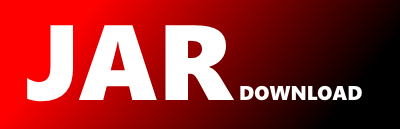
com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSetting Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.outputs;
import com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSettingDefaultValueSpecification;
import com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSettingPromptSpecification;
import com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSettingSampleUtterance;
import com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSettingSlotResolutionSetting;
import com.pulumi.aws.lex.outputs.V2modelsSlotValueElicitationSettingWaitAndContinueSpecification;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class V2modelsSlotValueElicitationSetting {
/**
* @return List of default values for a slot.
* See the `default_value_specification` argument reference below.
*
*/
private @Nullable List defaultValueSpecifications;
/**
* @return Prompt that Amazon Lex uses to elicit the slot value from the user.
* See the `aws.lex.V2modelsIntent` resource for details on the `prompt_specification` argument reference - they are identical.
*
*/
private V2modelsSlotValueElicitationSettingPromptSpecification promptSpecification;
private @Nullable List sampleUtterances;
/**
* @return Whether the slot is required or optional. Valid values are `Required` or `Optional`.
*
*/
private String slotConstraint;
/**
* @return Information about whether assisted slot resolution is turned on for the slot or not.
* See the `slot_resolution_setting` argument reference below.
*
*/
private @Nullable List slotResolutionSettings;
/**
* @return Specifies the prompts that Amazon Lex uses while a bot is waiting for customer input.
* See the `wait_and_continue_specification` argument reference below.
*
*/
private @Nullable List waitAndContinueSpecifications;
private V2modelsSlotValueElicitationSetting() {}
/**
* @return List of default values for a slot.
* See the `default_value_specification` argument reference below.
*
*/
public List defaultValueSpecifications() {
return this.defaultValueSpecifications == null ? List.of() : this.defaultValueSpecifications;
}
/**
* @return Prompt that Amazon Lex uses to elicit the slot value from the user.
* See the `aws.lex.V2modelsIntent` resource for details on the `prompt_specification` argument reference - they are identical.
*
*/
public V2modelsSlotValueElicitationSettingPromptSpecification promptSpecification() {
return this.promptSpecification;
}
public List sampleUtterances() {
return this.sampleUtterances == null ? List.of() : this.sampleUtterances;
}
/**
* @return Whether the slot is required or optional. Valid values are `Required` or `Optional`.
*
*/
public String slotConstraint() {
return this.slotConstraint;
}
/**
* @return Information about whether assisted slot resolution is turned on for the slot or not.
* See the `slot_resolution_setting` argument reference below.
*
*/
public List slotResolutionSettings() {
return this.slotResolutionSettings == null ? List.of() : this.slotResolutionSettings;
}
/**
* @return Specifies the prompts that Amazon Lex uses while a bot is waiting for customer input.
* See the `wait_and_continue_specification` argument reference below.
*
*/
public List waitAndContinueSpecifications() {
return this.waitAndContinueSpecifications == null ? List.of() : this.waitAndContinueSpecifications;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(V2modelsSlotValueElicitationSetting defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List defaultValueSpecifications;
private V2modelsSlotValueElicitationSettingPromptSpecification promptSpecification;
private @Nullable List sampleUtterances;
private String slotConstraint;
private @Nullable List slotResolutionSettings;
private @Nullable List waitAndContinueSpecifications;
public Builder() {}
public Builder(V2modelsSlotValueElicitationSetting defaults) {
Objects.requireNonNull(defaults);
this.defaultValueSpecifications = defaults.defaultValueSpecifications;
this.promptSpecification = defaults.promptSpecification;
this.sampleUtterances = defaults.sampleUtterances;
this.slotConstraint = defaults.slotConstraint;
this.slotResolutionSettings = defaults.slotResolutionSettings;
this.waitAndContinueSpecifications = defaults.waitAndContinueSpecifications;
}
@CustomType.Setter
public Builder defaultValueSpecifications(@Nullable List defaultValueSpecifications) {
this.defaultValueSpecifications = defaultValueSpecifications;
return this;
}
public Builder defaultValueSpecifications(V2modelsSlotValueElicitationSettingDefaultValueSpecification... defaultValueSpecifications) {
return defaultValueSpecifications(List.of(defaultValueSpecifications));
}
@CustomType.Setter
public Builder promptSpecification(V2modelsSlotValueElicitationSettingPromptSpecification promptSpecification) {
if (promptSpecification == null) {
throw new MissingRequiredPropertyException("V2modelsSlotValueElicitationSetting", "promptSpecification");
}
this.promptSpecification = promptSpecification;
return this;
}
@CustomType.Setter
public Builder sampleUtterances(@Nullable List sampleUtterances) {
this.sampleUtterances = sampleUtterances;
return this;
}
public Builder sampleUtterances(V2modelsSlotValueElicitationSettingSampleUtterance... sampleUtterances) {
return sampleUtterances(List.of(sampleUtterances));
}
@CustomType.Setter
public Builder slotConstraint(String slotConstraint) {
if (slotConstraint == null) {
throw new MissingRequiredPropertyException("V2modelsSlotValueElicitationSetting", "slotConstraint");
}
this.slotConstraint = slotConstraint;
return this;
}
@CustomType.Setter
public Builder slotResolutionSettings(@Nullable List slotResolutionSettings) {
this.slotResolutionSettings = slotResolutionSettings;
return this;
}
public Builder slotResolutionSettings(V2modelsSlotValueElicitationSettingSlotResolutionSetting... slotResolutionSettings) {
return slotResolutionSettings(List.of(slotResolutionSettings));
}
@CustomType.Setter
public Builder waitAndContinueSpecifications(@Nullable List waitAndContinueSpecifications) {
this.waitAndContinueSpecifications = waitAndContinueSpecifications;
return this;
}
public Builder waitAndContinueSpecifications(V2modelsSlotValueElicitationSettingWaitAndContinueSpecification... waitAndContinueSpecifications) {
return waitAndContinueSpecifications(List.of(waitAndContinueSpecifications));
}
public V2modelsSlotValueElicitationSetting build() {
final var _resultValue = new V2modelsSlotValueElicitationSetting();
_resultValue.defaultValueSpecifications = defaultValueSpecifications;
_resultValue.promptSpecification = promptSpecification;
_resultValue.sampleUtterances = sampleUtterances;
_resultValue.slotConstraint = slotConstraint;
_resultValue.slotResolutionSettings = slotResolutionSettings;
_resultValue.waitAndContinueSpecifications = waitAndContinueSpecifications;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy