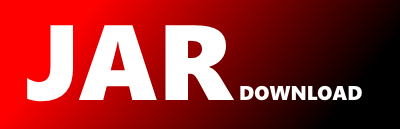
com.pulumi.aws.sagemaker.inputs.DomainDefaultSpaceSettingsJupyterServerAppSettingsDefaultResourceSpecArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DomainDefaultSpaceSettingsJupyterServerAppSettingsDefaultResourceSpecArgs extends com.pulumi.resources.ResourceArgs {
public static final DomainDefaultSpaceSettingsJupyterServerAppSettingsDefaultResourceSpecArgs Empty = new DomainDefaultSpaceSettingsJupyterServerAppSettingsDefaultResourceSpecArgs();
/**
* The instance type that the image version runs on.. For valid values see [SageMaker Instance Types](https://docs.aws.amazon.com/sagemaker/latest/dg/notebooks-available-instance-types.html).
*
*/
@Import(name="instanceType")
private @Nullable Output instanceType;
/**
* @return The instance type that the image version runs on.. For valid values see [SageMaker Instance Types](https://docs.aws.amazon.com/sagemaker/latest/dg/notebooks-available-instance-types.html).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy