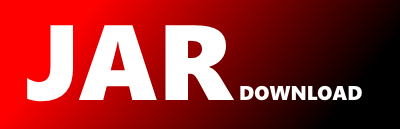
com.pulumi.aws.transfer.outputs.ServerEndpointDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transfer.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ServerEndpointDetails {
/**
* @return A list of address allocation IDs that are required to attach an Elastic IP address to your SFTP server's endpoint. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
private @Nullable List addressAllocationIds;
/**
* @return A list of security groups IDs that are available to attach to your server's endpoint. If no security groups are specified, the VPC's default security groups are automatically assigned to your endpoint. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
private @Nullable List securityGroupIds;
/**
* @return A list of subnet IDs that are required to host your SFTP server endpoint in your VPC. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
private @Nullable List subnetIds;
/**
* @return The ID of the VPC endpoint. This property can only be used when `endpoint_type` is set to `VPC_ENDPOINT`
*
*/
private @Nullable String vpcEndpointId;
/**
* @return The VPC ID of the virtual private cloud in which the SFTP server's endpoint will be hosted. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
private @Nullable String vpcId;
private ServerEndpointDetails() {}
/**
* @return A list of address allocation IDs that are required to attach an Elastic IP address to your SFTP server's endpoint. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
public List addressAllocationIds() {
return this.addressAllocationIds == null ? List.of() : this.addressAllocationIds;
}
/**
* @return A list of security groups IDs that are available to attach to your server's endpoint. If no security groups are specified, the VPC's default security groups are automatically assigned to your endpoint. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
public List securityGroupIds() {
return this.securityGroupIds == null ? List.of() : this.securityGroupIds;
}
/**
* @return A list of subnet IDs that are required to host your SFTP server endpoint in your VPC. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
public List subnetIds() {
return this.subnetIds == null ? List.of() : this.subnetIds;
}
/**
* @return The ID of the VPC endpoint. This property can only be used when `endpoint_type` is set to `VPC_ENDPOINT`
*
*/
public Optional vpcEndpointId() {
return Optional.ofNullable(this.vpcEndpointId);
}
/**
* @return The VPC ID of the virtual private cloud in which the SFTP server's endpoint will be hosted. This property can only be used when `endpoint_type` is set to `VPC`.
*
*/
public Optional vpcId() {
return Optional.ofNullable(this.vpcId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ServerEndpointDetails defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List addressAllocationIds;
private @Nullable List securityGroupIds;
private @Nullable List subnetIds;
private @Nullable String vpcEndpointId;
private @Nullable String vpcId;
public Builder() {}
public Builder(ServerEndpointDetails defaults) {
Objects.requireNonNull(defaults);
this.addressAllocationIds = defaults.addressAllocationIds;
this.securityGroupIds = defaults.securityGroupIds;
this.subnetIds = defaults.subnetIds;
this.vpcEndpointId = defaults.vpcEndpointId;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder addressAllocationIds(@Nullable List addressAllocationIds) {
this.addressAllocationIds = addressAllocationIds;
return this;
}
public Builder addressAllocationIds(String... addressAllocationIds) {
return addressAllocationIds(List.of(addressAllocationIds));
}
@CustomType.Setter
public Builder securityGroupIds(@Nullable List securityGroupIds) {
this.securityGroupIds = securityGroupIds;
return this;
}
public Builder securityGroupIds(String... securityGroupIds) {
return securityGroupIds(List.of(securityGroupIds));
}
@CustomType.Setter
public Builder subnetIds(@Nullable List subnetIds) {
this.subnetIds = subnetIds;
return this;
}
public Builder subnetIds(String... subnetIds) {
return subnetIds(List.of(subnetIds));
}
@CustomType.Setter
public Builder vpcEndpointId(@Nullable String vpcEndpointId) {
this.vpcEndpointId = vpcEndpointId;
return this;
}
@CustomType.Setter
public Builder vpcId(@Nullable String vpcId) {
this.vpcId = vpcId;
return this;
}
public ServerEndpointDetails build() {
final var _resultValue = new ServerEndpointDetails();
_resultValue.addressAllocationIds = addressAllocationIds;
_resultValue.securityGroupIds = securityGroupIds;
_resultValue.subnetIds = subnetIds;
_resultValue.vpcEndpointId = vpcEndpointId;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy