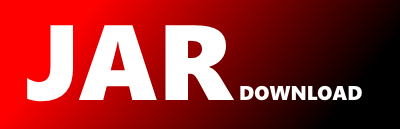
com.pulumi.aws.neptune.Cluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.neptune;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.neptune.ClusterArgs;
import com.pulumi.aws.neptune.inputs.ClusterState;
import com.pulumi.aws.neptune.outputs.ClusterServerlessV2ScalingConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Neptune Cluster Resource. A Cluster Resource defines attributes that are
* applied to the entire cluster of Neptune Cluster Instances.
*
* Changes to a Neptune Cluster can occur when you manually change a
* parameter, such as `backup_retention_period`, and are reflected in the next maintenance
* window. Because of this, this provider may report a difference in its planning
* phase because a modification has not yet taken place. You can use the
* `apply_immediately` flag to instruct the service to apply the change immediately
* (see documentation below).
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.neptune.Cluster;
* import com.pulumi.aws.neptune.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("neptune-cluster-demo")
* .engine("neptune")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .skipFinalSnapshot(true)
* .iamDatabaseAuthenticationEnabled(true)
* .applyImmediately(true)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* > **Note:** AWS Neptune does not support user name/password–based access control.
* See the AWS [Docs](https://docs.aws.amazon.com/neptune/latest/userguide/limits.html) for more information.
*
* ## Import
*
* Using `pulumi import`, import `aws_neptune_cluster` using the cluster identifier. For example:
*
* ```sh
* $ pulumi import aws:neptune/cluster:Cluster example my-cluster
* ```
*
*/
@ResourceType(type="aws:neptune/cluster:Cluster")
public class Cluster extends com.pulumi.resources.CustomResource {
/**
* Specifies whether upgrades between different major versions are allowed. You must set it to `true` when providing an `engine_version` parameter that uses a different major version than the DB cluster's current version. Default is `false`.
*
*/
@Export(name="allowMajorVersionUpgrade", refs={Boolean.class}, tree="[0]")
private Output allowMajorVersionUpgrade;
/**
* @return Specifies whether upgrades between different major versions are allowed. You must set it to `true` when providing an `engine_version` parameter that uses a different major version than the DB cluster's current version. Default is `false`.
*
*/
public Output allowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
* Specifies whether any cluster modifications are applied immediately, or during the next maintenance window. Default is `false`.
*
*/
@Export(name="applyImmediately", refs={Boolean.class}, tree="[0]")
private Output applyImmediately;
/**
* @return Specifies whether any cluster modifications are applied immediately, or during the next maintenance window. Default is `false`.
*
*/
public Output applyImmediately() {
return this.applyImmediately;
}
/**
* The Neptune Cluster Amazon Resource Name (ARN)
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Neptune Cluster Amazon Resource Name (ARN)
*
*/
public Output arn() {
return this.arn;
}
/**
* A list of EC2 Availability Zones that instances in the Neptune cluster can be created in.
*
*/
@Export(name="availabilityZones", refs={List.class,String.class}, tree="[0,1]")
private Output> availabilityZones;
/**
* @return A list of EC2 Availability Zones that instances in the Neptune cluster can be created in.
*
*/
public Output> availabilityZones() {
return this.availabilityZones;
}
/**
* The days to retain backups for. Default `1`
*
*/
@Export(name="backupRetentionPeriod", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> backupRetentionPeriod;
/**
* @return The days to retain backups for. Default `1`
*
*/
public Output> backupRetentionPeriod() {
return Codegen.optional(this.backupRetentionPeriod);
}
/**
* The cluster identifier. If omitted, this provider will assign a random, unique identifier.
*
*/
@Export(name="clusterIdentifier", refs={String.class}, tree="[0]")
private Output clusterIdentifier;
/**
* @return The cluster identifier. If omitted, this provider will assign a random, unique identifier.
*
*/
public Output clusterIdentifier() {
return this.clusterIdentifier;
}
/**
* Creates a unique cluster identifier beginning with the specified prefix. Conflicts with `cluster_identifier`.
*
*/
@Export(name="clusterIdentifierPrefix", refs={String.class}, tree="[0]")
private Output clusterIdentifierPrefix;
/**
* @return Creates a unique cluster identifier beginning with the specified prefix. Conflicts with `cluster_identifier`.
*
*/
public Output clusterIdentifierPrefix() {
return this.clusterIdentifierPrefix;
}
/**
* List of Neptune Instances that are a part of this cluster
*
*/
@Export(name="clusterMembers", refs={List.class,String.class}, tree="[0,1]")
private Output> clusterMembers;
/**
* @return List of Neptune Instances that are a part of this cluster
*
*/
public Output> clusterMembers() {
return this.clusterMembers;
}
/**
* The Neptune Cluster Resource ID
*
*/
@Export(name="clusterResourceId", refs={String.class}, tree="[0]")
private Output clusterResourceId;
/**
* @return The Neptune Cluster Resource ID
*
*/
public Output clusterResourceId() {
return this.clusterResourceId;
}
/**
* If set to true, tags are copied to any snapshot of the DB cluster that is created.
*
*/
@Export(name="copyTagsToSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> copyTagsToSnapshot;
/**
* @return If set to true, tags are copied to any snapshot of the DB cluster that is created.
*
*/
public Output> copyTagsToSnapshot() {
return Codegen.optional(this.copyTagsToSnapshot);
}
/**
* A value that indicates whether the DB cluster has deletion protection enabled.The database can't be deleted when deletion protection is enabled. By default, deletion protection is disabled.
*
*/
@Export(name="deletionProtection", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> deletionProtection;
/**
* @return A value that indicates whether the DB cluster has deletion protection enabled.The database can't be deleted when deletion protection is enabled. By default, deletion protection is disabled.
*
*/
public Output> deletionProtection() {
return Codegen.optional(this.deletionProtection);
}
/**
* A list of the log types this DB cluster is configured to export to Cloudwatch Logs. Currently only supports `audit` and `slowquery`.
*
*/
@Export(name="enableCloudwatchLogsExports", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> enableCloudwatchLogsExports;
/**
* @return A list of the log types this DB cluster is configured to export to Cloudwatch Logs. Currently only supports `audit` and `slowquery`.
*
*/
public Output>> enableCloudwatchLogsExports() {
return Codegen.optional(this.enableCloudwatchLogsExports);
}
/**
* The DNS address of the Neptune instance
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return The DNS address of the Neptune instance
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* The name of the database engine to be used for this Neptune cluster. Defaults to `neptune`.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> engine;
/**
* @return The name of the database engine to be used for this Neptune cluster. Defaults to `neptune`.
*
*/
public Output> engine() {
return Codegen.optional(this.engine);
}
/**
* The database engine version.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return The database engine version.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
/**
* The name of your final Neptune snapshot when this Neptune cluster is deleted. If omitted, no final snapshot will be made.
*
*/
@Export(name="finalSnapshotIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> finalSnapshotIdentifier;
/**
* @return The name of your final Neptune snapshot when this Neptune cluster is deleted. If omitted, no final snapshot will be made.
*
*/
public Output> finalSnapshotIdentifier() {
return Codegen.optional(this.finalSnapshotIdentifier);
}
/**
* The global cluster identifier specified on `aws.neptune.GlobalCluster`.
*
*/
@Export(name="globalClusterIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> globalClusterIdentifier;
/**
* @return The global cluster identifier specified on `aws.neptune.GlobalCluster`.
*
*/
public Output> globalClusterIdentifier() {
return Codegen.optional(this.globalClusterIdentifier);
}
/**
* The Route53 Hosted Zone ID of the endpoint
*
*/
@Export(name="hostedZoneId", refs={String.class}, tree="[0]")
private Output hostedZoneId;
/**
* @return The Route53 Hosted Zone ID of the endpoint
*
*/
public Output hostedZoneId() {
return this.hostedZoneId;
}
/**
* Specifies whether or not mappings of AWS Identity and Access Management (IAM) accounts to database accounts is enabled.
*
*/
@Export(name="iamDatabaseAuthenticationEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> iamDatabaseAuthenticationEnabled;
/**
* @return Specifies whether or not mappings of AWS Identity and Access Management (IAM) accounts to database accounts is enabled.
*
*/
public Output> iamDatabaseAuthenticationEnabled() {
return Codegen.optional(this.iamDatabaseAuthenticationEnabled);
}
/**
* A List of ARNs for the IAM roles to associate to the Neptune Cluster.
*
*/
@Export(name="iamRoles", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> iamRoles;
/**
* @return A List of ARNs for the IAM roles to associate to the Neptune Cluster.
*
*/
public Output>> iamRoles() {
return Codegen.optional(this.iamRoles);
}
/**
* The ARN for the KMS encryption key. When specifying `kms_key_arn`, `storage_encrypted` needs to be set to true.
*
*/
@Export(name="kmsKeyArn", refs={String.class}, tree="[0]")
private Output kmsKeyArn;
/**
* @return The ARN for the KMS encryption key. When specifying `kms_key_arn`, `storage_encrypted` needs to be set to true.
*
*/
public Output kmsKeyArn() {
return this.kmsKeyArn;
}
/**
* A cluster parameter group to associate with the cluster.
*
*/
@Export(name="neptuneClusterParameterGroupName", refs={String.class}, tree="[0]")
private Output neptuneClusterParameterGroupName;
/**
* @return A cluster parameter group to associate with the cluster.
*
*/
public Output neptuneClusterParameterGroupName() {
return this.neptuneClusterParameterGroupName;
}
/**
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*/
@Export(name="neptuneInstanceParameterGroupName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> neptuneInstanceParameterGroupName;
/**
* @return The name of the DB parameter group to apply to all instances of the DB cluster.
*
*/
public Output> neptuneInstanceParameterGroupName() {
return Codegen.optional(this.neptuneInstanceParameterGroupName);
}
/**
* A Neptune subnet group to associate with this Neptune instance.
*
*/
@Export(name="neptuneSubnetGroupName", refs={String.class}, tree="[0]")
private Output neptuneSubnetGroupName;
/**
* @return A Neptune subnet group to associate with this Neptune instance.
*
*/
public Output neptuneSubnetGroupName() {
return this.neptuneSubnetGroupName;
}
/**
* The port on which the Neptune accepts connections. Default is `8182`.
*
*/
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> port;
/**
* @return The port on which the Neptune accepts connections. Default is `8182`.
*
*/
public Output> port() {
return Codegen.optional(this.port);
}
/**
* The daily time range during which automated backups are created if automated backups are enabled using the BackupRetentionPeriod parameter. Time in UTC. Default: A 30-minute window selected at random from an 8-hour block of time per regionE.g., 04:00-09:00
*
*/
@Export(name="preferredBackupWindow", refs={String.class}, tree="[0]")
private Output preferredBackupWindow;
/**
* @return The daily time range during which automated backups are created if automated backups are enabled using the BackupRetentionPeriod parameter. Time in UTC. Default: A 30-minute window selected at random from an 8-hour block of time per regionE.g., 04:00-09:00
*
*/
public Output preferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
* The weekly time range during which system maintenance can occur, in (UTC) e.g., wed:04:00-wed:04:30
*
*/
@Export(name="preferredMaintenanceWindow", refs={String.class}, tree="[0]")
private Output preferredMaintenanceWindow;
/**
* @return The weekly time range during which system maintenance can occur, in (UTC) e.g., wed:04:00-wed:04:30
*
*/
public Output preferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
* A read-only endpoint for the Neptune cluster, automatically load-balanced across replicas
*
*/
@Export(name="readerEndpoint", refs={String.class}, tree="[0]")
private Output readerEndpoint;
/**
* @return A read-only endpoint for the Neptune cluster, automatically load-balanced across replicas
*
*/
public Output readerEndpoint() {
return this.readerEndpoint;
}
/**
* ARN of a source Neptune cluster or Neptune instance if this Neptune cluster is to be created as a Read Replica.
*
*/
@Export(name="replicationSourceIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> replicationSourceIdentifier;
/**
* @return ARN of a source Neptune cluster or Neptune instance if this Neptune cluster is to be created as a Read Replica.
*
*/
public Output> replicationSourceIdentifier() {
return Codegen.optional(this.replicationSourceIdentifier);
}
/**
* If set, create the Neptune cluster as a serverless one. See Serverless for example block attributes.
*
*/
@Export(name="serverlessV2ScalingConfiguration", refs={ClusterServerlessV2ScalingConfiguration.class}, tree="[0]")
private Output* @Nullable */ ClusterServerlessV2ScalingConfiguration> serverlessV2ScalingConfiguration;
/**
* @return If set, create the Neptune cluster as a serverless one. See Serverless for example block attributes.
*
*/
public Output> serverlessV2ScalingConfiguration() {
return Codegen.optional(this.serverlessV2ScalingConfiguration);
}
/**
* Determines whether a final Neptune snapshot is created before the Neptune cluster is deleted. If true is specified, no Neptune snapshot is created. If false is specified, a Neptune snapshot is created before the Neptune cluster is deleted, using the value from `final_snapshot_identifier`. Default is `false`.
*
*/
@Export(name="skipFinalSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipFinalSnapshot;
/**
* @return Determines whether a final Neptune snapshot is created before the Neptune cluster is deleted. If true is specified, no Neptune snapshot is created. If false is specified, a Neptune snapshot is created before the Neptune cluster is deleted, using the value from `final_snapshot_identifier`. Default is `false`.
*
*/
public Output> skipFinalSnapshot() {
return Codegen.optional(this.skipFinalSnapshot);
}
/**
* Specifies whether or not to create this cluster from a snapshot. You can use either the name or ARN when specifying a Neptune cluster snapshot, or the ARN when specifying a Neptune snapshot. Automated snapshots **should not** be used for this attribute, unless from a different cluster. Automated snapshots are deleted as part of cluster destruction when the resource is replaced.
*
*/
@Export(name="snapshotIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> snapshotIdentifier;
/**
* @return Specifies whether or not to create this cluster from a snapshot. You can use either the name or ARN when specifying a Neptune cluster snapshot, or the ARN when specifying a Neptune snapshot. Automated snapshots **should not** be used for this attribute, unless from a different cluster. Automated snapshots are deleted as part of cluster destruction when the resource is replaced.
*
*/
public Output> snapshotIdentifier() {
return Codegen.optional(this.snapshotIdentifier);
}
/**
* Specifies whether the Neptune cluster is encrypted. The default is `false` if not specified.
*
*/
@Export(name="storageEncrypted", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> storageEncrypted;
/**
* @return Specifies whether the Neptune cluster is encrypted. The default is `false` if not specified.
*
*/
public Output> storageEncrypted() {
return Codegen.optional(this.storageEncrypted);
}
/**
* Storage type associated with the cluster `standard/iopt1`. Default: `standard`
*
*/
@Export(name="storageType", refs={String.class}, tree="[0]")
private Output storageType;
/**
* @return Storage type associated with the cluster `standard/iopt1`. Default: `standard`
*
*/
public Output storageType() {
return this.storageType;
}
/**
* A map of tags to assign to the Neptune cluster. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the Neptune cluster. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy