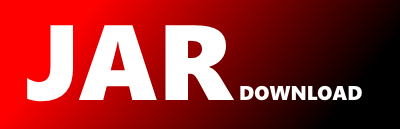
com.pulumi.aws.ssm.outputs.ContactsRotationRecurrence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ssm.outputs;
import com.pulumi.aws.ssm.outputs.ContactsRotationRecurrenceDailySetting;
import com.pulumi.aws.ssm.outputs.ContactsRotationRecurrenceMonthlySetting;
import com.pulumi.aws.ssm.outputs.ContactsRotationRecurrenceShiftCoverage;
import com.pulumi.aws.ssm.outputs.ContactsRotationRecurrenceWeeklySetting;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class ContactsRotationRecurrence {
private @Nullable List dailySettings;
/**
* @return (Optional) Information about on-call rotations that recur monthly. See Monthly Settings for more details.
*
*/
private @Nullable List monthlySettings;
/**
* @return (Required) The number of contacts, or shift team members designated to be on call concurrently during a shift.
*
*/
private Integer numberOfOnCalls;
/**
* @return (Required) The number of days, weeks, or months a single rotation lasts.
*
*/
private Integer recurrenceMultiplier;
/**
* @return (Optional) Information about the days of the week that the on-call rotation coverage includes. See Shift Coverages for more details.
*
*/
private @Nullable List shiftCoverages;
/**
* @return (Optional) Information about on-call rotations that recur weekly. See Weekly Settings for more details.
*
*/
private @Nullable List weeklySettings;
private ContactsRotationRecurrence() {}
public List dailySettings() {
return this.dailySettings == null ? List.of() : this.dailySettings;
}
/**
* @return (Optional) Information about on-call rotations that recur monthly. See Monthly Settings for more details.
*
*/
public List monthlySettings() {
return this.monthlySettings == null ? List.of() : this.monthlySettings;
}
/**
* @return (Required) The number of contacts, or shift team members designated to be on call concurrently during a shift.
*
*/
public Integer numberOfOnCalls() {
return this.numberOfOnCalls;
}
/**
* @return (Required) The number of days, weeks, or months a single rotation lasts.
*
*/
public Integer recurrenceMultiplier() {
return this.recurrenceMultiplier;
}
/**
* @return (Optional) Information about the days of the week that the on-call rotation coverage includes. See Shift Coverages for more details.
*
*/
public List shiftCoverages() {
return this.shiftCoverages == null ? List.of() : this.shiftCoverages;
}
/**
* @return (Optional) Information about on-call rotations that recur weekly. See Weekly Settings for more details.
*
*/
public List weeklySettings() {
return this.weeklySettings == null ? List.of() : this.weeklySettings;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ContactsRotationRecurrence defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List dailySettings;
private @Nullable List monthlySettings;
private Integer numberOfOnCalls;
private Integer recurrenceMultiplier;
private @Nullable List shiftCoverages;
private @Nullable List weeklySettings;
public Builder() {}
public Builder(ContactsRotationRecurrence defaults) {
Objects.requireNonNull(defaults);
this.dailySettings = defaults.dailySettings;
this.monthlySettings = defaults.monthlySettings;
this.numberOfOnCalls = defaults.numberOfOnCalls;
this.recurrenceMultiplier = defaults.recurrenceMultiplier;
this.shiftCoverages = defaults.shiftCoverages;
this.weeklySettings = defaults.weeklySettings;
}
@CustomType.Setter
public Builder dailySettings(@Nullable List dailySettings) {
this.dailySettings = dailySettings;
return this;
}
public Builder dailySettings(ContactsRotationRecurrenceDailySetting... dailySettings) {
return dailySettings(List.of(dailySettings));
}
@CustomType.Setter
public Builder monthlySettings(@Nullable List monthlySettings) {
this.monthlySettings = monthlySettings;
return this;
}
public Builder monthlySettings(ContactsRotationRecurrenceMonthlySetting... monthlySettings) {
return monthlySettings(List.of(monthlySettings));
}
@CustomType.Setter
public Builder numberOfOnCalls(Integer numberOfOnCalls) {
if (numberOfOnCalls == null) {
throw new MissingRequiredPropertyException("ContactsRotationRecurrence", "numberOfOnCalls");
}
this.numberOfOnCalls = numberOfOnCalls;
return this;
}
@CustomType.Setter
public Builder recurrenceMultiplier(Integer recurrenceMultiplier) {
if (recurrenceMultiplier == null) {
throw new MissingRequiredPropertyException("ContactsRotationRecurrence", "recurrenceMultiplier");
}
this.recurrenceMultiplier = recurrenceMultiplier;
return this;
}
@CustomType.Setter
public Builder shiftCoverages(@Nullable List shiftCoverages) {
this.shiftCoverages = shiftCoverages;
return this;
}
public Builder shiftCoverages(ContactsRotationRecurrenceShiftCoverage... shiftCoverages) {
return shiftCoverages(List.of(shiftCoverages));
}
@CustomType.Setter
public Builder weeklySettings(@Nullable List weeklySettings) {
this.weeklySettings = weeklySettings;
return this;
}
public Builder weeklySettings(ContactsRotationRecurrenceWeeklySetting... weeklySettings) {
return weeklySettings(List.of(weeklySettings));
}
public ContactsRotationRecurrence build() {
final var _resultValue = new ContactsRotationRecurrence();
_resultValue.dailySettings = dailySettings;
_resultValue.monthlySettings = monthlySettings;
_resultValue.numberOfOnCalls = numberOfOnCalls;
_resultValue.recurrenceMultiplier = recurrenceMultiplier;
_resultValue.shiftCoverages = shiftCoverages;
_resultValue.weeklySettings = weeklySettings;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy