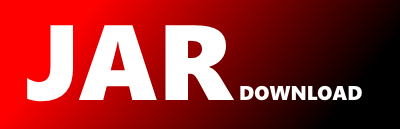
com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.inputs;
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleDeleteMarkerReplicationArgs;
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleDestinationArgs;
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleExistingObjectReplicationArgs;
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleFilterArgs;
import com.pulumi.aws.s3.inputs.BucketReplicationConfigRuleSourceSelectionCriteriaArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BucketReplicationConfigRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final BucketReplicationConfigRuleArgs Empty = new BucketReplicationConfigRuleArgs();
/**
* Whether delete markers are replicated. This argument is only valid with V2 replication configurations (i.e., when `filter` is used)documented below.
*
*/
@Import(name="deleteMarkerReplication")
private @Nullable Output deleteMarkerReplication;
/**
* @return Whether delete markers are replicated. This argument is only valid with V2 replication configurations (i.e., when `filter` is used)documented below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy