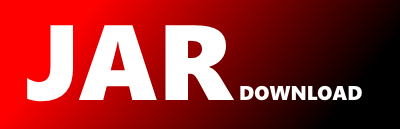
com.pulumi.aws.dynamodb.outputs.GetTableResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dynamodb.outputs;
import com.pulumi.aws.dynamodb.outputs.GetTableAttribute;
import com.pulumi.aws.dynamodb.outputs.GetTableGlobalSecondaryIndex;
import com.pulumi.aws.dynamodb.outputs.GetTableLocalSecondaryIndex;
import com.pulumi.aws.dynamodb.outputs.GetTablePointInTimeRecovery;
import com.pulumi.aws.dynamodb.outputs.GetTableReplica;
import com.pulumi.aws.dynamodb.outputs.GetTableServerSideEncryption;
import com.pulumi.aws.dynamodb.outputs.GetTableTtl;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetTableResult {
private String arn;
private List attributes;
private String billingMode;
private Boolean deletionProtectionEnabled;
private List globalSecondaryIndexes;
private String hashKey;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private List localSecondaryIndexes;
private String name;
private GetTablePointInTimeRecovery pointInTimeRecovery;
private String rangeKey;
private Integer readCapacity;
private List replicas;
private GetTableServerSideEncryption serverSideEncryption;
private String streamArn;
private Boolean streamEnabled;
private String streamLabel;
private String streamViewType;
private String tableClass;
private Map tags;
private GetTableTtl ttl;
private Integer writeCapacity;
private GetTableResult() {}
public String arn() {
return this.arn;
}
public List attributes() {
return this.attributes;
}
public String billingMode() {
return this.billingMode;
}
public Boolean deletionProtectionEnabled() {
return this.deletionProtectionEnabled;
}
public List globalSecondaryIndexes() {
return this.globalSecondaryIndexes;
}
public String hashKey() {
return this.hashKey;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public List localSecondaryIndexes() {
return this.localSecondaryIndexes;
}
public String name() {
return this.name;
}
public GetTablePointInTimeRecovery pointInTimeRecovery() {
return this.pointInTimeRecovery;
}
public String rangeKey() {
return this.rangeKey;
}
public Integer readCapacity() {
return this.readCapacity;
}
public List replicas() {
return this.replicas;
}
public GetTableServerSideEncryption serverSideEncryption() {
return this.serverSideEncryption;
}
public String streamArn() {
return this.streamArn;
}
public Boolean streamEnabled() {
return this.streamEnabled;
}
public String streamLabel() {
return this.streamLabel;
}
public String streamViewType() {
return this.streamViewType;
}
public String tableClass() {
return this.tableClass;
}
public Map tags() {
return this.tags;
}
public GetTableTtl ttl() {
return this.ttl;
}
public Integer writeCapacity() {
return this.writeCapacity;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetTableResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List attributes;
private String billingMode;
private Boolean deletionProtectionEnabled;
private List globalSecondaryIndexes;
private String hashKey;
private String id;
private List localSecondaryIndexes;
private String name;
private GetTablePointInTimeRecovery pointInTimeRecovery;
private String rangeKey;
private Integer readCapacity;
private List replicas;
private GetTableServerSideEncryption serverSideEncryption;
private String streamArn;
private Boolean streamEnabled;
private String streamLabel;
private String streamViewType;
private String tableClass;
private Map tags;
private GetTableTtl ttl;
private Integer writeCapacity;
public Builder() {}
public Builder(GetTableResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.attributes = defaults.attributes;
this.billingMode = defaults.billingMode;
this.deletionProtectionEnabled = defaults.deletionProtectionEnabled;
this.globalSecondaryIndexes = defaults.globalSecondaryIndexes;
this.hashKey = defaults.hashKey;
this.id = defaults.id;
this.localSecondaryIndexes = defaults.localSecondaryIndexes;
this.name = defaults.name;
this.pointInTimeRecovery = defaults.pointInTimeRecovery;
this.rangeKey = defaults.rangeKey;
this.readCapacity = defaults.readCapacity;
this.replicas = defaults.replicas;
this.serverSideEncryption = defaults.serverSideEncryption;
this.streamArn = defaults.streamArn;
this.streamEnabled = defaults.streamEnabled;
this.streamLabel = defaults.streamLabel;
this.streamViewType = defaults.streamViewType;
this.tableClass = defaults.tableClass;
this.tags = defaults.tags;
this.ttl = defaults.ttl;
this.writeCapacity = defaults.writeCapacity;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetTableResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder attributes(List attributes) {
if (attributes == null) {
throw new MissingRequiredPropertyException("GetTableResult", "attributes");
}
this.attributes = attributes;
return this;
}
public Builder attributes(GetTableAttribute... attributes) {
return attributes(List.of(attributes));
}
@CustomType.Setter
public Builder billingMode(String billingMode) {
if (billingMode == null) {
throw new MissingRequiredPropertyException("GetTableResult", "billingMode");
}
this.billingMode = billingMode;
return this;
}
@CustomType.Setter
public Builder deletionProtectionEnabled(Boolean deletionProtectionEnabled) {
if (deletionProtectionEnabled == null) {
throw new MissingRequiredPropertyException("GetTableResult", "deletionProtectionEnabled");
}
this.deletionProtectionEnabled = deletionProtectionEnabled;
return this;
}
@CustomType.Setter
public Builder globalSecondaryIndexes(List globalSecondaryIndexes) {
if (globalSecondaryIndexes == null) {
throw new MissingRequiredPropertyException("GetTableResult", "globalSecondaryIndexes");
}
this.globalSecondaryIndexes = globalSecondaryIndexes;
return this;
}
public Builder globalSecondaryIndexes(GetTableGlobalSecondaryIndex... globalSecondaryIndexes) {
return globalSecondaryIndexes(List.of(globalSecondaryIndexes));
}
@CustomType.Setter
public Builder hashKey(String hashKey) {
if (hashKey == null) {
throw new MissingRequiredPropertyException("GetTableResult", "hashKey");
}
this.hashKey = hashKey;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetTableResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder localSecondaryIndexes(List localSecondaryIndexes) {
if (localSecondaryIndexes == null) {
throw new MissingRequiredPropertyException("GetTableResult", "localSecondaryIndexes");
}
this.localSecondaryIndexes = localSecondaryIndexes;
return this;
}
public Builder localSecondaryIndexes(GetTableLocalSecondaryIndex... localSecondaryIndexes) {
return localSecondaryIndexes(List.of(localSecondaryIndexes));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetTableResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder pointInTimeRecovery(GetTablePointInTimeRecovery pointInTimeRecovery) {
if (pointInTimeRecovery == null) {
throw new MissingRequiredPropertyException("GetTableResult", "pointInTimeRecovery");
}
this.pointInTimeRecovery = pointInTimeRecovery;
return this;
}
@CustomType.Setter
public Builder rangeKey(String rangeKey) {
if (rangeKey == null) {
throw new MissingRequiredPropertyException("GetTableResult", "rangeKey");
}
this.rangeKey = rangeKey;
return this;
}
@CustomType.Setter
public Builder readCapacity(Integer readCapacity) {
if (readCapacity == null) {
throw new MissingRequiredPropertyException("GetTableResult", "readCapacity");
}
this.readCapacity = readCapacity;
return this;
}
@CustomType.Setter
public Builder replicas(List replicas) {
if (replicas == null) {
throw new MissingRequiredPropertyException("GetTableResult", "replicas");
}
this.replicas = replicas;
return this;
}
public Builder replicas(GetTableReplica... replicas) {
return replicas(List.of(replicas));
}
@CustomType.Setter
public Builder serverSideEncryption(GetTableServerSideEncryption serverSideEncryption) {
if (serverSideEncryption == null) {
throw new MissingRequiredPropertyException("GetTableResult", "serverSideEncryption");
}
this.serverSideEncryption = serverSideEncryption;
return this;
}
@CustomType.Setter
public Builder streamArn(String streamArn) {
if (streamArn == null) {
throw new MissingRequiredPropertyException("GetTableResult", "streamArn");
}
this.streamArn = streamArn;
return this;
}
@CustomType.Setter
public Builder streamEnabled(Boolean streamEnabled) {
if (streamEnabled == null) {
throw new MissingRequiredPropertyException("GetTableResult", "streamEnabled");
}
this.streamEnabled = streamEnabled;
return this;
}
@CustomType.Setter
public Builder streamLabel(String streamLabel) {
if (streamLabel == null) {
throw new MissingRequiredPropertyException("GetTableResult", "streamLabel");
}
this.streamLabel = streamLabel;
return this;
}
@CustomType.Setter
public Builder streamViewType(String streamViewType) {
if (streamViewType == null) {
throw new MissingRequiredPropertyException("GetTableResult", "streamViewType");
}
this.streamViewType = streamViewType;
return this;
}
@CustomType.Setter
public Builder tableClass(String tableClass) {
if (tableClass == null) {
throw new MissingRequiredPropertyException("GetTableResult", "tableClass");
}
this.tableClass = tableClass;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetTableResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder ttl(GetTableTtl ttl) {
if (ttl == null) {
throw new MissingRequiredPropertyException("GetTableResult", "ttl");
}
this.ttl = ttl;
return this;
}
@CustomType.Setter
public Builder writeCapacity(Integer writeCapacity) {
if (writeCapacity == null) {
throw new MissingRequiredPropertyException("GetTableResult", "writeCapacity");
}
this.writeCapacity = writeCapacity;
return this;
}
public GetTableResult build() {
final var _resultValue = new GetTableResult();
_resultValue.arn = arn;
_resultValue.attributes = attributes;
_resultValue.billingMode = billingMode;
_resultValue.deletionProtectionEnabled = deletionProtectionEnabled;
_resultValue.globalSecondaryIndexes = globalSecondaryIndexes;
_resultValue.hashKey = hashKey;
_resultValue.id = id;
_resultValue.localSecondaryIndexes = localSecondaryIndexes;
_resultValue.name = name;
_resultValue.pointInTimeRecovery = pointInTimeRecovery;
_resultValue.rangeKey = rangeKey;
_resultValue.readCapacity = readCapacity;
_resultValue.replicas = replicas;
_resultValue.serverSideEncryption = serverSideEncryption;
_resultValue.streamArn = streamArn;
_resultValue.streamEnabled = streamEnabled;
_resultValue.streamLabel = streamLabel;
_resultValue.streamViewType = streamViewType;
_resultValue.tableClass = tableClass;
_resultValue.tags = tags;
_resultValue.ttl = ttl;
_resultValue.writeCapacity = writeCapacity;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy