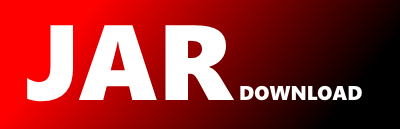
com.pulumi.aws.ec2.outputs.GetInstanceRootBlockDevice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetInstanceRootBlockDevice {
/**
* @return If the root block device will be deleted on termination.
*
*/
private Boolean deleteOnTermination;
/**
* @return Physical name of the device.
*
*/
private String deviceName;
/**
* @return If the EBS volume is encrypted.
*
*/
private Boolean encrypted;
/**
* @return `0` If the volume is not a provisioned IOPS image, otherwise the supported IOPS count.
*
*/
private Integer iops;
private String kmsKeyId;
/**
* @return Map of tags assigned to the Instance.
*
*/
private Map tags;
/**
* @return Throughput of the volume, in MiB/s.
*
*/
private Integer throughput;
private String volumeId;
/**
* @return Size of the volume, in GiB.
*
*/
private Integer volumeSize;
/**
* @return Type of the volume.
*
*/
private String volumeType;
private GetInstanceRootBlockDevice() {}
/**
* @return If the root block device will be deleted on termination.
*
*/
public Boolean deleteOnTermination() {
return this.deleteOnTermination;
}
/**
* @return Physical name of the device.
*
*/
public String deviceName() {
return this.deviceName;
}
/**
* @return If the EBS volume is encrypted.
*
*/
public Boolean encrypted() {
return this.encrypted;
}
/**
* @return `0` If the volume is not a provisioned IOPS image, otherwise the supported IOPS count.
*
*/
public Integer iops() {
return this.iops;
}
public String kmsKeyId() {
return this.kmsKeyId;
}
/**
* @return Map of tags assigned to the Instance.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Throughput of the volume, in MiB/s.
*
*/
public Integer throughput() {
return this.throughput;
}
public String volumeId() {
return this.volumeId;
}
/**
* @return Size of the volume, in GiB.
*
*/
public Integer volumeSize() {
return this.volumeSize;
}
/**
* @return Type of the volume.
*
*/
public String volumeType() {
return this.volumeType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetInstanceRootBlockDevice defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean deleteOnTermination;
private String deviceName;
private Boolean encrypted;
private Integer iops;
private String kmsKeyId;
private Map tags;
private Integer throughput;
private String volumeId;
private Integer volumeSize;
private String volumeType;
public Builder() {}
public Builder(GetInstanceRootBlockDevice defaults) {
Objects.requireNonNull(defaults);
this.deleteOnTermination = defaults.deleteOnTermination;
this.deviceName = defaults.deviceName;
this.encrypted = defaults.encrypted;
this.iops = defaults.iops;
this.kmsKeyId = defaults.kmsKeyId;
this.tags = defaults.tags;
this.throughput = defaults.throughput;
this.volumeId = defaults.volumeId;
this.volumeSize = defaults.volumeSize;
this.volumeType = defaults.volumeType;
}
@CustomType.Setter
public Builder deleteOnTermination(Boolean deleteOnTermination) {
if (deleteOnTermination == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "deleteOnTermination");
}
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder deviceName(String deviceName) {
if (deviceName == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "deviceName");
}
this.deviceName = deviceName;
return this;
}
@CustomType.Setter
public Builder encrypted(Boolean encrypted) {
if (encrypted == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "encrypted");
}
this.encrypted = encrypted;
return this;
}
@CustomType.Setter
public Builder iops(Integer iops) {
if (iops == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "iops");
}
this.iops = iops;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(String kmsKeyId) {
if (kmsKeyId == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "kmsKeyId");
}
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder throughput(Integer throughput) {
if (throughput == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "throughput");
}
this.throughput = throughput;
return this;
}
@CustomType.Setter
public Builder volumeId(String volumeId) {
if (volumeId == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "volumeId");
}
this.volumeId = volumeId;
return this;
}
@CustomType.Setter
public Builder volumeSize(Integer volumeSize) {
if (volumeSize == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "volumeSize");
}
this.volumeSize = volumeSize;
return this;
}
@CustomType.Setter
public Builder volumeType(String volumeType) {
if (volumeType == null) {
throw new MissingRequiredPropertyException("GetInstanceRootBlockDevice", "volumeType");
}
this.volumeType = volumeType;
return this;
}
public GetInstanceRootBlockDevice build() {
final var _resultValue = new GetInstanceRootBlockDevice();
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.deviceName = deviceName;
_resultValue.encrypted = encrypted;
_resultValue.iops = iops;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.tags = tags;
_resultValue.throughput = throughput;
_resultValue.volumeId = volumeId;
_resultValue.volumeSize = volumeSize;
_resultValue.volumeType = volumeType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy