refs={String.class}, tree="[0]")
private Output availabilityZoneId;
/**
* @return The identifier of the Availability Zone in which the file system's One Zone storage classes exist.
*
*/
public Output availabilityZoneId() {
return this.availabilityZoneId;
}
/**
* the AWS Availability Zone in which to create the file system. Used to create a file system that uses One Zone storage classes. See [user guide](https://docs.aws.amazon.com/efs/latest/ug/availability-durability.html) for more information.
*
*/
@Export(name="availabilityZoneName", refs={String.class}, tree="[0]")
private Output availabilityZoneName;
/**
* @return the AWS Availability Zone in which to create the file system. Used to create a file system that uses One Zone storage classes. See [user guide](https://docs.aws.amazon.com/efs/latest/ug/availability-durability.html) for more information.
*
*/
public Output availabilityZoneName() {
return this.availabilityZoneName;
}
/**
* A unique name (a maximum of 64 characters are allowed)
* used as reference when creating the Elastic File System to ensure idempotent file
* system creation. By default generated by this provider. See [Elastic File System]
* user guide for more information.
*
*/
@Export(name="creationToken", refs={String.class}, tree="[0]")
private Output creationToken;
/**
* @return A unique name (a maximum of 64 characters are allowed)
* used as reference when creating the Elastic File System to ensure idempotent file
* system creation. By default generated by this provider. See [Elastic File System]
* user guide for more information.
*
*/
public Output creationToken() {
return this.creationToken;
}
/**
* The DNS name for the filesystem per [documented convention](http://docs.aws.amazon.com/efs/latest/ug/mounting-fs-mount-cmd-dns-name.html).
*
*/
@Export(name="dnsName", refs={String.class}, tree="[0]")
private Output dnsName;
/**
* @return The DNS name for the filesystem per [documented convention](http://docs.aws.amazon.com/efs/latest/ug/mounting-fs-mount-cmd-dns-name.html).
*
*/
public Output dnsName() {
return this.dnsName;
}
/**
* If true, the disk will be encrypted.
*
*/
@Export(name="encrypted", refs={Boolean.class}, tree="[0]")
private Output encrypted;
/**
* @return If true, the disk will be encrypted.
*
*/
public Output encrypted() {
return this.encrypted;
}
/**
* The ARN for the KMS encryption key. When specifying kms_key_id, encrypted needs to be set to true.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return The ARN for the KMS encryption key. When specifying kms_key_id, encrypted needs to be set to true.
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
/**
* A file system [lifecycle policy](https://docs.aws.amazon.com/efs/latest/ug/API_LifecyclePolicy.html) object. See `lifecycle_policy` block below for details.
*
*/
@Export(name="lifecyclePolicies", refs={List.class,FileSystemLifecyclePolicy.class}, tree="[0,1]")
private Output* @Nullable */ List> lifecyclePolicies;
/**
* @return A file system [lifecycle policy](https://docs.aws.amazon.com/efs/latest/ug/API_LifecyclePolicy.html) object. See `lifecycle_policy` block below for details.
*
*/
public Output>> lifecyclePolicies() {
return Codegen.optional(this.lifecyclePolicies);
}
/**
* The value of the file system's `Name` tag.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The value of the file system's `Name` tag.
*
*/
public Output name() {
return this.name;
}
/**
* The current number of mount targets that the file system has.
*
*/
@Export(name="numberOfMountTargets", refs={Integer.class}, tree="[0]")
private Output numberOfMountTargets;
/**
* @return The current number of mount targets that the file system has.
*
*/
public Output numberOfMountTargets() {
return this.numberOfMountTargets;
}
/**
* The AWS account that created the file system. If the file system was createdby an IAM user, the parent account to which the user belongs is the owner.
*
*/
@Export(name="ownerId", refs={String.class}, tree="[0]")
private Output ownerId;
/**
* @return The AWS account that created the file system. If the file system was createdby an IAM user, the parent account to which the user belongs is the owner.
*
*/
public Output ownerId() {
return this.ownerId;
}
/**
* The file system performance mode. Can be either `"generalPurpose"` or `"maxIO"` (Default: `"generalPurpose"`).
*
*/
@Export(name="performanceMode", refs={String.class}, tree="[0]")
private Output performanceMode;
/**
* @return The file system performance mode. Can be either `"generalPurpose"` or `"maxIO"` (Default: `"generalPurpose"`).
*
*/
public Output performanceMode() {
return this.performanceMode;
}
/**
* A file system [protection](https://docs.aws.amazon.com/efs/latest/ug/API_FileSystemProtectionDescription.html) object. See `protection` block below for details.
*
*/
@Export(name="protection", refs={FileSystemProtection.class}, tree="[0]")
private Output protection;
/**
* @return A file system [protection](https://docs.aws.amazon.com/efs/latest/ug/API_FileSystemProtectionDescription.html) object. See `protection` block below for details.
*
*/
public Output protection() {
return this.protection;
}
/**
* The throughput, measured in MiB/s, that you want to provision for the file system. Only applicable with `throughput_mode` set to `provisioned`.
*
*/
@Export(name="provisionedThroughputInMibps", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> provisionedThroughputInMibps;
/**
* @return The throughput, measured in MiB/s, that you want to provision for the file system. Only applicable with `throughput_mode` set to `provisioned`.
*
*/
public Output> provisionedThroughputInMibps() {
return Codegen.optional(this.provisionedThroughputInMibps);
}
/**
* The latest known metered size (in bytes) of data stored in the file system, the value is not the exact size that the file system was at any point in time. See Size In Bytes.
*
*/
@Export(name="sizeInBytes", refs={List.class,FileSystemSizeInByte.class}, tree="[0,1]")
private Output> sizeInBytes;
/**
* @return The latest known metered size (in bytes) of data stored in the file system, the value is not the exact size that the file system was at any point in time. See Size In Bytes.
*
*/
public Output> sizeInBytes() {
return this.sizeInBytes;
}
/**
* A map of tags to assign to the file system. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the file system. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy