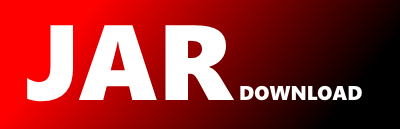
com.pulumi.aws.keyspaces.outputs.TableSchemaDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.keyspaces.outputs;
import com.pulumi.aws.keyspaces.outputs.TableSchemaDefinitionClusteringKey;
import com.pulumi.aws.keyspaces.outputs.TableSchemaDefinitionColumn;
import com.pulumi.aws.keyspaces.outputs.TableSchemaDefinitionPartitionKey;
import com.pulumi.aws.keyspaces.outputs.TableSchemaDefinitionStaticColumn;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class TableSchemaDefinition {
/**
* @return The columns that are part of the clustering key of the table.
*
*/
private @Nullable List clusteringKeys;
/**
* @return The regular columns of the table.
*
*/
private List columns;
/**
* @return The columns that are part of the partition key of the table .
*
*/
private List partitionKeys;
/**
* @return The columns that have been defined as `STATIC`. Static columns store values that are shared by all rows in the same partition.
*
*/
private @Nullable List staticColumns;
private TableSchemaDefinition() {}
/**
* @return The columns that are part of the clustering key of the table.
*
*/
public List clusteringKeys() {
return this.clusteringKeys == null ? List.of() : this.clusteringKeys;
}
/**
* @return The regular columns of the table.
*
*/
public List columns() {
return this.columns;
}
/**
* @return The columns that are part of the partition key of the table .
*
*/
public List partitionKeys() {
return this.partitionKeys;
}
/**
* @return The columns that have been defined as `STATIC`. Static columns store values that are shared by all rows in the same partition.
*
*/
public List staticColumns() {
return this.staticColumns == null ? List.of() : this.staticColumns;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TableSchemaDefinition defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List clusteringKeys;
private List columns;
private List partitionKeys;
private @Nullable List staticColumns;
public Builder() {}
public Builder(TableSchemaDefinition defaults) {
Objects.requireNonNull(defaults);
this.clusteringKeys = defaults.clusteringKeys;
this.columns = defaults.columns;
this.partitionKeys = defaults.partitionKeys;
this.staticColumns = defaults.staticColumns;
}
@CustomType.Setter
public Builder clusteringKeys(@Nullable List clusteringKeys) {
this.clusteringKeys = clusteringKeys;
return this;
}
public Builder clusteringKeys(TableSchemaDefinitionClusteringKey... clusteringKeys) {
return clusteringKeys(List.of(clusteringKeys));
}
@CustomType.Setter
public Builder columns(List columns) {
if (columns == null) {
throw new MissingRequiredPropertyException("TableSchemaDefinition", "columns");
}
this.columns = columns;
return this;
}
public Builder columns(TableSchemaDefinitionColumn... columns) {
return columns(List.of(columns));
}
@CustomType.Setter
public Builder partitionKeys(List partitionKeys) {
if (partitionKeys == null) {
throw new MissingRequiredPropertyException("TableSchemaDefinition", "partitionKeys");
}
this.partitionKeys = partitionKeys;
return this;
}
public Builder partitionKeys(TableSchemaDefinitionPartitionKey... partitionKeys) {
return partitionKeys(List.of(partitionKeys));
}
@CustomType.Setter
public Builder staticColumns(@Nullable List staticColumns) {
this.staticColumns = staticColumns;
return this;
}
public Builder staticColumns(TableSchemaDefinitionStaticColumn... staticColumns) {
return staticColumns(List.of(staticColumns));
}
public TableSchemaDefinition build() {
final var _resultValue = new TableSchemaDefinition();
_resultValue.clusteringKeys = clusteringKeys;
_resultValue.columns = columns;
_resultValue.partitionKeys = partitionKeys;
_resultValue.staticColumns = staticColumns;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy