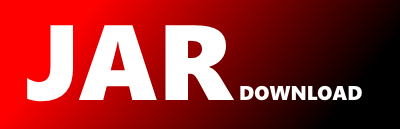
com.pulumi.aws.sagemaker.FlowDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker;
import com.pulumi.aws.sagemaker.inputs.FlowDefinitionHumanLoopActivationConfigArgs;
import com.pulumi.aws.sagemaker.inputs.FlowDefinitionHumanLoopConfigArgs;
import com.pulumi.aws.sagemaker.inputs.FlowDefinitionHumanLoopRequestSourceArgs;
import com.pulumi.aws.sagemaker.inputs.FlowDefinitionOutputConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FlowDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final FlowDefinitionArgs Empty = new FlowDefinitionArgs();
/**
* The name of your flow definition.
*
*/
@Import(name="flowDefinitionName", required=true)
private Output flowDefinitionName;
/**
* @return The name of your flow definition.
*
*/
public Output flowDefinitionName() {
return this.flowDefinitionName;
}
/**
* An object containing information about the events that trigger a human workflow. See Human Loop Activation Config details below.
*
*/
@Import(name="humanLoopActivationConfig")
private @Nullable Output humanLoopActivationConfig;
/**
* @return An object containing information about the events that trigger a human workflow. See Human Loop Activation Config details below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy