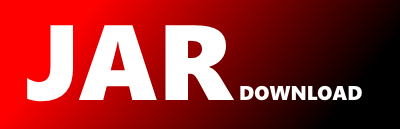
com.pulumi.aws.transfer.Profile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transfer;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.transfer.ProfileArgs;
import com.pulumi.aws.transfer.inputs.ProfileState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a AWS Transfer AS2 Profile resource.
*
* ## Example Usage
*
* ### Basic
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Transfer AS2 Profile using the `profile_id`. For example:
*
* ```sh
* $ pulumi import aws:transfer/profile:Profile example p-4221a88afd5f4362a
* ```
*
*/
@ResourceType(type="aws:transfer/profile:Profile")
public class Profile extends com.pulumi.resources.CustomResource {
/**
* The ARN of the profile.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the profile.
*
*/
public Output arn() {
return this.arn;
}
/**
* The As2Id is the AS2 name as defined in the RFC 4130. For inbound ttransfers this is the AS2 From Header for the AS2 messages sent from the partner. For Outbound messages this is the AS2 To Header for the AS2 messages sent to the partner. his ID cannot include spaces.
*
*/
@Export(name="as2Id", refs={String.class}, tree="[0]")
private Output as2Id;
/**
* @return The As2Id is the AS2 name as defined in the RFC 4130. For inbound ttransfers this is the AS2 From Header for the AS2 messages sent from the partner. For Outbound messages this is the AS2 To Header for the AS2 messages sent to the partner. his ID cannot include spaces.
*
*/
public Output as2Id() {
return this.as2Id;
}
/**
* The list of certificate Ids from the imported certificate operation.
*
*/
@Export(name="certificateIds", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> certificateIds;
/**
* @return The list of certificate Ids from the imported certificate operation.
*
*/
public Output>> certificateIds() {
return Codegen.optional(this.certificateIds);
}
/**
* The unique identifier for the AS2 profile.
*
*/
@Export(name="profileId", refs={String.class}, tree="[0]")
private Output profileId;
/**
* @return The unique identifier for the AS2 profile.
*
*/
public Output profileId() {
return this.profileId;
}
/**
* The profile type should be LOCAL or PARTNER.
*
*/
@Export(name="profileType", refs={String.class}, tree="[0]")
private Output profileType;
/**
* @return The profile type should be LOCAL or PARTNER.
*
*/
public Output profileType() {
return this.profileType;
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy