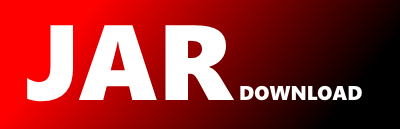
com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3.outputs;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestinationAccessControlTranslation;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestinationEncryptionConfiguration;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestinationMetrics;
import com.pulumi.aws.s3.outputs.BucketReplicationConfigRuleDestinationReplicationTime;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BucketReplicationConfigRuleDestination {
/**
* @return Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
*
*/
private @Nullable BucketReplicationConfigRuleDestinationAccessControlTranslation accessControlTranslation;
/**
* @return Account ID to specify the replica ownership. Must be used in conjunction with `access_control_translation` override configuration.
*
*/
private @Nullable String account;
/**
* @return ARN of the bucket where you want Amazon S3 to store the results.
*
*/
private String bucket;
/**
* @return Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
*
*/
private @Nullable BucketReplicationConfigRuleDestinationEncryptionConfiguration encryptionConfiguration;
/**
* @return Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
*
*/
private @Nullable BucketReplicationConfigRuleDestinationMetrics metrics;
/**
* @return Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
*
*/
private @Nullable BucketReplicationConfigRuleDestinationReplicationTime replicationTime;
/**
* @return The [storage class](https://docs.aws.amazon.com/AmazonS3/latest/API/API_Destination.html#AmazonS3-Type-Destination-StorageClass) used to store the object. By default, Amazon S3 uses the storage class of the source object to create the object replica.
*
*/
private @Nullable String storageClass;
private BucketReplicationConfigRuleDestination() {}
/**
* @return Configuration block that specifies the overrides to use for object owners on replication. See below. Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS account that owns the source object. Must be used in conjunction with `account` owner override configuration.
*
*/
public Optional accessControlTranslation() {
return Optional.ofNullable(this.accessControlTranslation);
}
/**
* @return Account ID to specify the replica ownership. Must be used in conjunction with `access_control_translation` override configuration.
*
*/
public Optional account() {
return Optional.ofNullable(this.account);
}
/**
* @return ARN of the bucket where you want Amazon S3 to store the results.
*
*/
public String bucket() {
return this.bucket;
}
/**
* @return Configuration block that provides information about encryption. See below. If `source_selection_criteria` is specified, you must specify this element.
*
*/
public Optional encryptionConfiguration() {
return Optional.ofNullable(this.encryptionConfiguration);
}
/**
* @return Configuration block that specifies replication metrics-related settings enabling replication metrics and events. See below.
*
*/
public Optional metrics() {
return Optional.ofNullable(this.metrics);
}
/**
* @return Configuration block that specifies S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. See below. Replication Time Control must be used in conjunction with `metrics`.
*
*/
public Optional replicationTime() {
return Optional.ofNullable(this.replicationTime);
}
/**
* @return The [storage class](https://docs.aws.amazon.com/AmazonS3/latest/API/API_Destination.html#AmazonS3-Type-Destination-StorageClass) used to store the object. By default, Amazon S3 uses the storage class of the source object to create the object replica.
*
*/
public Optional storageClass() {
return Optional.ofNullable(this.storageClass);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BucketReplicationConfigRuleDestination defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable BucketReplicationConfigRuleDestinationAccessControlTranslation accessControlTranslation;
private @Nullable String account;
private String bucket;
private @Nullable BucketReplicationConfigRuleDestinationEncryptionConfiguration encryptionConfiguration;
private @Nullable BucketReplicationConfigRuleDestinationMetrics metrics;
private @Nullable BucketReplicationConfigRuleDestinationReplicationTime replicationTime;
private @Nullable String storageClass;
public Builder() {}
public Builder(BucketReplicationConfigRuleDestination defaults) {
Objects.requireNonNull(defaults);
this.accessControlTranslation = defaults.accessControlTranslation;
this.account = defaults.account;
this.bucket = defaults.bucket;
this.encryptionConfiguration = defaults.encryptionConfiguration;
this.metrics = defaults.metrics;
this.replicationTime = defaults.replicationTime;
this.storageClass = defaults.storageClass;
}
@CustomType.Setter
public Builder accessControlTranslation(@Nullable BucketReplicationConfigRuleDestinationAccessControlTranslation accessControlTranslation) {
this.accessControlTranslation = accessControlTranslation;
return this;
}
@CustomType.Setter
public Builder account(@Nullable String account) {
this.account = account;
return this;
}
@CustomType.Setter
public Builder bucket(String bucket) {
if (bucket == null) {
throw new MissingRequiredPropertyException("BucketReplicationConfigRuleDestination", "bucket");
}
this.bucket = bucket;
return this;
}
@CustomType.Setter
public Builder encryptionConfiguration(@Nullable BucketReplicationConfigRuleDestinationEncryptionConfiguration encryptionConfiguration) {
this.encryptionConfiguration = encryptionConfiguration;
return this;
}
@CustomType.Setter
public Builder metrics(@Nullable BucketReplicationConfigRuleDestinationMetrics metrics) {
this.metrics = metrics;
return this;
}
@CustomType.Setter
public Builder replicationTime(@Nullable BucketReplicationConfigRuleDestinationReplicationTime replicationTime) {
this.replicationTime = replicationTime;
return this;
}
@CustomType.Setter
public Builder storageClass(@Nullable String storageClass) {
this.storageClass = storageClass;
return this;
}
public BucketReplicationConfigRuleDestination build() {
final var _resultValue = new BucketReplicationConfigRuleDestination();
_resultValue.accessControlTranslation = accessControlTranslation;
_resultValue.account = account;
_resultValue.bucket = bucket;
_resultValue.encryptionConfiguration = encryptionConfiguration;
_resultValue.metrics = metrics;
_resultValue.replicationTime = replicationTime;
_resultValue.storageClass = storageClass;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy