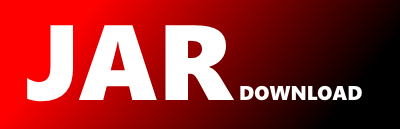
com.pulumi.aws.finspace.inputs.KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.finspace.inputs;
import com.pulumi.aws.finspace.inputs.KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationIcmpTypeCodeArgs;
import com.pulumi.aws.finspace.inputs.KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationPortRangeArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationArgs Empty = new KxEnvironmentTransitGatewayConfigurationAttachmentNetworkAclConfigurationArgs();
/**
* The IPv4 network range to allow or deny, in CIDR notation. The specified CIDR block is modified to its canonical form. For example, `100.68.0.18/18` will be converted to `100.68.0.0/18`.
*
*/
@Import(name="cidrBlock", required=true)
private Output cidrBlock;
/**
* @return The IPv4 network range to allow or deny, in CIDR notation. The specified CIDR block is modified to its canonical form. For example, `100.68.0.18/18` will be converted to `100.68.0.0/18`.
*
*/
public Output cidrBlock() {
return this.cidrBlock;
}
/**
* Defines the ICMP protocol that consists of the ICMP type and code. Defined below.
*
*/
@Import(name="icmpTypeCode")
private @Nullable Output icmpTypeCode;
/**
* @return Defines the ICMP protocol that consists of the ICMP type and code. Defined below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy