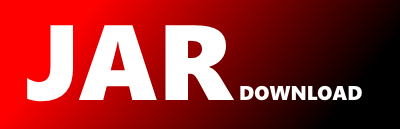
com.pulumi.aws.transfer.outputs.GetConnectorAs2Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transfer.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetConnectorAs2Config {
/**
* @return Basic authentication for AS2 connector API. Returns a null value if not set.
*
*/
private String basicAuthSecretId;
/**
* @return Specifies whether AS2 file is compressed. Will be ZLIB or DISABLED
*
*/
private String compression;
/**
* @return Algorithm used to encrypt file. Will be AES128_CBC or AES192_CBC or AES256_CBC or DES_EDE3_CBC or NONE.
*
*/
private String encryptionAlgorithm;
/**
* @return Unique identifier for AS2 local profile.
*
*/
private String localProfileId;
/**
* @return Used for outbound requests to tell if response is asynchronous or not. Will be either SYNC or NONE.
*
*/
private String mdnResponse;
/**
* @return Signing algorithm for MDN response. Will be SHA256 or SHA384 or SHA512 or SHA1 or NONE or DEFAULT.
*
*/
private String mdnSigningAlgorithm;
/**
* @return Subject HTTP header attribute in outbound AS2 messages to the connector.
*
*/
private String messageSubject;
/**
* @return Unique identifier used by connector for partner profile.
*
*/
private String partnerProfileId;
private String singingAlgorithm;
private GetConnectorAs2Config() {}
/**
* @return Basic authentication for AS2 connector API. Returns a null value if not set.
*
*/
public String basicAuthSecretId() {
return this.basicAuthSecretId;
}
/**
* @return Specifies whether AS2 file is compressed. Will be ZLIB or DISABLED
*
*/
public String compression() {
return this.compression;
}
/**
* @return Algorithm used to encrypt file. Will be AES128_CBC or AES192_CBC or AES256_CBC or DES_EDE3_CBC or NONE.
*
*/
public String encryptionAlgorithm() {
return this.encryptionAlgorithm;
}
/**
* @return Unique identifier for AS2 local profile.
*
*/
public String localProfileId() {
return this.localProfileId;
}
/**
* @return Used for outbound requests to tell if response is asynchronous or not. Will be either SYNC or NONE.
*
*/
public String mdnResponse() {
return this.mdnResponse;
}
/**
* @return Signing algorithm for MDN response. Will be SHA256 or SHA384 or SHA512 or SHA1 or NONE or DEFAULT.
*
*/
public String mdnSigningAlgorithm() {
return this.mdnSigningAlgorithm;
}
/**
* @return Subject HTTP header attribute in outbound AS2 messages to the connector.
*
*/
public String messageSubject() {
return this.messageSubject;
}
/**
* @return Unique identifier used by connector for partner profile.
*
*/
public String partnerProfileId() {
return this.partnerProfileId;
}
public String singingAlgorithm() {
return this.singingAlgorithm;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetConnectorAs2Config defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String basicAuthSecretId;
private String compression;
private String encryptionAlgorithm;
private String localProfileId;
private String mdnResponse;
private String mdnSigningAlgorithm;
private String messageSubject;
private String partnerProfileId;
private String singingAlgorithm;
public Builder() {}
public Builder(GetConnectorAs2Config defaults) {
Objects.requireNonNull(defaults);
this.basicAuthSecretId = defaults.basicAuthSecretId;
this.compression = defaults.compression;
this.encryptionAlgorithm = defaults.encryptionAlgorithm;
this.localProfileId = defaults.localProfileId;
this.mdnResponse = defaults.mdnResponse;
this.mdnSigningAlgorithm = defaults.mdnSigningAlgorithm;
this.messageSubject = defaults.messageSubject;
this.partnerProfileId = defaults.partnerProfileId;
this.singingAlgorithm = defaults.singingAlgorithm;
}
@CustomType.Setter
public Builder basicAuthSecretId(String basicAuthSecretId) {
if (basicAuthSecretId == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "basicAuthSecretId");
}
this.basicAuthSecretId = basicAuthSecretId;
return this;
}
@CustomType.Setter
public Builder compression(String compression) {
if (compression == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "compression");
}
this.compression = compression;
return this;
}
@CustomType.Setter
public Builder encryptionAlgorithm(String encryptionAlgorithm) {
if (encryptionAlgorithm == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "encryptionAlgorithm");
}
this.encryptionAlgorithm = encryptionAlgorithm;
return this;
}
@CustomType.Setter
public Builder localProfileId(String localProfileId) {
if (localProfileId == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "localProfileId");
}
this.localProfileId = localProfileId;
return this;
}
@CustomType.Setter
public Builder mdnResponse(String mdnResponse) {
if (mdnResponse == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "mdnResponse");
}
this.mdnResponse = mdnResponse;
return this;
}
@CustomType.Setter
public Builder mdnSigningAlgorithm(String mdnSigningAlgorithm) {
if (mdnSigningAlgorithm == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "mdnSigningAlgorithm");
}
this.mdnSigningAlgorithm = mdnSigningAlgorithm;
return this;
}
@CustomType.Setter
public Builder messageSubject(String messageSubject) {
if (messageSubject == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "messageSubject");
}
this.messageSubject = messageSubject;
return this;
}
@CustomType.Setter
public Builder partnerProfileId(String partnerProfileId) {
if (partnerProfileId == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "partnerProfileId");
}
this.partnerProfileId = partnerProfileId;
return this;
}
@CustomType.Setter
public Builder singingAlgorithm(String singingAlgorithm) {
if (singingAlgorithm == null) {
throw new MissingRequiredPropertyException("GetConnectorAs2Config", "singingAlgorithm");
}
this.singingAlgorithm = singingAlgorithm;
return this;
}
public GetConnectorAs2Config build() {
final var _resultValue = new GetConnectorAs2Config();
_resultValue.basicAuthSecretId = basicAuthSecretId;
_resultValue.compression = compression;
_resultValue.encryptionAlgorithm = encryptionAlgorithm;
_resultValue.localProfileId = localProfileId;
_resultValue.mdnResponse = mdnResponse;
_resultValue.mdnSigningAlgorithm = mdnSigningAlgorithm;
_resultValue.messageSubject = messageSubject;
_resultValue.partnerProfileId = partnerProfileId;
_resultValue.singingAlgorithm = singingAlgorithm;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy