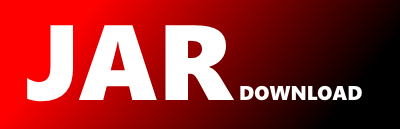
com.pulumi.aws.sagemaker.outputs.ModelContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker.outputs;
import com.pulumi.aws.sagemaker.outputs.ModelContainerImageConfig;
import com.pulumi.aws.sagemaker.outputs.ModelContainerModelDataSource;
import com.pulumi.aws.sagemaker.outputs.ModelContainerMultiModelConfig;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ModelContainer {
/**
* @return The DNS host name for the container.
*
*/
private @Nullable String containerHostname;
/**
* @return Environment variables for the Docker container.
* A list of key value pairs.
*
*/
private @Nullable Map environment;
/**
* @return The registry path where the inference code image is stored in Amazon ECR.
*
*/
private @Nullable String image;
/**
* @return Specifies whether the model container is in Amazon ECR or a private Docker registry accessible from your Amazon Virtual Private Cloud (VPC). For more information see [Using a Private Docker Registry for Real-Time Inference Containers](https://docs.aws.amazon.com/sagemaker/latest/dg/your-algorithms-containers-inference-private.html). see Image Config.
*
*/
private @Nullable ModelContainerImageConfig imageConfig;
/**
* @return The inference specification name in the model package version.
*
*/
private @Nullable String inferenceSpecificationName;
/**
* @return The container hosts value `SingleModel/MultiModel`. The default value is `SingleModel`.
*
*/
private @Nullable String mode;
/**
* @return The location of model data to deploy. Use this for uncompressed model deployment. For information about how to deploy an uncompressed model, see [Deploying uncompressed models](https://docs.aws.amazon.com/sagemaker/latest/dg/large-model-inference-uncompressed.html) in the _AWS SageMaker Developer Guide_.
*
*/
private @Nullable ModelContainerModelDataSource modelDataSource;
/**
* @return The URL for the S3 location where model artifacts are stored.
*
*/
private @Nullable String modelDataUrl;
/**
* @return The Amazon Resource Name (ARN) of the model package to use to create the model.
*
*/
private @Nullable String modelPackageName;
/**
* @return Specifies additional configuration for multi-model endpoints. see Multi Model Config.
*
*/
private @Nullable ModelContainerMultiModelConfig multiModelConfig;
private ModelContainer() {}
/**
* @return The DNS host name for the container.
*
*/
public Optional containerHostname() {
return Optional.ofNullable(this.containerHostname);
}
/**
* @return Environment variables for the Docker container.
* A list of key value pairs.
*
*/
public Map environment() {
return this.environment == null ? Map.of() : this.environment;
}
/**
* @return The registry path where the inference code image is stored in Amazon ECR.
*
*/
public Optional image() {
return Optional.ofNullable(this.image);
}
/**
* @return Specifies whether the model container is in Amazon ECR or a private Docker registry accessible from your Amazon Virtual Private Cloud (VPC). For more information see [Using a Private Docker Registry for Real-Time Inference Containers](https://docs.aws.amazon.com/sagemaker/latest/dg/your-algorithms-containers-inference-private.html). see Image Config.
*
*/
public Optional imageConfig() {
return Optional.ofNullable(this.imageConfig);
}
/**
* @return The inference specification name in the model package version.
*
*/
public Optional inferenceSpecificationName() {
return Optional.ofNullable(this.inferenceSpecificationName);
}
/**
* @return The container hosts value `SingleModel/MultiModel`. The default value is `SingleModel`.
*
*/
public Optional mode() {
return Optional.ofNullable(this.mode);
}
/**
* @return The location of model data to deploy. Use this for uncompressed model deployment. For information about how to deploy an uncompressed model, see [Deploying uncompressed models](https://docs.aws.amazon.com/sagemaker/latest/dg/large-model-inference-uncompressed.html) in the _AWS SageMaker Developer Guide_.
*
*/
public Optional modelDataSource() {
return Optional.ofNullable(this.modelDataSource);
}
/**
* @return The URL for the S3 location where model artifacts are stored.
*
*/
public Optional modelDataUrl() {
return Optional.ofNullable(this.modelDataUrl);
}
/**
* @return The Amazon Resource Name (ARN) of the model package to use to create the model.
*
*/
public Optional modelPackageName() {
return Optional.ofNullable(this.modelPackageName);
}
/**
* @return Specifies additional configuration for multi-model endpoints. see Multi Model Config.
*
*/
public Optional multiModelConfig() {
return Optional.ofNullable(this.multiModelConfig);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ModelContainer defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String containerHostname;
private @Nullable Map environment;
private @Nullable String image;
private @Nullable ModelContainerImageConfig imageConfig;
private @Nullable String inferenceSpecificationName;
private @Nullable String mode;
private @Nullable ModelContainerModelDataSource modelDataSource;
private @Nullable String modelDataUrl;
private @Nullable String modelPackageName;
private @Nullable ModelContainerMultiModelConfig multiModelConfig;
public Builder() {}
public Builder(ModelContainer defaults) {
Objects.requireNonNull(defaults);
this.containerHostname = defaults.containerHostname;
this.environment = defaults.environment;
this.image = defaults.image;
this.imageConfig = defaults.imageConfig;
this.inferenceSpecificationName = defaults.inferenceSpecificationName;
this.mode = defaults.mode;
this.modelDataSource = defaults.modelDataSource;
this.modelDataUrl = defaults.modelDataUrl;
this.modelPackageName = defaults.modelPackageName;
this.multiModelConfig = defaults.multiModelConfig;
}
@CustomType.Setter
public Builder containerHostname(@Nullable String containerHostname) {
this.containerHostname = containerHostname;
return this;
}
@CustomType.Setter
public Builder environment(@Nullable Map environment) {
this.environment = environment;
return this;
}
@CustomType.Setter
public Builder image(@Nullable String image) {
this.image = image;
return this;
}
@CustomType.Setter
public Builder imageConfig(@Nullable ModelContainerImageConfig imageConfig) {
this.imageConfig = imageConfig;
return this;
}
@CustomType.Setter
public Builder inferenceSpecificationName(@Nullable String inferenceSpecificationName) {
this.inferenceSpecificationName = inferenceSpecificationName;
return this;
}
@CustomType.Setter
public Builder mode(@Nullable String mode) {
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder modelDataSource(@Nullable ModelContainerModelDataSource modelDataSource) {
this.modelDataSource = modelDataSource;
return this;
}
@CustomType.Setter
public Builder modelDataUrl(@Nullable String modelDataUrl) {
this.modelDataUrl = modelDataUrl;
return this;
}
@CustomType.Setter
public Builder modelPackageName(@Nullable String modelPackageName) {
this.modelPackageName = modelPackageName;
return this;
}
@CustomType.Setter
public Builder multiModelConfig(@Nullable ModelContainerMultiModelConfig multiModelConfig) {
this.multiModelConfig = multiModelConfig;
return this;
}
public ModelContainer build() {
final var _resultValue = new ModelContainer();
_resultValue.containerHostname = containerHostname;
_resultValue.environment = environment;
_resultValue.image = image;
_resultValue.imageConfig = imageConfig;
_resultValue.inferenceSpecificationName = inferenceSpecificationName;
_resultValue.mode = mode;
_resultValue.modelDataSource = modelDataSource;
_resultValue.modelDataUrl = modelDataUrl;
_resultValue.modelPackageName = modelPackageName;
_resultValue.multiModelConfig = multiModelConfig;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy