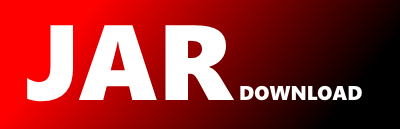
com.pulumi.aws.vpclattice.outputs.GetServiceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.vpclattice.outputs;
import com.pulumi.aws.vpclattice.outputs.GetServiceDnsEntry;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetServiceResult {
/**
* @return ARN of the service.
*
*/
private String arn;
/**
* @return Type of IAM policy. Either `NONE` or `AWS_IAM`.
*
*/
private String authType;
/**
* @return Amazon Resource Name (ARN) of the certificate.
*
*/
private String certificateArn;
/**
* @return Custom domain name of the service.
*
*/
private String customDomainName;
/**
* @return DNS name of the service.
*
*/
private List dnsEntries;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String name;
private String serviceIdentifier;
/**
* @return Status of the service.
*
*/
private String status;
/**
* @return List of tags associated with the service.
*
*/
private Map tags;
private GetServiceResult() {}
/**
* @return ARN of the service.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Type of IAM policy. Either `NONE` or `AWS_IAM`.
*
*/
public String authType() {
return this.authType;
}
/**
* @return Amazon Resource Name (ARN) of the certificate.
*
*/
public String certificateArn() {
return this.certificateArn;
}
/**
* @return Custom domain name of the service.
*
*/
public String customDomainName() {
return this.customDomainName;
}
/**
* @return DNS name of the service.
*
*/
public List dnsEntries() {
return this.dnsEntries;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String name() {
return this.name;
}
public String serviceIdentifier() {
return this.serviceIdentifier;
}
/**
* @return Status of the service.
*
*/
public String status() {
return this.status;
}
/**
* @return List of tags associated with the service.
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServiceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String authType;
private String certificateArn;
private String customDomainName;
private List dnsEntries;
private String id;
private String name;
private String serviceIdentifier;
private String status;
private Map tags;
public Builder() {}
public Builder(GetServiceResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.authType = defaults.authType;
this.certificateArn = defaults.certificateArn;
this.customDomainName = defaults.customDomainName;
this.dnsEntries = defaults.dnsEntries;
this.id = defaults.id;
this.name = defaults.name;
this.serviceIdentifier = defaults.serviceIdentifier;
this.status = defaults.status;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder authType(String authType) {
if (authType == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "authType");
}
this.authType = authType;
return this;
}
@CustomType.Setter
public Builder certificateArn(String certificateArn) {
if (certificateArn == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "certificateArn");
}
this.certificateArn = certificateArn;
return this;
}
@CustomType.Setter
public Builder customDomainName(String customDomainName) {
if (customDomainName == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "customDomainName");
}
this.customDomainName = customDomainName;
return this;
}
@CustomType.Setter
public Builder dnsEntries(List dnsEntries) {
if (dnsEntries == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "dnsEntries");
}
this.dnsEntries = dnsEntries;
return this;
}
public Builder dnsEntries(GetServiceDnsEntry... dnsEntries) {
return dnsEntries(List.of(dnsEntries));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder serviceIdentifier(String serviceIdentifier) {
if (serviceIdentifier == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "serviceIdentifier");
}
this.serviceIdentifier = serviceIdentifier;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetServiceResult", "tags");
}
this.tags = tags;
return this;
}
public GetServiceResult build() {
final var _resultValue = new GetServiceResult();
_resultValue.arn = arn;
_resultValue.authType = authType;
_resultValue.certificateArn = certificateArn;
_resultValue.customDomainName = customDomainName;
_resultValue.dnsEntries = dnsEntries;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.serviceIdentifier = serviceIdentifier;
_resultValue.status = status;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy