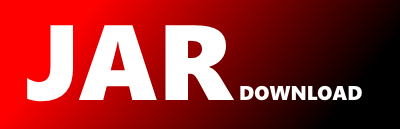
com.pulumi.aws.sagemaker.NotebookInstance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.sagemaker.NotebookInstanceArgs;
import com.pulumi.aws.sagemaker.inputs.NotebookInstanceState;
import com.pulumi.aws.sagemaker.outputs.NotebookInstanceInstanceMetadataServiceConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a SageMaker Notebook Instance resource.
*
* ## Example Usage
*
* ### Basic usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sagemaker.NotebookInstance;
* import com.pulumi.aws.sagemaker.NotebookInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ni = new NotebookInstance("ni", NotebookInstanceArgs.builder()
* .name("my-notebook-instance")
* .roleArn(role.arn())
* .instanceType("ml.t2.medium")
* .tags(Map.of("Name", "foo"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Code repository usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sagemaker.CodeRepository;
* import com.pulumi.aws.sagemaker.CodeRepositoryArgs;
* import com.pulumi.aws.sagemaker.inputs.CodeRepositoryGitConfigArgs;
* import com.pulumi.aws.sagemaker.NotebookInstance;
* import com.pulumi.aws.sagemaker.NotebookInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new CodeRepository("example", CodeRepositoryArgs.builder()
* .codeRepositoryName("my-notebook-instance-code-repo")
* .gitConfig(CodeRepositoryGitConfigArgs.builder()
* .repositoryUrl("https://github.com/github/docs.git")
* .build())
* .build());
*
* var ni = new NotebookInstance("ni", NotebookInstanceArgs.builder()
* .name("my-notebook-instance")
* .roleArn(role.arn())
* .instanceType("ml.t2.medium")
* .defaultCodeRepository(example.codeRepositoryName())
* .tags(Map.of("Name", "foo"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import SageMaker Notebook Instances using the `name`. For example:
*
* ```sh
* $ pulumi import aws:sagemaker/notebookInstance:NotebookInstance test_notebook_instance my-notebook-instance
* ```
*
*/
@ResourceType(type="aws:sagemaker/notebookInstance:NotebookInstance")
public class NotebookInstance extends com.pulumi.resources.CustomResource {
/**
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. See [Elastic Inference Accelerator](https://docs.aws.amazon.com/sagemaker/latest/dg/ei.html) for more details. Valid values: `ml.eia1.medium`, `ml.eia1.large`, `ml.eia1.xlarge`, `ml.eia2.medium`, `ml.eia2.large`, `ml.eia2.xlarge`.
*
*/
@Export(name="acceleratorTypes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> acceleratorTypes;
/**
* @return A list of Elastic Inference (EI) instance types to associate with this notebook instance. See [Elastic Inference Accelerator](https://docs.aws.amazon.com/sagemaker/latest/dg/ei.html) for more details. Valid values: `ml.eia1.medium`, `ml.eia1.large`, `ml.eia1.xlarge`, `ml.eia2.medium`, `ml.eia2.large`, `ml.eia2.xlarge`.
*
*/
public Output>> acceleratorTypes() {
return Codegen.optional(this.acceleratorTypes);
}
/**
* An array of up to three Git repositories to associate with the notebook instance.
* These can be either the names of Git repositories stored as resources in your account, or the URL of Git repositories in [AWS CodeCommit](https://docs.aws.amazon.com/codecommit/latest/userguide/welcome.html) or in any other Git repository. These repositories are cloned at the same level as the default repository of your notebook instance.
*
*/
@Export(name="additionalCodeRepositories", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> additionalCodeRepositories;
/**
* @return An array of up to three Git repositories to associate with the notebook instance.
* These can be either the names of Git repositories stored as resources in your account, or the URL of Git repositories in [AWS CodeCommit](https://docs.aws.amazon.com/codecommit/latest/userguide/welcome.html) or in any other Git repository. These repositories are cloned at the same level as the default repository of your notebook instance.
*
*/
public Output>> additionalCodeRepositories() {
return Codegen.optional(this.additionalCodeRepositories);
}
/**
* The Amazon Resource Name (ARN) assigned by AWS to this notebook instance.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) assigned by AWS to this notebook instance.
*
*/
public Output arn() {
return this.arn;
}
/**
* The Git repository associated with the notebook instance as its default code repository. This can be either the name of a Git repository stored as a resource in your account, or the URL of a Git repository in [AWS CodeCommit](https://docs.aws.amazon.com/codecommit/latest/userguide/welcome.html) or in any other Git repository.
*
*/
@Export(name="defaultCodeRepository", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> defaultCodeRepository;
/**
* @return The Git repository associated with the notebook instance as its default code repository. This can be either the name of a Git repository stored as a resource in your account, or the URL of a Git repository in [AWS CodeCommit](https://docs.aws.amazon.com/codecommit/latest/userguide/welcome.html) or in any other Git repository.
*
*/
public Output> defaultCodeRepository() {
return Codegen.optional(this.defaultCodeRepository);
}
/**
* Set to `Disabled` to disable internet access to notebook. Requires `security_groups` and `subnet_id` to be set. Supported values: `Enabled` (Default) or `Disabled`. If set to `Disabled`, the notebook instance will be able to access resources only in your VPC, and will not be able to connect to Amazon SageMaker training and endpoint services unless your configure a NAT Gateway in your VPC.
*
*/
@Export(name="directInternetAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> directInternetAccess;
/**
* @return Set to `Disabled` to disable internet access to notebook. Requires `security_groups` and `subnet_id` to be set. Supported values: `Enabled` (Default) or `Disabled`. If set to `Disabled`, the notebook instance will be able to access resources only in your VPC, and will not be able to connect to Amazon SageMaker training and endpoint services unless your configure a NAT Gateway in your VPC.
*
*/
public Output> directInternetAccess() {
return Codegen.optional(this.directInternetAccess);
}
/**
* Information on the IMDS configuration of the notebook instance. Conflicts with `instance_metadata_service_configuration`. see details below.
*
*/
@Export(name="instanceMetadataServiceConfiguration", refs={NotebookInstanceInstanceMetadataServiceConfiguration.class}, tree="[0]")
private Output* @Nullable */ NotebookInstanceInstanceMetadataServiceConfiguration> instanceMetadataServiceConfiguration;
/**
* @return Information on the IMDS configuration of the notebook instance. Conflicts with `instance_metadata_service_configuration`. see details below.
*
*/
public Output> instanceMetadataServiceConfiguration() {
return Codegen.optional(this.instanceMetadataServiceConfiguration);
}
/**
* The name of ML compute instance type.
*
*/
@Export(name="instanceType", refs={String.class}, tree="[0]")
private Output instanceType;
/**
* @return The name of ML compute instance type.
*
*/
public Output instanceType() {
return this.instanceType;
}
/**
* The AWS Key Management Service (AWS KMS) key that Amazon SageMaker uses to encrypt the model artifacts at rest using Amazon S3 server-side encryption.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsKeyId;
/**
* @return The AWS Key Management Service (AWS KMS) key that Amazon SageMaker uses to encrypt the model artifacts at rest using Amazon S3 server-side encryption.
*
*/
public Output> kmsKeyId() {
return Codegen.optional(this.kmsKeyId);
}
/**
* The name of a lifecycle configuration to associate with the notebook instance.
*
*/
@Export(name="lifecycleConfigName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lifecycleConfigName;
/**
* @return The name of a lifecycle configuration to associate with the notebook instance.
*
*/
public Output> lifecycleConfigName() {
return Codegen.optional(this.lifecycleConfigName);
}
/**
* The name of the notebook instance (must be unique).
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the notebook instance (must be unique).
*
*/
public Output name() {
return this.name;
}
/**
* The network interface ID that Amazon SageMaker created at the time of creating the instance. Only available when setting `subnet_id`.
*
*/
@Export(name="networkInterfaceId", refs={String.class}, tree="[0]")
private Output networkInterfaceId;
/**
* @return The network interface ID that Amazon SageMaker created at the time of creating the instance. Only available when setting `subnet_id`.
*
*/
public Output networkInterfaceId() {
return this.networkInterfaceId;
}
/**
* The platform identifier of the notebook instance runtime environment. This value can be either `notebook-al1-v1`, `notebook-al2-v1`, or `notebook-al2-v2`, depending on which version of Amazon Linux you require.
*
*/
@Export(name="platformIdentifier", refs={String.class}, tree="[0]")
private Output platformIdentifier;
/**
* @return The platform identifier of the notebook instance runtime environment. This value can be either `notebook-al1-v1`, `notebook-al2-v1`, or `notebook-al2-v2`, depending on which version of Amazon Linux you require.
*
*/
public Output platformIdentifier() {
return this.platformIdentifier;
}
/**
* The ARN of the IAM role to be used by the notebook instance which allows SageMaker to call other services on your behalf.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output roleArn;
/**
* @return The ARN of the IAM role to be used by the notebook instance which allows SageMaker to call other services on your behalf.
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* Whether root access is `Enabled` or `Disabled` for users of the notebook instance. The default value is `Enabled`.
*
*/
@Export(name="rootAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> rootAccess;
/**
* @return Whether root access is `Enabled` or `Disabled` for users of the notebook instance. The default value is `Enabled`.
*
*/
public Output> rootAccess() {
return Codegen.optional(this.rootAccess);
}
/**
* The associated security groups.
*
*/
@Export(name="securityGroups", refs={List.class,String.class}, tree="[0,1]")
private Output> securityGroups;
/**
* @return The associated security groups.
*
*/
public Output> securityGroups() {
return this.securityGroups;
}
/**
* The VPC subnet ID.
*
*/
@Export(name="subnetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> subnetId;
/**
* @return The VPC subnet ID.
*
*/
public Output> subnetId() {
return Codegen.optional(this.subnetId);
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy