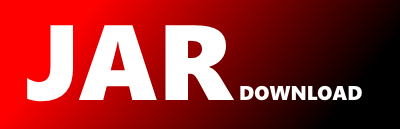
com.pulumi.aws.emr.outputs.ClusterMasterInstanceFleet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emr.outputs;
import com.pulumi.aws.emr.outputs.ClusterMasterInstanceFleetInstanceTypeConfig;
import com.pulumi.aws.emr.outputs.ClusterMasterInstanceFleetLaunchSpecifications;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterMasterInstanceFleet {
/**
* @return ID of the cluster.
*
*/
private @Nullable String id;
/**
* @return Configuration block for instance fleet.
*
*/
private @Nullable List instanceTypeConfigs;
/**
* @return Configuration block for launch specification.
*
*/
private @Nullable ClusterMasterInstanceFleetLaunchSpecifications launchSpecifications;
/**
* @return Friendly name given to the instance fleet.
*
*/
private @Nullable String name;
private @Nullable Integer provisionedOnDemandCapacity;
private @Nullable Integer provisionedSpotCapacity;
/**
* @return Target capacity of On-Demand units for the instance fleet, which determines how many On-Demand instances to provision.
*
*/
private @Nullable Integer targetOnDemandCapacity;
/**
* @return Target capacity of Spot units for the instance fleet, which determines how many Spot instances to provision.
*
*/
private @Nullable Integer targetSpotCapacity;
private ClusterMasterInstanceFleet() {}
/**
* @return ID of the cluster.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Configuration block for instance fleet.
*
*/
public List instanceTypeConfigs() {
return this.instanceTypeConfigs == null ? List.of() : this.instanceTypeConfigs;
}
/**
* @return Configuration block for launch specification.
*
*/
public Optional launchSpecifications() {
return Optional.ofNullable(this.launchSpecifications);
}
/**
* @return Friendly name given to the instance fleet.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
public Optional provisionedOnDemandCapacity() {
return Optional.ofNullable(this.provisionedOnDemandCapacity);
}
public Optional provisionedSpotCapacity() {
return Optional.ofNullable(this.provisionedSpotCapacity);
}
/**
* @return Target capacity of On-Demand units for the instance fleet, which determines how many On-Demand instances to provision.
*
*/
public Optional targetOnDemandCapacity() {
return Optional.ofNullable(this.targetOnDemandCapacity);
}
/**
* @return Target capacity of Spot units for the instance fleet, which determines how many Spot instances to provision.
*
*/
public Optional targetSpotCapacity() {
return Optional.ofNullable(this.targetSpotCapacity);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterMasterInstanceFleet defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String id;
private @Nullable List instanceTypeConfigs;
private @Nullable ClusterMasterInstanceFleetLaunchSpecifications launchSpecifications;
private @Nullable String name;
private @Nullable Integer provisionedOnDemandCapacity;
private @Nullable Integer provisionedSpotCapacity;
private @Nullable Integer targetOnDemandCapacity;
private @Nullable Integer targetSpotCapacity;
public Builder() {}
public Builder(ClusterMasterInstanceFleet defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.instanceTypeConfigs = defaults.instanceTypeConfigs;
this.launchSpecifications = defaults.launchSpecifications;
this.name = defaults.name;
this.provisionedOnDemandCapacity = defaults.provisionedOnDemandCapacity;
this.provisionedSpotCapacity = defaults.provisionedSpotCapacity;
this.targetOnDemandCapacity = defaults.targetOnDemandCapacity;
this.targetSpotCapacity = defaults.targetSpotCapacity;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceTypeConfigs(@Nullable List instanceTypeConfigs) {
this.instanceTypeConfigs = instanceTypeConfigs;
return this;
}
public Builder instanceTypeConfigs(ClusterMasterInstanceFleetInstanceTypeConfig... instanceTypeConfigs) {
return instanceTypeConfigs(List.of(instanceTypeConfigs));
}
@CustomType.Setter
public Builder launchSpecifications(@Nullable ClusterMasterInstanceFleetLaunchSpecifications launchSpecifications) {
this.launchSpecifications = launchSpecifications;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisionedOnDemandCapacity(@Nullable Integer provisionedOnDemandCapacity) {
this.provisionedOnDemandCapacity = provisionedOnDemandCapacity;
return this;
}
@CustomType.Setter
public Builder provisionedSpotCapacity(@Nullable Integer provisionedSpotCapacity) {
this.provisionedSpotCapacity = provisionedSpotCapacity;
return this;
}
@CustomType.Setter
public Builder targetOnDemandCapacity(@Nullable Integer targetOnDemandCapacity) {
this.targetOnDemandCapacity = targetOnDemandCapacity;
return this;
}
@CustomType.Setter
public Builder targetSpotCapacity(@Nullable Integer targetSpotCapacity) {
this.targetSpotCapacity = targetSpotCapacity;
return this;
}
public ClusterMasterInstanceFleet build() {
final var _resultValue = new ClusterMasterInstanceFleet();
_resultValue.id = id;
_resultValue.instanceTypeConfigs = instanceTypeConfigs;
_resultValue.launchSpecifications = launchSpecifications;
_resultValue.name = name;
_resultValue.provisionedOnDemandCapacity = provisionedOnDemandCapacity;
_resultValue.provisionedSpotCapacity = provisionedSpotCapacity;
_resultValue.targetOnDemandCapacity = targetOnDemandCapacity;
_resultValue.targetSpotCapacity = targetSpotCapacity;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy