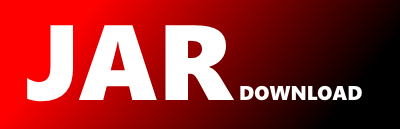
com.pulumi.aws.emrcontainers.outputs.JobTemplateJobTemplateData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emrcontainers.outputs;
import com.pulumi.aws.emrcontainers.outputs.JobTemplateJobTemplateDataConfigurationOverrides;
import com.pulumi.aws.emrcontainers.outputs.JobTemplateJobTemplateDataJobDriver;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class JobTemplateJobTemplateData {
/**
* @return The configuration settings that are used to override defaults configuration.
*
*/
private @Nullable JobTemplateJobTemplateDataConfigurationOverrides configurationOverrides;
/**
* @return The execution role ARN of the job run.
*
*/
private String executionRoleArn;
/**
* @return Specify the driver that the job runs on. Exactly one of the two available job drivers is required, either sparkSqlJobDriver or sparkSubmitJobDriver.
*
*/
private JobTemplateJobTemplateDataJobDriver jobDriver;
/**
* @return The tags assigned to jobs started using the job template.
*
*/
private @Nullable Map jobTags;
/**
* @return The release version of Amazon EMR.
*
*/
private String releaseLabel;
private JobTemplateJobTemplateData() {}
/**
* @return The configuration settings that are used to override defaults configuration.
*
*/
public Optional configurationOverrides() {
return Optional.ofNullable(this.configurationOverrides);
}
/**
* @return The execution role ARN of the job run.
*
*/
public String executionRoleArn() {
return this.executionRoleArn;
}
/**
* @return Specify the driver that the job runs on. Exactly one of the two available job drivers is required, either sparkSqlJobDriver or sparkSubmitJobDriver.
*
*/
public JobTemplateJobTemplateDataJobDriver jobDriver() {
return this.jobDriver;
}
/**
* @return The tags assigned to jobs started using the job template.
*
*/
public Map jobTags() {
return this.jobTags == null ? Map.of() : this.jobTags;
}
/**
* @return The release version of Amazon EMR.
*
*/
public String releaseLabel() {
return this.releaseLabel;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(JobTemplateJobTemplateData defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable JobTemplateJobTemplateDataConfigurationOverrides configurationOverrides;
private String executionRoleArn;
private JobTemplateJobTemplateDataJobDriver jobDriver;
private @Nullable Map jobTags;
private String releaseLabel;
public Builder() {}
public Builder(JobTemplateJobTemplateData defaults) {
Objects.requireNonNull(defaults);
this.configurationOverrides = defaults.configurationOverrides;
this.executionRoleArn = defaults.executionRoleArn;
this.jobDriver = defaults.jobDriver;
this.jobTags = defaults.jobTags;
this.releaseLabel = defaults.releaseLabel;
}
@CustomType.Setter
public Builder configurationOverrides(@Nullable JobTemplateJobTemplateDataConfigurationOverrides configurationOverrides) {
this.configurationOverrides = configurationOverrides;
return this;
}
@CustomType.Setter
public Builder executionRoleArn(String executionRoleArn) {
if (executionRoleArn == null) {
throw new MissingRequiredPropertyException("JobTemplateJobTemplateData", "executionRoleArn");
}
this.executionRoleArn = executionRoleArn;
return this;
}
@CustomType.Setter
public Builder jobDriver(JobTemplateJobTemplateDataJobDriver jobDriver) {
if (jobDriver == null) {
throw new MissingRequiredPropertyException("JobTemplateJobTemplateData", "jobDriver");
}
this.jobDriver = jobDriver;
return this;
}
@CustomType.Setter
public Builder jobTags(@Nullable Map jobTags) {
this.jobTags = jobTags;
return this;
}
@CustomType.Setter
public Builder releaseLabel(String releaseLabel) {
if (releaseLabel == null) {
throw new MissingRequiredPropertyException("JobTemplateJobTemplateData", "releaseLabel");
}
this.releaseLabel = releaseLabel;
return this;
}
public JobTemplateJobTemplateData build() {
final var _resultValue = new JobTemplateJobTemplateData();
_resultValue.configurationOverrides = configurationOverrides;
_resultValue.executionRoleArn = executionRoleArn;
_resultValue.jobDriver = jobDriver;
_resultValue.jobTags = jobTags;
_resultValue.releaseLabel = releaseLabel;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy