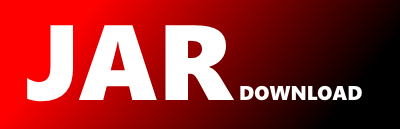
com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.inputs;
import com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationContinueResponseArgs;
import com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationStillWaitingResponseArgs;
import com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationWaitingResponseArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationArgs extends com.pulumi.resources.ResourceArgs {
public static final V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationArgs Empty = new V2modelsSlotValueElicitationSettingWaitAndContinueSpecificationArgs();
/**
* Specifies whether the bot will wait for a user to respond.
* When this field is `false`, wait and continue responses for a slot aren't used.
* If the active field isn't specified, the default is `true`.
*
*/
@Import(name="active")
private @Nullable Output active;
/**
* @return Specifies whether the bot will wait for a user to respond.
* When this field is `false`, wait and continue responses for a slot aren't used.
* If the active field isn't specified, the default is `true`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy