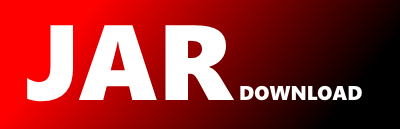
com.pulumi.aws.eks.outputs.ClusterOutpostConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks.outputs;
import com.pulumi.aws.eks.outputs.ClusterOutpostConfigControlPlanePlacement;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterOutpostConfig {
/**
* @return The Amazon EC2 instance type that you want to use for your local Amazon EKS cluster on Outposts. The instance type that you specify is used for all Kubernetes control plane instances. The instance type can't be changed after cluster creation. Choose an instance type based on the number of nodes that your cluster will have. If your cluster will have:
*
* * 1–20 nodes, then we recommend specifying a large instance type.
*
* * 21–100 nodes, then we recommend specifying an xlarge instance type.
*
* * 101–250 nodes, then we recommend specifying a 2xlarge instance type.
*
* For a list of the available Amazon EC2 instance types, see Compute and storage in AWS Outposts rack features The control plane is not automatically scaled by Amazon EKS.
*
*/
private String controlPlaneInstanceType;
/**
* @return An object representing the placement configuration for all the control plane instances of your local Amazon EKS cluster on AWS Outpost.
* The `control_plane_placement` configuration block supports the following arguments:
*
*/
private @Nullable ClusterOutpostConfigControlPlanePlacement controlPlanePlacement;
/**
* @return The ARN of the Outpost that you want to use for your local Amazon EKS cluster on Outposts. This argument is a list of arns, but only a single Outpost ARN is supported currently.
*
*/
private List outpostArns;
private ClusterOutpostConfig() {}
/**
* @return The Amazon EC2 instance type that you want to use for your local Amazon EKS cluster on Outposts. The instance type that you specify is used for all Kubernetes control plane instances. The instance type can't be changed after cluster creation. Choose an instance type based on the number of nodes that your cluster will have. If your cluster will have:
*
* * 1–20 nodes, then we recommend specifying a large instance type.
*
* * 21–100 nodes, then we recommend specifying an xlarge instance type.
*
* * 101–250 nodes, then we recommend specifying a 2xlarge instance type.
*
* For a list of the available Amazon EC2 instance types, see Compute and storage in AWS Outposts rack features The control plane is not automatically scaled by Amazon EKS.
*
*/
public String controlPlaneInstanceType() {
return this.controlPlaneInstanceType;
}
/**
* @return An object representing the placement configuration for all the control plane instances of your local Amazon EKS cluster on AWS Outpost.
* The `control_plane_placement` configuration block supports the following arguments:
*
*/
public Optional controlPlanePlacement() {
return Optional.ofNullable(this.controlPlanePlacement);
}
/**
* @return The ARN of the Outpost that you want to use for your local Amazon EKS cluster on Outposts. This argument is a list of arns, but only a single Outpost ARN is supported currently.
*
*/
public List outpostArns() {
return this.outpostArns;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterOutpostConfig defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String controlPlaneInstanceType;
private @Nullable ClusterOutpostConfigControlPlanePlacement controlPlanePlacement;
private List outpostArns;
public Builder() {}
public Builder(ClusterOutpostConfig defaults) {
Objects.requireNonNull(defaults);
this.controlPlaneInstanceType = defaults.controlPlaneInstanceType;
this.controlPlanePlacement = defaults.controlPlanePlacement;
this.outpostArns = defaults.outpostArns;
}
@CustomType.Setter
public Builder controlPlaneInstanceType(String controlPlaneInstanceType) {
if (controlPlaneInstanceType == null) {
throw new MissingRequiredPropertyException("ClusterOutpostConfig", "controlPlaneInstanceType");
}
this.controlPlaneInstanceType = controlPlaneInstanceType;
return this;
}
@CustomType.Setter
public Builder controlPlanePlacement(@Nullable ClusterOutpostConfigControlPlanePlacement controlPlanePlacement) {
this.controlPlanePlacement = controlPlanePlacement;
return this;
}
@CustomType.Setter
public Builder outpostArns(List outpostArns) {
if (outpostArns == null) {
throw new MissingRequiredPropertyException("ClusterOutpostConfig", "outpostArns");
}
this.outpostArns = outpostArns;
return this;
}
public Builder outpostArns(String... outpostArns) {
return outpostArns(List.of(outpostArns));
}
public ClusterOutpostConfig build() {
final var _resultValue = new ClusterOutpostConfig();
_resultValue.controlPlaneInstanceType = controlPlaneInstanceType;
_resultValue.controlPlanePlacement = controlPlanePlacement;
_resultValue.outpostArns = outpostArns;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy