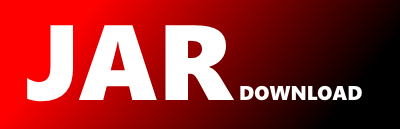
com.pulumi.aws.lambda.Alias Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lambda;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.lambda.AliasArgs;
import com.pulumi.aws.lambda.inputs.AliasState;
import com.pulumi.aws.lambda.outputs.AliasRoutingConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a Lambda function alias. Creates an alias that points to the specified Lambda function version.
*
* For information about Lambda and how to use it, see [What is AWS Lambda?](http://docs.aws.amazon.com/lambda/latest/dg/welcome.html)
* For information about function aliases, see [CreateAlias](http://docs.aws.amazon.com/lambda/latest/dg/API_CreateAlias.html) and [AliasRoutingConfiguration](https://docs.aws.amazon.com/lambda/latest/dg/API_AliasRoutingConfiguration.html) in the API docs.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Alias;
* import com.pulumi.aws.lambda.AliasArgs;
* import com.pulumi.aws.lambda.inputs.AliasRoutingConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var testLambdaAlias = new Alias("testLambdaAlias", AliasArgs.builder()
* .name("my_alias")
* .description("a sample description")
* .functionName(lambdaFunctionTest.arn())
* .functionVersion("1")
* .routingConfig(AliasRoutingConfigArgs.builder()
* .additionalVersionWeights(Map.of("2", 0.5))
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Lambda Function Aliases using the `function_name/alias`. For example:
*
* ```sh
* $ pulumi import aws:lambda/alias:Alias test_lambda_alias my_test_lambda_function/my_alias
* ```
*
*/
@ResourceType(type="aws:lambda/alias:Alias")
public class Alias extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) identifying your Lambda function alias.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) identifying your Lambda function alias.
*
*/
public Output arn() {
return this.arn;
}
/**
* Description of the alias.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the alias.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Lambda Function name or ARN.
*
*/
@Export(name="functionName", refs={String.class}, tree="[0]")
private Output functionName;
/**
* @return Lambda Function name or ARN.
*
*/
public Output functionName() {
return this.functionName;
}
/**
* Lambda function version for which you are creating the alias. Pattern: `(\$LATEST|[0-9]+)`.
*
*/
@Export(name="functionVersion", refs={String.class}, tree="[0]")
private Output functionVersion;
/**
* @return Lambda function version for which you are creating the alias. Pattern: `(\$LATEST|[0-9]+)`.
*
*/
public Output functionVersion() {
return this.functionVersion;
}
/**
* The ARN to be used for invoking Lambda Function from API Gateway - to be used in `aws.apigateway.Integration`'s `uri`
*
*/
@Export(name="invokeArn", refs={String.class}, tree="[0]")
private Output invokeArn;
/**
* @return The ARN to be used for invoking Lambda Function from API Gateway - to be used in `aws.apigateway.Integration`'s `uri`
*
*/
public Output invokeArn() {
return this.invokeArn;
}
/**
* Name for the alias you are creating. Pattern: `(?!^[0-9]+$)([a-zA-Z0-9-_]+)`
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name for the alias you are creating. Pattern: `(?!^[0-9]+$)([a-zA-Z0-9-_]+)`
*
*/
public Output name() {
return this.name;
}
/**
* The Lambda alias' route configuration settings. Fields documented below
*
*/
@Export(name="routingConfig", refs={AliasRoutingConfig.class}, tree="[0]")
private Output* @Nullable */ AliasRoutingConfig> routingConfig;
/**
* @return The Lambda alias' route configuration settings. Fields documented below
*
*/
public Output> routingConfig() {
return Codegen.optional(this.routingConfig);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Alias(java.lang.String name) {
this(name, AliasArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Alias(java.lang.String name, AliasArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Alias(java.lang.String name, AliasArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/alias:Alias", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Alias(java.lang.String name, Output id, @Nullable AliasState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/alias:Alias", name, state, makeResourceOptions(options, id), false);
}
private static AliasArgs makeArgs(AliasArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AliasArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Alias get(java.lang.String name, Output id, @Nullable AliasState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Alias(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy