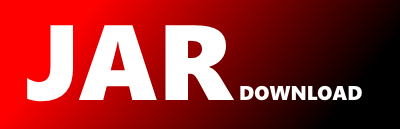
com.pulumi.aws.lambda.outputs.GetFunctionUrlResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lambda.outputs;
import com.pulumi.aws.lambda.outputs.GetFunctionUrlCor;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetFunctionUrlResult {
/**
* @return Type of authentication that the function URL uses.
*
*/
private String authorizationType;
/**
* @return The [cross-origin resource sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for the function URL. See the `aws.lambda.FunctionUrl` resource documentation for more details.
*
*/
private List cors;
/**
* @return When the function URL was created, in [ISO-8601 format](https://www.w3.org/TR/NOTE-datetime).
*
*/
private String creationTime;
/**
* @return ARN of the function.
*
*/
private String functionArn;
private String functionName;
/**
* @return HTTP URL endpoint for the function in the format `https://<url_id>.lambda-url.<region>.on.aws/`.
*
*/
private String functionUrl;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Whether the Lambda function responds in `BUFFERED` or `RESPONSE_STREAM` mode.
*
*/
private String invokeMode;
/**
* @return When the function URL configuration was last updated, in [ISO-8601 format](https://www.w3.org/TR/NOTE-datetime).
*
*/
private String lastModifiedTime;
private @Nullable String qualifier;
/**
* @return Generated ID for the endpoint.
*
*/
private String urlId;
private GetFunctionUrlResult() {}
/**
* @return Type of authentication that the function URL uses.
*
*/
public String authorizationType() {
return this.authorizationType;
}
/**
* @return The [cross-origin resource sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for the function URL. See the `aws.lambda.FunctionUrl` resource documentation for more details.
*
*/
public List cors() {
return this.cors;
}
/**
* @return When the function URL was created, in [ISO-8601 format](https://www.w3.org/TR/NOTE-datetime).
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return ARN of the function.
*
*/
public String functionArn() {
return this.functionArn;
}
public String functionName() {
return this.functionName;
}
/**
* @return HTTP URL endpoint for the function in the format `https://<url_id>.lambda-url.<region>.on.aws/`.
*
*/
public String functionUrl() {
return this.functionUrl;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Whether the Lambda function responds in `BUFFERED` or `RESPONSE_STREAM` mode.
*
*/
public String invokeMode() {
return this.invokeMode;
}
/**
* @return When the function URL configuration was last updated, in [ISO-8601 format](https://www.w3.org/TR/NOTE-datetime).
*
*/
public String lastModifiedTime() {
return this.lastModifiedTime;
}
public Optional qualifier() {
return Optional.ofNullable(this.qualifier);
}
/**
* @return Generated ID for the endpoint.
*
*/
public String urlId() {
return this.urlId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFunctionUrlResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String authorizationType;
private List cors;
private String creationTime;
private String functionArn;
private String functionName;
private String functionUrl;
private String id;
private String invokeMode;
private String lastModifiedTime;
private @Nullable String qualifier;
private String urlId;
public Builder() {}
public Builder(GetFunctionUrlResult defaults) {
Objects.requireNonNull(defaults);
this.authorizationType = defaults.authorizationType;
this.cors = defaults.cors;
this.creationTime = defaults.creationTime;
this.functionArn = defaults.functionArn;
this.functionName = defaults.functionName;
this.functionUrl = defaults.functionUrl;
this.id = defaults.id;
this.invokeMode = defaults.invokeMode;
this.lastModifiedTime = defaults.lastModifiedTime;
this.qualifier = defaults.qualifier;
this.urlId = defaults.urlId;
}
@CustomType.Setter
public Builder authorizationType(String authorizationType) {
if (authorizationType == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "authorizationType");
}
this.authorizationType = authorizationType;
return this;
}
@CustomType.Setter
public Builder cors(List cors) {
if (cors == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "cors");
}
this.cors = cors;
return this;
}
public Builder cors(GetFunctionUrlCor... cors) {
return cors(List.of(cors));
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder functionArn(String functionArn) {
if (functionArn == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "functionArn");
}
this.functionArn = functionArn;
return this;
}
@CustomType.Setter
public Builder functionName(String functionName) {
if (functionName == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "functionName");
}
this.functionName = functionName;
return this;
}
@CustomType.Setter
public Builder functionUrl(String functionUrl) {
if (functionUrl == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "functionUrl");
}
this.functionUrl = functionUrl;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder invokeMode(String invokeMode) {
if (invokeMode == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "invokeMode");
}
this.invokeMode = invokeMode;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(String lastModifiedTime) {
if (lastModifiedTime == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "lastModifiedTime");
}
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder qualifier(@Nullable String qualifier) {
this.qualifier = qualifier;
return this;
}
@CustomType.Setter
public Builder urlId(String urlId) {
if (urlId == null) {
throw new MissingRequiredPropertyException("GetFunctionUrlResult", "urlId");
}
this.urlId = urlId;
return this;
}
public GetFunctionUrlResult build() {
final var _resultValue = new GetFunctionUrlResult();
_resultValue.authorizationType = authorizationType;
_resultValue.cors = cors;
_resultValue.creationTime = creationTime;
_resultValue.functionArn = functionArn;
_resultValue.functionName = functionName;
_resultValue.functionUrl = functionUrl;
_resultValue.id = id;
_resultValue.invokeMode = invokeMode;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.qualifier = qualifier;
_resultValue.urlId = urlId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy