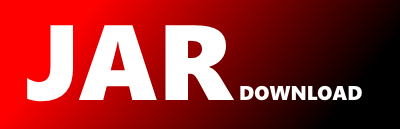
com.pulumi.aws.cloudfront.outputs.DistributionViewerCertificate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudfront.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DistributionViewerCertificate {
/**
* @return ARN of the [AWS Certificate Manager](https://aws.amazon.com/certificate-manager/) certificate that you wish to use with this distribution. Specify this, `cloudfront_default_certificate`, or `iam_certificate_id`. The ACM certificate must be in US-EAST-1.
*
*/
private @Nullable String acmCertificateArn;
/**
* @return `true` if you want viewers to use HTTPS to request your objects and you're using the CloudFront domain name for your distribution. Specify this, `acm_certificate_arn`, or `iam_certificate_id`.
*
*/
private @Nullable Boolean cloudfrontDefaultCertificate;
/**
* @return IAM certificate identifier of the custom viewer certificate for this distribution if you are using a custom domain. Specify this, `acm_certificate_arn`, or `cloudfront_default_certificate`.
*
*/
private @Nullable String iamCertificateId;
/**
* @return Minimum version of the SSL protocol that you want CloudFront to use for HTTPS connections. Can only be set if `cloudfront_default_certificate = false`. See all possible values in [this](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html) table under "Security policy." Some examples include: `TLSv1.2_2019` and `TLSv1.2_2021`. Default: `TLSv1`. **NOTE**: If you are using a custom certificate (specified with `acm_certificate_arn` or `iam_certificate_id`), and have specified `sni-only` in `ssl_support_method`, `TLSv1` or later must be specified. If you have specified `vip` in `ssl_support_method`, only `SSLv3` or `TLSv1` can be specified. If you have specified `cloudfront_default_certificate`, `TLSv1` must be specified.
*
*/
private @Nullable String minimumProtocolVersion;
/**
* @return How you want CloudFront to serve HTTPS requests. One of `vip`, `sni-only`, or `static-ip`. Required if you specify `acm_certificate_arn` or `iam_certificate_id`. **NOTE:** `vip` causes CloudFront to use a dedicated IP address and may incur extra charges.
*
*/
private @Nullable String sslSupportMethod;
private DistributionViewerCertificate() {}
/**
* @return ARN of the [AWS Certificate Manager](https://aws.amazon.com/certificate-manager/) certificate that you wish to use with this distribution. Specify this, `cloudfront_default_certificate`, or `iam_certificate_id`. The ACM certificate must be in US-EAST-1.
*
*/
public Optional acmCertificateArn() {
return Optional.ofNullable(this.acmCertificateArn);
}
/**
* @return `true` if you want viewers to use HTTPS to request your objects and you're using the CloudFront domain name for your distribution. Specify this, `acm_certificate_arn`, or `iam_certificate_id`.
*
*/
public Optional cloudfrontDefaultCertificate() {
return Optional.ofNullable(this.cloudfrontDefaultCertificate);
}
/**
* @return IAM certificate identifier of the custom viewer certificate for this distribution if you are using a custom domain. Specify this, `acm_certificate_arn`, or `cloudfront_default_certificate`.
*
*/
public Optional iamCertificateId() {
return Optional.ofNullable(this.iamCertificateId);
}
/**
* @return Minimum version of the SSL protocol that you want CloudFront to use for HTTPS connections. Can only be set if `cloudfront_default_certificate = false`. See all possible values in [this](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html) table under "Security policy." Some examples include: `TLSv1.2_2019` and `TLSv1.2_2021`. Default: `TLSv1`. **NOTE**: If you are using a custom certificate (specified with `acm_certificate_arn` or `iam_certificate_id`), and have specified `sni-only` in `ssl_support_method`, `TLSv1` or later must be specified. If you have specified `vip` in `ssl_support_method`, only `SSLv3` or `TLSv1` can be specified. If you have specified `cloudfront_default_certificate`, `TLSv1` must be specified.
*
*/
public Optional minimumProtocolVersion() {
return Optional.ofNullable(this.minimumProtocolVersion);
}
/**
* @return How you want CloudFront to serve HTTPS requests. One of `vip`, `sni-only`, or `static-ip`. Required if you specify `acm_certificate_arn` or `iam_certificate_id`. **NOTE:** `vip` causes CloudFront to use a dedicated IP address and may incur extra charges.
*
*/
public Optional sslSupportMethod() {
return Optional.ofNullable(this.sslSupportMethod);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DistributionViewerCertificate defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String acmCertificateArn;
private @Nullable Boolean cloudfrontDefaultCertificate;
private @Nullable String iamCertificateId;
private @Nullable String minimumProtocolVersion;
private @Nullable String sslSupportMethod;
public Builder() {}
public Builder(DistributionViewerCertificate defaults) {
Objects.requireNonNull(defaults);
this.acmCertificateArn = defaults.acmCertificateArn;
this.cloudfrontDefaultCertificate = defaults.cloudfrontDefaultCertificate;
this.iamCertificateId = defaults.iamCertificateId;
this.minimumProtocolVersion = defaults.minimumProtocolVersion;
this.sslSupportMethod = defaults.sslSupportMethod;
}
@CustomType.Setter
public Builder acmCertificateArn(@Nullable String acmCertificateArn) {
this.acmCertificateArn = acmCertificateArn;
return this;
}
@CustomType.Setter
public Builder cloudfrontDefaultCertificate(@Nullable Boolean cloudfrontDefaultCertificate) {
this.cloudfrontDefaultCertificate = cloudfrontDefaultCertificate;
return this;
}
@CustomType.Setter
public Builder iamCertificateId(@Nullable String iamCertificateId) {
this.iamCertificateId = iamCertificateId;
return this;
}
@CustomType.Setter
public Builder minimumProtocolVersion(@Nullable String minimumProtocolVersion) {
this.minimumProtocolVersion = minimumProtocolVersion;
return this;
}
@CustomType.Setter
public Builder sslSupportMethod(@Nullable String sslSupportMethod) {
this.sslSupportMethod = sslSupportMethod;
return this;
}
public DistributionViewerCertificate build() {
final var _resultValue = new DistributionViewerCertificate();
_resultValue.acmCertificateArn = acmCertificateArn;
_resultValue.cloudfrontDefaultCertificate = cloudfrontDefaultCertificate;
_resultValue.iamCertificateId = iamCertificateId;
_resultValue.minimumProtocolVersion = minimumProtocolVersion;
_resultValue.sslSupportMethod = sslSupportMethod;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy