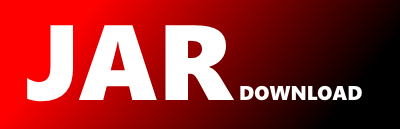
com.pulumi.aws.eks.Cluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.eks.ClusterArgs;
import com.pulumi.aws.eks.inputs.ClusterState;
import com.pulumi.aws.eks.outputs.ClusterAccessConfig;
import com.pulumi.aws.eks.outputs.ClusterCertificateAuthority;
import com.pulumi.aws.eks.outputs.ClusterEncryptionConfig;
import com.pulumi.aws.eks.outputs.ClusterIdentity;
import com.pulumi.aws.eks.outputs.ClusterKubernetesNetworkConfig;
import com.pulumi.aws.eks.outputs.ClusterOutpostConfig;
import com.pulumi.aws.eks.outputs.ClusterUpgradePolicy;
import com.pulumi.aws.eks.outputs.ClusterVpcConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an EKS Cluster.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.eks.Cluster;
* import com.pulumi.aws.eks.ClusterArgs;
* import com.pulumi.aws.eks.inputs.ClusterVpcConfigArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Cluster("example", ClusterArgs.builder()
* .name("example")
* .roleArn(exampleAwsIamRole.arn())
* .vpcConfig(ClusterVpcConfigArgs.builder()
* .subnetIds(
* example1.id(),
* example2.id())
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* example_AmazonEKSClusterPolicy,
* example_AmazonEKSVPCResourceController)
* .build());
*
* ctx.export("endpoint", example.endpoint());
* ctx.export("kubeconfig-certificate-authority-data", example.certificateAuthority().applyValue(certificateAuthority -> certificateAuthority.data()));
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Example IAM Role for EKS Cluster
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("eks.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var example = new Role("example", RoleArgs.builder()
* .name("eks-cluster-example")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var example_AmazonEKSClusterPolicy = new RolePolicyAttachment("example-AmazonEKSClusterPolicy", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/AmazonEKSClusterPolicy")
* .role(example.name())
* .build());
*
* // Optionally, enable Security Groups for Pods
* // Reference: https://docs.aws.amazon.com/eks/latest/userguide/security-groups-for-pods.html
* var example_AmazonEKSVPCResourceController = new RolePolicyAttachment("example-AmazonEKSVPCResourceController", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/AmazonEKSVPCResourceController")
* .role(example.name())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Enabling Control Plane Logging
*
* [EKS Control Plane Logging](https://docs.aws.amazon.com/eks/latest/userguide/control-plane-logs.html) can be enabled via the `enabled_cluster_log_types` argument. To manage the CloudWatch Log Group retention period, the `aws.cloudwatch.LogGroup` resource can be used.
*
* > The below configuration uses [`dependsOn`](https://www.pulumi.com/docs/intro/concepts/programming-model/#dependson) to prevent ordering issues with EKS automatically creating the log group first and a variable for naming consistency. Other ordering and naming methodologies may be more appropriate for your environment.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogGroup;
* import com.pulumi.aws.cloudwatch.LogGroupArgs;
* import com.pulumi.aws.eks.Cluster;
* import com.pulumi.aws.eks.ClusterArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var clusterName = config.get("clusterName").orElse("example");
* var exampleLogGroup = new LogGroup("exampleLogGroup", LogGroupArgs.builder()
* .name(String.format("/aws/eks/%s/cluster", clusterName))
* .retentionInDays(7)
* .build());
*
* var example = new Cluster("example", ClusterArgs.builder()
* .enabledClusterLogTypes(
* "api",
* "audit")
* .name(clusterName)
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleLogGroup)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Enabling IAM Roles for Service Accounts
*
* For more information about this feature, see the [EKS User Guide](https://docs.aws.amazon.com/eks/latest/userguide/enable-iam-roles-for-service-accounts.html).
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.eks.Cluster;
* import com.pulumi.tls.TlsFunctions;
* import com.pulumi.tls.inputs.GetCertificateArgs;
* import com.pulumi.aws.iam.OpenIdConnectProvider;
* import com.pulumi.aws.iam.OpenIdConnectProviderArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleCluster = new Cluster("exampleCluster");
*
* final var example = TlsFunctions.getCertificate(GetCertificateArgs.builder()
* .url(exampleCluster.identities().applyValue(identities -> identities[0].oidcs()[0].issuer()))
* .build());
*
* var exampleOpenIdConnectProvider = new OpenIdConnectProvider("exampleOpenIdConnectProvider", OpenIdConnectProviderArgs.builder()
* .clientIdLists("sts.amazonaws.com")
* .thumbprintLists(example.applyValue(getCertificateResult -> getCertificateResult).applyValue(example -> example.applyValue(getCertificateResult -> getCertificateResult.certificates()[0].sha1Fingerprint())))
* .url(example.applyValue(getCertificateResult -> getCertificateResult).applyValue(example -> example.applyValue(getCertificateResult -> getCertificateResult.url())))
* .build());
*
* final var exampleAssumeRolePolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("sts:AssumeRoleWithWebIdentity")
* .effect("Allow")
* .conditions(GetPolicyDocumentStatementConditionArgs.builder()
* .test("StringEquals")
* .variable(StdFunctions.replace().applyValue(invoke -> String.format("%s:sub", invoke.result())))
* .values("system:serviceaccount:kube-system:aws-node")
* .build())
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .identifiers(exampleOpenIdConnectProvider.arn())
* .type("Federated")
* .build())
* .build())
* .build());
*
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .assumeRolePolicy(exampleAssumeRolePolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(exampleAssumeRolePolicy -> exampleAssumeRolePolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .name("example")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### EKS Cluster on AWS Outpost
*
* [Creating a local Amazon EKS cluster on an AWS Outpost](https://docs.aws.amazon.com/eks/latest/userguide/create-cluster-outpost.html)
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.eks.Cluster;
* import com.pulumi.aws.eks.ClusterArgs;
* import com.pulumi.aws.eks.inputs.ClusterVpcConfigArgs;
* import com.pulumi.aws.eks.inputs.ClusterOutpostConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Role("example", RoleArgs.builder()
* .assumeRolePolicy(exampleAssumeRolePolicy.json())
* .name("example")
* .build());
*
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .name("example-cluster")
* .roleArn(example.arn())
* .vpcConfig(ClusterVpcConfigArgs.builder()
* .endpointPrivateAccess(true)
* .endpointPublicAccess(false)
* .build())
* .outpostConfig(ClusterOutpostConfigArgs.builder()
* .controlPlaneInstanceType("m5d.large")
* .outpostArns(exampleAwsOutpostsOutpost.arn())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### EKS Cluster with Access Config
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.eks.Cluster;
* import com.pulumi.aws.eks.ClusterArgs;
* import com.pulumi.aws.eks.inputs.ClusterVpcConfigArgs;
* import com.pulumi.aws.eks.inputs.ClusterAccessConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Role("example", RoleArgs.builder()
* .assumeRolePolicy(exampleAssumeRolePolicy.json())
* .name("example")
* .build());
*
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .name("example-cluster")
* .roleArn(example.arn())
* .vpcConfig(ClusterVpcConfigArgs.builder()
* .endpointPrivateAccess(true)
* .endpointPublicAccess(false)
* .build())
* .accessConfig(ClusterAccessConfigArgs.builder()
* .authenticationMode("CONFIG_MAP")
* .bootstrapClusterCreatorAdminPermissions(true)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* After adding inline IAM Policies (e.g., `aws.iam.RolePolicy` resource) or attaching IAM Policies (e.g., `aws.iam.Policy` resource and `aws.iam.RolePolicyAttachment` resource) with the desired permissions to the IAM Role, annotate the Kubernetes service account (e.g., `kubernetes_service_account` resource) and recreate any pods.
*
* ## Import
*
* Using `pulumi import`, import EKS Clusters using the `name`. For example:
*
* ```sh
* $ pulumi import aws:eks/cluster:Cluster my_cluster my_cluster
* ```
*
*/
@ResourceType(type="aws:eks/cluster:Cluster")
public class Cluster extends com.pulumi.resources.CustomResource {
/**
* Configuration block for the access config associated with your cluster, see [Amazon EKS Access Entries](https://docs.aws.amazon.com/eks/latest/userguide/access-entries.html).
*
*/
@Export(name="accessConfig", refs={ClusterAccessConfig.class}, tree="[0]")
private Output accessConfig;
/**
* @return Configuration block for the access config associated with your cluster, see [Amazon EKS Access Entries](https://docs.aws.amazon.com/eks/latest/userguide/access-entries.html).
*
*/
public Output accessConfig() {
return this.accessConfig;
}
/**
* ARN of the cluster.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the cluster.
*
*/
public Output arn() {
return this.arn;
}
/**
* Install default unmanaged add-ons, such as `aws-cni`, `kube-proxy`, and CoreDNS during cluster creation. If `false`, you must manually install desired add-ons. Changing this value will force a new cluster to be created. Defaults to `true`.
*
*/
@Export(name="bootstrapSelfManagedAddons", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> bootstrapSelfManagedAddons;
/**
* @return Install default unmanaged add-ons, such as `aws-cni`, `kube-proxy`, and CoreDNS during cluster creation. If `false`, you must manually install desired add-ons. Changing this value will force a new cluster to be created. Defaults to `true`.
*
*/
public Output> bootstrapSelfManagedAddons() {
return Codegen.optional(this.bootstrapSelfManagedAddons);
}
@Export(name="certificateAuthorities", refs={List.class,ClusterCertificateAuthority.class}, tree="[0,1]")
private Output> certificateAuthorities;
public Output> certificateAuthorities() {
return this.certificateAuthorities;
}
/**
* Attribute block containing `certificate-authority-data` for your cluster. Detailed below.
*
*/
@Export(name="certificateAuthority", refs={ClusterCertificateAuthority.class}, tree="[0]")
private Output certificateAuthority;
/**
* @return Attribute block containing `certificate-authority-data` for your cluster. Detailed below.
*
*/
public Output certificateAuthority() {
return this.certificateAuthority;
}
/**
* The ID of your local Amazon EKS cluster on the AWS Outpost. This attribute isn't available for an AWS EKS cluster on AWS cloud.
*
*/
@Export(name="clusterId", refs={String.class}, tree="[0]")
private Output clusterId;
/**
* @return The ID of your local Amazon EKS cluster on the AWS Outpost. This attribute isn't available for an AWS EKS cluster on AWS cloud.
*
*/
public Output clusterId() {
return this.clusterId;
}
/**
* Unix epoch timestamp in seconds for when the cluster was created.
*
*/
@Export(name="createdAt", refs={String.class}, tree="[0]")
private Output createdAt;
/**
* @return Unix epoch timestamp in seconds for when the cluster was created.
*
*/
public Output createdAt() {
return this.createdAt;
}
@Export(name="defaultAddonsToRemoves", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> defaultAddonsToRemoves;
public Output>> defaultAddonsToRemoves() {
return Codegen.optional(this.defaultAddonsToRemoves);
}
/**
* List of the desired control plane logging to enable. For more information, see [Amazon EKS Control Plane Logging](https://docs.aws.amazon.com/eks/latest/userguide/control-plane-logs.html).
*
*/
@Export(name="enabledClusterLogTypes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> enabledClusterLogTypes;
/**
* @return List of the desired control plane logging to enable. For more information, see [Amazon EKS Control Plane Logging](https://docs.aws.amazon.com/eks/latest/userguide/control-plane-logs.html).
*
*/
public Output>> enabledClusterLogTypes() {
return Codegen.optional(this.enabledClusterLogTypes);
}
/**
* Configuration block with encryption configuration for the cluster. Only available on Kubernetes 1.13 and above clusters created after March 6, 2020. Detailed below.
*
*/
@Export(name="encryptionConfig", refs={ClusterEncryptionConfig.class}, tree="[0]")
private Output* @Nullable */ ClusterEncryptionConfig> encryptionConfig;
/**
* @return Configuration block with encryption configuration for the cluster. Only available on Kubernetes 1.13 and above clusters created after March 6, 2020. Detailed below.
*
*/
public Output> encryptionConfig() {
return Codegen.optional(this.encryptionConfig);
}
/**
* Endpoint for your Kubernetes API server.
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return Endpoint for your Kubernetes API server.
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* Attribute block containing identity provider information for your cluster. Only available on Kubernetes version 1.13 and 1.14 clusters created or upgraded on or after September 3, 2019. Detailed below.
*
*/
@Export(name="identities", refs={List.class,ClusterIdentity.class}, tree="[0,1]")
private Output> identities;
/**
* @return Attribute block containing identity provider information for your cluster. Only available on Kubernetes version 1.13 and 1.14 clusters created or upgraded on or after September 3, 2019. Detailed below.
*
*/
public Output> identities() {
return this.identities;
}
/**
* Configuration block with kubernetes network configuration for the cluster. Detailed below. If removed, this provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="kubernetesNetworkConfig", refs={ClusterKubernetesNetworkConfig.class}, tree="[0]")
private Output kubernetesNetworkConfig;
/**
* @return Configuration block with kubernetes network configuration for the cluster. Detailed below. If removed, this provider will only perform drift detection if a configuration value is provided.
*
*/
public Output kubernetesNetworkConfig() {
return this.kubernetesNetworkConfig;
}
/**
* Name of the cluster. Must be between 1-100 characters in length. Must begin with an alphanumeric character, and must only contain alphanumeric characters, dashes and underscores (`^[0-9A-Za-z][A-Za-z0-9\-_]*$`).
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the cluster. Must be between 1-100 characters in length. Must begin with an alphanumeric character, and must only contain alphanumeric characters, dashes and underscores (`^[0-9A-Za-z][A-Za-z0-9\-_]*$`).
*
*/
public Output name() {
return this.name;
}
/**
* Configuration block representing the configuration of your local Amazon EKS cluster on an AWS Outpost. This block isn't available for creating Amazon EKS clusters on the AWS cloud.
*
*/
@Export(name="outpostConfig", refs={ClusterOutpostConfig.class}, tree="[0]")
private Output* @Nullable */ ClusterOutpostConfig> outpostConfig;
/**
* @return Configuration block representing the configuration of your local Amazon EKS cluster on an AWS Outpost. This block isn't available for creating Amazon EKS clusters on the AWS cloud.
*
*/
public Output> outpostConfig() {
return Codegen.optional(this.outpostConfig);
}
/**
* Platform version for the cluster.
*
*/
@Export(name="platformVersion", refs={String.class}, tree="[0]")
private Output platformVersion;
/**
* @return Platform version for the cluster.
*
*/
public Output platformVersion() {
return this.platformVersion;
}
/**
* ARN of the IAM role that provides permissions for the Kubernetes control plane to make calls to AWS API operations on your behalf. Ensure the resource configuration includes explicit dependencies on the IAM Role permissions by adding `depends_on` if using the `aws.iam.RolePolicy` resource or `aws.iam.RolePolicyAttachment` resource, otherwise EKS cannot delete EKS managed EC2 infrastructure such as Security Groups on EKS Cluster deletion.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output roleArn;
/**
* @return ARN of the IAM role that provides permissions for the Kubernetes control plane to make calls to AWS API operations on your behalf. Ensure the resource configuration includes explicit dependencies on the IAM Role permissions by adding `depends_on` if using the `aws.iam.RolePolicy` resource or `aws.iam.RolePolicyAttachment` resource, otherwise EKS cannot delete EKS managed EC2 infrastructure such as Security Groups on EKS Cluster deletion.
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* Status of the EKS cluster. One of `CREATING`, `ACTIVE`, `DELETING`, `FAILED`.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Status of the EKS cluster. One of `CREATING`, `ACTIVE`, `DELETING`, `FAILED`.
*
*/
public Output status() {
return this.status;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy