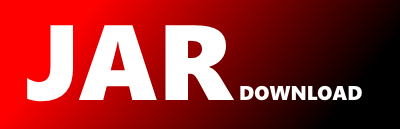
com.pulumi.aws.imagebuilder.ContainerRecipeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.imagebuilder;
import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeComponentArgs;
import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeInstanceConfigurationArgs;
import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeTargetRepositoryArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ContainerRecipeArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerRecipeArgs Empty = new ContainerRecipeArgs();
/**
* Ordered configuration block(s) with components for the container recipe. Detailed below.
*
*/
@Import(name="components", required=true)
private Output> components;
/**
* @return Ordered configuration block(s) with components for the container recipe. Detailed below.
*
*/
public Output> components() {
return this.components;
}
/**
* The type of the container to create. Valid values: `DOCKER`.
*
*/
@Import(name="containerType", required=true)
private Output containerType;
/**
* @return The type of the container to create. Valid values: `DOCKER`.
*
*/
public Output containerType() {
return this.containerType;
}
/**
* The description of the container recipe.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return The description of the container recipe.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy