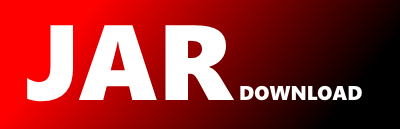
com.pulumi.aws.emr.outputs.ClusterKerberosAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emr.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterKerberosAttributes {
/**
* @return Active Directory password for `ad_domain_join_user`. This provider cannot perform drift detection of this configuration.
*
*/
private @Nullable String adDomainJoinPassword;
/**
* @return Required only when establishing a cross-realm trust with an Active Directory domain. A user with sufficient privileges to join resources to the domain. This provider cannot perform drift detection of this configuration.
*
*/
private @Nullable String adDomainJoinUser;
/**
* @return Required only when establishing a cross-realm trust with a KDC in a different realm. The cross-realm principal password, which must be identical across realms. This provider cannot perform drift detection of this configuration.
*
*/
private @Nullable String crossRealmTrustPrincipalPassword;
/**
* @return Password used within the cluster for the kadmin service on the cluster-dedicated KDC, which maintains Kerberos principals, password policies, and keytabs for the cluster. This provider cannot perform drift detection of this configuration.
*
*/
private String kdcAdminPassword;
/**
* @return Name of the Kerberos realm to which all nodes in a cluster belong. For example, `EC2.INTERNAL`
*
*/
private String realm;
private ClusterKerberosAttributes() {}
/**
* @return Active Directory password for `ad_domain_join_user`. This provider cannot perform drift detection of this configuration.
*
*/
public Optional adDomainJoinPassword() {
return Optional.ofNullable(this.adDomainJoinPassword);
}
/**
* @return Required only when establishing a cross-realm trust with an Active Directory domain. A user with sufficient privileges to join resources to the domain. This provider cannot perform drift detection of this configuration.
*
*/
public Optional adDomainJoinUser() {
return Optional.ofNullable(this.adDomainJoinUser);
}
/**
* @return Required only when establishing a cross-realm trust with a KDC in a different realm. The cross-realm principal password, which must be identical across realms. This provider cannot perform drift detection of this configuration.
*
*/
public Optional crossRealmTrustPrincipalPassword() {
return Optional.ofNullable(this.crossRealmTrustPrincipalPassword);
}
/**
* @return Password used within the cluster for the kadmin service on the cluster-dedicated KDC, which maintains Kerberos principals, password policies, and keytabs for the cluster. This provider cannot perform drift detection of this configuration.
*
*/
public String kdcAdminPassword() {
return this.kdcAdminPassword;
}
/**
* @return Name of the Kerberos realm to which all nodes in a cluster belong. For example, `EC2.INTERNAL`
*
*/
public String realm() {
return this.realm;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterKerberosAttributes defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String adDomainJoinPassword;
private @Nullable String adDomainJoinUser;
private @Nullable String crossRealmTrustPrincipalPassword;
private String kdcAdminPassword;
private String realm;
public Builder() {}
public Builder(ClusterKerberosAttributes defaults) {
Objects.requireNonNull(defaults);
this.adDomainJoinPassword = defaults.adDomainJoinPassword;
this.adDomainJoinUser = defaults.adDomainJoinUser;
this.crossRealmTrustPrincipalPassword = defaults.crossRealmTrustPrincipalPassword;
this.kdcAdminPassword = defaults.kdcAdminPassword;
this.realm = defaults.realm;
}
@CustomType.Setter
public Builder adDomainJoinPassword(@Nullable String adDomainJoinPassword) {
this.adDomainJoinPassword = adDomainJoinPassword;
return this;
}
@CustomType.Setter
public Builder adDomainJoinUser(@Nullable String adDomainJoinUser) {
this.adDomainJoinUser = adDomainJoinUser;
return this;
}
@CustomType.Setter
public Builder crossRealmTrustPrincipalPassword(@Nullable String crossRealmTrustPrincipalPassword) {
this.crossRealmTrustPrincipalPassword = crossRealmTrustPrincipalPassword;
return this;
}
@CustomType.Setter
public Builder kdcAdminPassword(String kdcAdminPassword) {
if (kdcAdminPassword == null) {
throw new MissingRequiredPropertyException("ClusterKerberosAttributes", "kdcAdminPassword");
}
this.kdcAdminPassword = kdcAdminPassword;
return this;
}
@CustomType.Setter
public Builder realm(String realm) {
if (realm == null) {
throw new MissingRequiredPropertyException("ClusterKerberosAttributes", "realm");
}
this.realm = realm;
return this;
}
public ClusterKerberosAttributes build() {
final var _resultValue = new ClusterKerberosAttributes();
_resultValue.adDomainJoinPassword = adDomainJoinPassword;
_resultValue.adDomainJoinUser = adDomainJoinUser;
_resultValue.crossRealmTrustPrincipalPassword = crossRealmTrustPrincipalPassword;
_resultValue.kdcAdminPassword = kdcAdminPassword;
_resultValue.realm = realm;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy