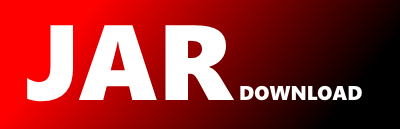
com.pulumi.aws.securityhub.StandardsControl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.securityhub;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.securityhub.StandardsControlArgs;
import com.pulumi.aws.securityhub.inputs.StandardsControlState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* Disable/enable Security Hub standards control in the current region.
*
* The `aws.securityhub.StandardsControl` behaves differently from normal resources, in that
* Pulumi does not _create_ this resource, but instead "adopts" it
* into management. When you _delete_ this resource configuration, Pulumi "abandons" resource as is and just removes it from the state.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.securityhub.Account;
* import com.pulumi.aws.securityhub.StandardsSubscription;
* import com.pulumi.aws.securityhub.StandardsSubscriptionArgs;
* import com.pulumi.aws.securityhub.StandardsControl;
* import com.pulumi.aws.securityhub.StandardsControlArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Account("example");
*
* var cisAwsFoundationsBenchmark = new StandardsSubscription("cisAwsFoundationsBenchmark", StandardsSubscriptionArgs.builder()
* .standardsArn("arn:aws:securityhub:::ruleset/cis-aws-foundations-benchmark/v/1.2.0")
* .build(), CustomResourceOptions.builder()
* .dependsOn(example)
* .build());
*
* var ensureIamPasswordPolicyPreventsPasswordReuse = new StandardsControl("ensureIamPasswordPolicyPreventsPasswordReuse", StandardsControlArgs.builder()
* .standardsControlArn("arn:aws:securityhub:us-east-1:111111111111:control/cis-aws-foundations-benchmark/v/1.2.0/1.10")
* .controlStatus("DISABLED")
* .disabledReason("We handle password policies within Okta")
* .build(), CustomResourceOptions.builder()
* .dependsOn(cisAwsFoundationsBenchmark)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:securityhub/standardsControl:StandardsControl")
public class StandardsControl extends com.pulumi.resources.CustomResource {
/**
* The identifier of the security standard control.
*
*/
@Export(name="controlId", refs={String.class}, tree="[0]")
private Output controlId;
/**
* @return The identifier of the security standard control.
*
*/
public Output controlId() {
return this.controlId;
}
/**
* The control status could be `ENABLED` or `DISABLED`. You have to specify `disabled_reason` argument for `DISABLED` control status.
*
*/
@Export(name="controlStatus", refs={String.class}, tree="[0]")
private Output controlStatus;
/**
* @return The control status could be `ENABLED` or `DISABLED`. You have to specify `disabled_reason` argument for `DISABLED` control status.
*
*/
public Output controlStatus() {
return this.controlStatus;
}
/**
* The date and time that the status of the security standard control was most recently updated.
*
*/
@Export(name="controlStatusUpdatedAt", refs={String.class}, tree="[0]")
private Output controlStatusUpdatedAt;
/**
* @return The date and time that the status of the security standard control was most recently updated.
*
*/
public Output controlStatusUpdatedAt() {
return this.controlStatusUpdatedAt;
}
/**
* The standard control longer description. Provides information about what the control is checking for.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return The standard control longer description. Provides information about what the control is checking for.
*
*/
public Output description() {
return this.description;
}
/**
* A description of the reason why you are disabling a security standard control. If you specify this attribute, `control_status` will be set to `DISABLED` automatically.
*
*/
@Export(name="disabledReason", refs={String.class}, tree="[0]")
private Output disabledReason;
/**
* @return A description of the reason why you are disabling a security standard control. If you specify this attribute, `control_status` will be set to `DISABLED` automatically.
*
*/
public Output disabledReason() {
return this.disabledReason;
}
/**
* The list of requirements that are related to this control.
*
*/
@Export(name="relatedRequirements", refs={List.class,String.class}, tree="[0,1]")
private Output> relatedRequirements;
/**
* @return The list of requirements that are related to this control.
*
*/
public Output> relatedRequirements() {
return this.relatedRequirements;
}
/**
* A link to remediation information for the control in the Security Hub user documentation.
*
*/
@Export(name="remediationUrl", refs={String.class}, tree="[0]")
private Output remediationUrl;
/**
* @return A link to remediation information for the control in the Security Hub user documentation.
*
*/
public Output remediationUrl() {
return this.remediationUrl;
}
/**
* The severity of findings generated from this security standard control.
*
*/
@Export(name="severityRating", refs={String.class}, tree="[0]")
private Output severityRating;
/**
* @return The severity of findings generated from this security standard control.
*
*/
public Output severityRating() {
return this.severityRating;
}
/**
* The standards control ARN. See the AWS documentation for how to list existing controls using [`get-enabled-standards`](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/securityhub/get-enabled-standards.html) and [`describe-standards-controls`](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/securityhub/describe-standards-controls.html).
*
*/
@Export(name="standardsControlArn", refs={String.class}, tree="[0]")
private Output standardsControlArn;
/**
* @return The standards control ARN. See the AWS documentation for how to list existing controls using [`get-enabled-standards`](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/securityhub/get-enabled-standards.html) and [`describe-standards-controls`](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/securityhub/describe-standards-controls.html).
*
*/
public Output standardsControlArn() {
return this.standardsControlArn;
}
/**
* The standard control title.
*
*/
@Export(name="title", refs={String.class}, tree="[0]")
private Output title;
/**
* @return The standard control title.
*
*/
public Output title() {
return this.title;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public StandardsControl(java.lang.String name) {
this(name, StandardsControlArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public StandardsControl(java.lang.String name, StandardsControlArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public StandardsControl(java.lang.String name, StandardsControlArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:securityhub/standardsControl:StandardsControl", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private StandardsControl(java.lang.String name, Output id, @Nullable StandardsControlState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:securityhub/standardsControl:StandardsControl", name, state, makeResourceOptions(options, id), false);
}
private static StandardsControlArgs makeArgs(StandardsControlArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? StandardsControlArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static StandardsControl get(java.lang.String name, Output id, @Nullable StandardsControlState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new StandardsControl(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy