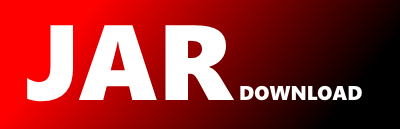
com.pulumi.aws.rds.Instance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.rds.InstanceArgs;
import com.pulumi.aws.rds.inputs.InstanceState;
import com.pulumi.aws.rds.outputs.InstanceBlueGreenUpdate;
import com.pulumi.aws.rds.outputs.InstanceListenerEndpoint;
import com.pulumi.aws.rds.outputs.InstanceMasterUserSecret;
import com.pulumi.aws.rds.outputs.InstanceRestoreToPointInTime;
import com.pulumi.aws.rds.outputs.InstanceS3Import;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an RDS instance resource. A DB instance is an isolated database
* environment in the cloud. A DB instance can contain multiple user-created
* databases.
*
* Changes to a DB instance can occur when you manually change a parameter, such as
* `allocated_storage`, and are reflected in the next maintenance window. Because
* of this, this provider may report a difference in its planning phase because a
* modification has not yet taken place. You can use the `apply_immediately` flag
* to instruct the service to apply the change immediately (see documentation
* below).
*
* When upgrading the major version of an engine, `allow_major_version_upgrade` must be set to `true`.
*
* > **Note:** using `apply_immediately` can result in a brief downtime as the server reboots.
* See the AWS Docs on [RDS Instance Maintenance][instance-maintenance] for more information.
*
* > **Note:** All arguments including the username and password will be stored in the raw state as plain-text.
* Read more about sensitive data instate.
*
* ## RDS Instance Class Types
*
* Amazon RDS supports instance classes for the following use cases: General-purpose, Memory-optimized, Burstable Performance, and Optimized-reads.
* For more information please read the AWS RDS documentation about [DB Instance Class Types](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.DBInstanceClass.html)
*
* ## Low-Downtime Updates
*
* By default, RDS applies updates to DB Instances in-place, which can lead to service interruptions.
* Low-downtime updates minimize service interruptions by performing the updates with an [RDS Blue/Green deployment][blue-green] and switching over the instances when complete.
*
* Low-downtime updates are only available for DB Instances using MySQL, MariaDB and PostgreSQL,
* as other engines are not supported by RDS Blue/Green deployments.
* They cannot be used with DB Instances with replicas.
*
* Backups must be enabled to use low-downtime updates.
*
* Enable low-downtime updates by setting `blue_green_update.enabled` to `true`.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(10)
* .dbName("mydb")
* .engine("mysql")
* .engineVersion("8.0")
* .instanceClass("db.t3.micro")
* .username("foo")
* .password("foobarbaz")
* .parameterGroupName("default.mysql8.0")
* .skipFinalSnapshot(true)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### RDS Custom for Oracle Usage with Replica
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.RdsFunctions;
* import com.pulumi.aws.rds.inputs.GetOrderableDbInstanceArgs;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetKeyArgs;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // Lookup the available instance classes for the custom engine for the region being operated in
* final var custom-oracle = RdsFunctions.getOrderableDbInstance(GetOrderableDbInstanceArgs.builder()
* .engine("custom-oracle-ee")
* .engineVersion("19.c.ee.002")
* .licenseModel("bring-your-own-license")
* .storageType("gp3")
* .preferredInstanceClasses(
* "db.r5.xlarge",
* "db.r5.2xlarge",
* "db.r5.4xlarge")
* .build());
*
* // The RDS instance resource requires an ARN. Look up the ARN of the KMS key associated with the CEV.
* final var byId = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("example-ef278353ceba4a5a97de6784565b9f78")
* .build());
*
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(50)
* .autoMinorVersionUpgrade(false)
* .customIamInstanceProfile("AWSRDSCustomInstanceProfile")
* .backupRetentionPeriod(7)
* .dbSubnetGroupName(dbSubnetGroupName)
* .engine(custom_oracle.engine())
* .engineVersion(custom_oracle.engineVersion())
* .identifier("ee-instance-demo")
* .instanceClass(custom_oracle.instanceClass())
* .kmsKeyId(byId.applyValue(getKeyResult -> getKeyResult.arn()))
* .licenseModel(custom_oracle.licenseModel())
* .multiAz(false)
* .password("avoid-plaintext-passwords")
* .username("test")
* .storageEncrypted(true)
* .build());
*
* var test_replica = new Instance("test-replica", InstanceArgs.builder()
* .replicateSourceDb(default_.identifier())
* .replicaMode("mounted")
* .autoMinorVersionUpgrade(false)
* .customIamInstanceProfile("AWSRDSCustomInstanceProfile")
* .backupRetentionPeriod(7)
* .identifier("ee-instance-replica")
* .instanceClass(custom_oracle.instanceClass())
* .kmsKeyId(byId.applyValue(getKeyResult -> getKeyResult.arn()))
* .multiAz(false)
* .skipFinalSnapshot(true)
* .storageEncrypted(true)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### RDS Custom for SQL Server
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.RdsFunctions;
* import com.pulumi.aws.rds.inputs.GetOrderableDbInstanceArgs;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetKeyArgs;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // Lookup the available instance classes for the custom engine for the region being operated in
* final var custom-sqlserver = RdsFunctions.getOrderableDbInstance(GetOrderableDbInstanceArgs.builder()
* .engine("custom-sqlserver-se")
* .engineVersion("15.00.4249.2.v1")
* .storageType("gp3")
* .preferredInstanceClasses(
* "db.r5.xlarge",
* "db.r5.2xlarge",
* "db.r5.4xlarge")
* .build());
*
* // The RDS instance resource requires an ARN. Look up the ARN of the KMS key.
* final var byId = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("example-ef278353ceba4a5a97de6784565b9f78")
* .build());
*
* var example = new Instance("example", InstanceArgs.builder()
* .allocatedStorage(500)
* .autoMinorVersionUpgrade(false)
* .customIamInstanceProfile("AWSRDSCustomSQLServerInstanceProfile")
* .backupRetentionPeriod(7)
* .dbSubnetGroupName(dbSubnetGroupName)
* .engine(custom_sqlserver.engine())
* .engineVersion(custom_sqlserver.engineVersion())
* .identifier("sql-instance-demo")
* .instanceClass(custom_sqlserver.instanceClass())
* .kmsKeyId(byId.applyValue(getKeyResult -> getKeyResult.arn()))
* .multiAz(false)
* .password("avoid-plaintext-passwords")
* .storageEncrypted(true)
* .username("test")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### RDS Db2 Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.RdsFunctions;
* import com.pulumi.aws.rds.inputs.GetEngineVersionArgs;
* import com.pulumi.aws.rds.inputs.GetOrderableDbInstanceArgs;
* import com.pulumi.aws.rds.ParameterGroup;
* import com.pulumi.aws.rds.ParameterGroupArgs;
* import com.pulumi.aws.rds.inputs.ParameterGroupParameterArgs;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // Lookup the default version for the engine. Db2 Standard Edition is `db2-se`, Db2 Advanced Edition is `db2-ae`.
* final var default = RdsFunctions.getEngineVersion(GetEngineVersionArgs.builder()
* .engine("db2-se")
* .build());
*
* // Lookup the available instance classes for the engine in the region being operated in
* final var example = RdsFunctions.getOrderableDbInstance(GetOrderableDbInstanceArgs.builder()
* .engine(default_.engine())
* .engineVersion(default_.version())
* .licenseModel("bring-your-own-license")
* .storageType("gp3")
* .preferredInstanceClasses(
* "db.t3.small",
* "db.r6i.large",
* "db.m6i.large")
* .build());
*
* // The RDS Db2 instance resource requires licensing information. Create a new parameter group using the default paramater group as a source, and set license information.
* var exampleParameterGroup = new ParameterGroup("exampleParameterGroup", ParameterGroupArgs.builder()
* .name("db-db2-params")
* .family(default_.parameterGroupFamily())
* .parameters(
* ParameterGroupParameterArgs.builder()
* .applyMethod("immediate")
* .name("rds.ibm_customer_id")
* .value(0)
* .build(),
* ParameterGroupParameterArgs.builder()
* .applyMethod("immediate")
* .name("rds.ibm_site_id")
* .value(0)
* .build())
* .build());
*
* // Create the RDS Db2 instance, use the data sources defined to set attributes
* var exampleInstance = new Instance("exampleInstance", InstanceArgs.builder()
* .allocatedStorage(100)
* .backupRetentionPeriod(7)
* .dbName("test")
* .engine(example.applyValue(getOrderableDbInstanceResult -> getOrderableDbInstanceResult.engine()))
* .engineVersion(example.applyValue(getOrderableDbInstanceResult -> getOrderableDbInstanceResult.engineVersion()))
* .identifier("db2-instance-demo")
* .instanceClass(example.applyValue(getOrderableDbInstanceResult -> getOrderableDbInstanceResult.instanceClass()))
* .parameterGroupName(exampleParameterGroup.name())
* .password("avoid-plaintext-passwords")
* .username("test")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Storage Autoscaling
*
* To enable Storage Autoscaling with instances that support the feature, define the `max_allocated_storage` argument higher than the `allocated_storage` argument. This provider will automatically hide differences with the `allocated_storage` argument value if autoscaling occurs.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Instance("example", InstanceArgs.builder()
* .allocatedStorage(50)
* .maxAllocatedStorage(100)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Managed Master Passwords via Secrets Manager, default KMS Key
*
* > More information about RDS/Aurora Aurora integrates with Secrets Manager to manage master user passwords for your DB clusters can be found in the [RDS User Guide](https://aws.amazon.com/about-aws/whats-new/2022/12/amazon-rds-integration-aws-secrets-manager/) and [Aurora User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/rds-secrets-manager.html).
*
* You can specify the `manage_master_user_password` attribute to enable managing the master password with Secrets Manager. You can also update an existing cluster to use Secrets Manager by specify the `manage_master_user_password` attribute and removing the `password` attribute (removal is required).
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(10)
* .dbName("mydb")
* .engine("mysql")
* .engineVersion("8.0")
* .instanceClass("db.t3.micro")
* .manageMasterUserPassword(true)
* .username("foo")
* .parameterGroupName("default.mysql8.0")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Managed Master Passwords via Secrets Manager, specific KMS Key
*
* > More information about RDS/Aurora Aurora integrates with Secrets Manager to manage master user passwords for your DB clusters can be found in the [RDS User Guide](https://aws.amazon.com/about-aws/whats-new/2022/12/amazon-rds-integration-aws-secrets-manager/) and [Aurora User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/rds-secrets-manager.html).
*
* You can specify the `master_user_secret_kms_key_id` attribute to specify a specific KMS Key.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Key("example", KeyArgs.builder()
* .description("Example KMS Key")
* .build());
*
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(10)
* .dbName("mydb")
* .engine("mysql")
* .engineVersion("8.0")
* .instanceClass("db.t3.micro")
* .manageMasterUserPassword(true)
* .masterUserSecretKmsKeyId(example.keyId())
* .username("foo")
* .parameterGroupName("default.mysql8.0")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import DB Instances using the `identifier`. For example:
*
* ```sh
* $ pulumi import aws:rds/instance:Instance default mydb-rds-instance
* ```
*
*/
@ResourceType(type="aws:rds/instance:Instance")
public class Instance extends com.pulumi.resources.CustomResource {
/**
* Specifies the DNS address of the DB instance.
*
*/
@Export(name="address", refs={String.class}, tree="[0]")
private Output address;
/**
* @return Specifies the DNS address of the DB instance.
*
*/
public Output address() {
return this.address;
}
/**
* The allocated storage in gibibytes. If `max_allocated_storage` is configured, this argument represents the initial storage allocation and differences from the configuration will be ignored automatically when Storage Autoscaling occurs. If `replicate_source_db` is set, the value is ignored during the creation of the instance.
*
*/
@Export(name="allocatedStorage", refs={Integer.class}, tree="[0]")
private Output allocatedStorage;
/**
* @return The allocated storage in gibibytes. If `max_allocated_storage` is configured, this argument represents the initial storage allocation and differences from the configuration will be ignored automatically when Storage Autoscaling occurs. If `replicate_source_db` is set, the value is ignored during the creation of the instance.
*
*/
public Output allocatedStorage() {
return this.allocatedStorage;
}
/**
* Indicates that major version
* upgrades are allowed. Changing this parameter does not result in an outage and
* the change is asynchronously applied as soon as possible.
*
*/
@Export(name="allowMajorVersionUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowMajorVersionUpgrade;
/**
* @return Indicates that major version
* upgrades are allowed. Changing this parameter does not result in an outage and
* the change is asynchronously applied as soon as possible.
*
*/
public Output> allowMajorVersionUpgrade() {
return Codegen.optional(this.allowMajorVersionUpgrade);
}
/**
* Specifies whether any database modifications
* are applied immediately, or during the next maintenance window. Default is
* `false`. See [Amazon RDS Documentation for more
* information.](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Overview.DBInstance.Modifying.html)
*
*/
@Export(name="applyImmediately", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> applyImmediately;
/**
* @return Specifies whether any database modifications
* are applied immediately, or during the next maintenance window. Default is
* `false`. See [Amazon RDS Documentation for more
* information.](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Overview.DBInstance.Modifying.html)
*
*/
public Output> applyImmediately() {
return Codegen.optional(this.applyImmediately);
}
/**
* The ARN of the RDS instance.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the RDS instance.
*
*/
public Output arn() {
return this.arn;
}
/**
* Indicates that minor engine upgrades
* will be applied automatically to the DB instance during the maintenance window.
* Defaults to true.
*
*/
@Export(name="autoMinorVersionUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoMinorVersionUpgrade;
/**
* @return Indicates that minor engine upgrades
* will be applied automatically to the DB instance during the maintenance window.
* Defaults to true.
*
*/
public Output> autoMinorVersionUpgrade() {
return Codegen.optional(this.autoMinorVersionUpgrade);
}
/**
* The AZ for the RDS instance.
*
*/
@Export(name="availabilityZone", refs={String.class}, tree="[0]")
private Output availabilityZone;
/**
* @return The AZ for the RDS instance.
*
*/
public Output availabilityZone() {
return this.availabilityZone;
}
/**
* The days to retain backups for.
* Must be between `0` and `35`.
* Default is `0`.
* Must be greater than `0` if the database is used as a source for a [Read Replica][instance-replication],
* uses low-downtime updates,
* or will use [RDS Blue/Green deployments][blue-green].
*
*/
@Export(name="backupRetentionPeriod", refs={Integer.class}, tree="[0]")
private Output backupRetentionPeriod;
/**
* @return The days to retain backups for.
* Must be between `0` and `35`.
* Default is `0`.
* Must be greater than `0` if the database is used as a source for a [Read Replica][instance-replication],
* uses low-downtime updates,
* or will use [RDS Blue/Green deployments][blue-green].
*
*/
public Output backupRetentionPeriod() {
return this.backupRetentionPeriod;
}
/**
* Specifies where automated backups and manual snapshots are stored. Possible values are `region` (default) and `outposts`. See [Working with Amazon RDS on AWS Outposts](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/rds-on-outposts.html) for more information.
*
*/
@Export(name="backupTarget", refs={String.class}, tree="[0]")
private Output backupTarget;
/**
* @return Specifies where automated backups and manual snapshots are stored. Possible values are `region` (default) and `outposts`. See [Working with Amazon RDS on AWS Outposts](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/rds-on-outposts.html) for more information.
*
*/
public Output backupTarget() {
return this.backupTarget;
}
/**
* The daily time range (in UTC) during which automated backups are created if they are enabled.
* Example: "09:46-10:16". Must not overlap with `maintenance_window`.
*
*/
@Export(name="backupWindow", refs={String.class}, tree="[0]")
private Output backupWindow;
/**
* @return The daily time range (in UTC) during which automated backups are created if they are enabled.
* Example: "09:46-10:16". Must not overlap with `maintenance_window`.
*
*/
public Output backupWindow() {
return this.backupWindow;
}
/**
* Enables low-downtime updates using [RDS Blue/Green deployments][blue-green].
* See `blue_green_update` below.
*
*/
@Export(name="blueGreenUpdate", refs={InstanceBlueGreenUpdate.class}, tree="[0]")
private Output* @Nullable */ InstanceBlueGreenUpdate> blueGreenUpdate;
/**
* @return Enables low-downtime updates using [RDS Blue/Green deployments][blue-green].
* See `blue_green_update` below.
*
*/
public Output> blueGreenUpdate() {
return Codegen.optional(this.blueGreenUpdate);
}
/**
* The identifier of the CA certificate for the DB instance.
*
*/
@Export(name="caCertIdentifier", refs={String.class}, tree="[0]")
private Output caCertIdentifier;
/**
* @return The identifier of the CA certificate for the DB instance.
*
*/
public Output caCertIdentifier() {
return this.caCertIdentifier;
}
/**
* The character set name to use for DB encoding in Oracle and Microsoft SQL instances (collation).
* This can't be changed.
* See [Oracle Character Sets Supported in Amazon RDS](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.OracleCharacterSets.html) or
* [Server-Level Collation for Microsoft SQL Server](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.SQLServer.CommonDBATasks.Collation.html) for more information.
* Cannot be set with `replicate_source_db`, `restore_to_point_in_time`, `s3_import`, or `snapshot_identifier`.
*
*/
@Export(name="characterSetName", refs={String.class}, tree="[0]")
private Output characterSetName;
/**
* @return The character set name to use for DB encoding in Oracle and Microsoft SQL instances (collation).
* This can't be changed.
* See [Oracle Character Sets Supported in Amazon RDS](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.OracleCharacterSets.html) or
* [Server-Level Collation for Microsoft SQL Server](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.SQLServer.CommonDBATasks.Collation.html) for more information.
* Cannot be set with `replicate_source_db`, `restore_to_point_in_time`, `s3_import`, or `snapshot_identifier`.
*
*/
public Output characterSetName() {
return this.characterSetName;
}
/**
* Copy all Instance `tags` to snapshots. Default is `false`.
*
*/
@Export(name="copyTagsToSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> copyTagsToSnapshot;
/**
* @return Copy all Instance `tags` to snapshots. Default is `false`.
*
*/
public Output> copyTagsToSnapshot() {
return Codegen.optional(this.copyTagsToSnapshot);
}
/**
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance.
*
*/
@Export(name="customIamInstanceProfile", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customIamInstanceProfile;
/**
* @return The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance.
*
*/
public Output> customIamInstanceProfile() {
return Codegen.optional(this.customIamInstanceProfile);
}
/**
* Indicates whether to enable a customer-owned IP address (CoIP) for an RDS on Outposts DB instance. See [CoIP for RDS on Outposts](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/rds-on-outposts.html#rds-on-outposts.coip) for more information.
*
* > **NOTE:** Removing the `replicate_source_db` attribute from an existing RDS
* Replicate database managed by the provider will promote the database to a fully
* standalone database.
*
*/
@Export(name="customerOwnedIpEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> customerOwnedIpEnabled;
/**
* @return Indicates whether to enable a customer-owned IP address (CoIP) for an RDS on Outposts DB instance. See [CoIP for RDS on Outposts](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/rds-on-outposts.html#rds-on-outposts.coip) for more information.
*
* > **NOTE:** Removing the `replicate_source_db` attribute from an existing RDS
* Replicate database managed by the provider will promote the database to a fully
* standalone database.
*
*/
public Output> customerOwnedIpEnabled() {
return Codegen.optional(this.customerOwnedIpEnabled);
}
/**
* The name of the database to create when the DB instance is created. If this parameter is not specified, no database is created in the DB instance. Note that this does not apply for Oracle or SQL Server engines. See the [AWS documentation](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/create-db-instance.html) for more details on what applies for those engines. If you are providing an Oracle db name, it needs to be in all upper case. Cannot be specified for a replica.
*
*/
@Export(name="dbName", refs={String.class}, tree="[0]")
private Output dbName;
/**
* @return The name of the database to create when the DB instance is created. If this parameter is not specified, no database is created in the DB instance. Note that this does not apply for Oracle or SQL Server engines. See the [AWS documentation](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/create-db-instance.html) for more details on what applies for those engines. If you are providing an Oracle db name, it needs to be in all upper case. Cannot be specified for a replica.
*
*/
public Output dbName() {
return this.dbName;
}
/**
* Name of DB subnet group. DB instance will
* be created in the VPC associated with the DB subnet group. If unspecified, will
* be created in the `default` VPC, or in EC2 Classic, if available. When working
* with read replicas, it should be specified only if the source database
* specifies an instance in another AWS Region. See [DBSubnetGroupName in API
* action CreateDBInstanceReadReplica](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstanceReadReplica.html)
* for additional read replica constraints.
*
*/
@Export(name="dbSubnetGroupName", refs={String.class}, tree="[0]")
private Output dbSubnetGroupName;
/**
* @return Name of DB subnet group. DB instance will
* be created in the VPC associated with the DB subnet group. If unspecified, will
* be created in the `default` VPC, or in EC2 Classic, if available. When working
* with read replicas, it should be specified only if the source database
* specifies an instance in another AWS Region. See [DBSubnetGroupName in API
* action CreateDBInstanceReadReplica](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstanceReadReplica.html)
* for additional read replica constraints.
*
*/
public Output dbSubnetGroupName() {
return this.dbSubnetGroupName;
}
/**
* Use a dedicated log volume (DLV) for the DB instance. Requires Provisioned IOPS. See the [AWS documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_PIOPS.StorageTypes.html#USER_PIOPS.dlv) for more details.
*
*/
@Export(name="dedicatedLogVolume", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> dedicatedLogVolume;
/**
* @return Use a dedicated log volume (DLV) for the DB instance. Requires Provisioned IOPS. See the [AWS documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_PIOPS.StorageTypes.html#USER_PIOPS.dlv) for more details.
*
*/
public Output> dedicatedLogVolume() {
return Codegen.optional(this.dedicatedLogVolume);
}
/**
* Specifies whether to remove automated backups immediately after the DB instance is deleted. Default is `true`.
*
*/
@Export(name="deleteAutomatedBackups", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> deleteAutomatedBackups;
/**
* @return Specifies whether to remove automated backups immediately after the DB instance is deleted. Default is `true`.
*
*/
public Output> deleteAutomatedBackups() {
return Codegen.optional(this.deleteAutomatedBackups);
}
/**
* If the DB instance should have deletion protection enabled. The database can't be deleted when this value is set to `true`. The default is `false`.
*
*/
@Export(name="deletionProtection", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> deletionProtection;
/**
* @return If the DB instance should have deletion protection enabled. The database can't be deleted when this value is set to `true`. The default is `false`.
*
*/
public Output> deletionProtection() {
return Codegen.optional(this.deletionProtection);
}
/**
* The ID of the Directory Service Active Directory domain to create the instance in. Conflicts with `domain_fqdn`, `domain_ou`, `domain_auth_secret_arn` and a `domain_dns_ips`.
*
*/
@Export(name="domain", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> domain;
/**
* @return The ID of the Directory Service Active Directory domain to create the instance in. Conflicts with `domain_fqdn`, `domain_ou`, `domain_auth_secret_arn` and a `domain_dns_ips`.
*
*/
public Output> domain() {
return Codegen.optional(this.domain);
}
/**
* The ARN for the Secrets Manager secret with the self managed Active Directory credentials for the user joining the domain. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
@Export(name="domainAuthSecretArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> domainAuthSecretArn;
/**
* @return The ARN for the Secrets Manager secret with the self managed Active Directory credentials for the user joining the domain. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
public Output> domainAuthSecretArn() {
return Codegen.optional(this.domainAuthSecretArn);
}
/**
* The IPv4 DNS IP addresses of your primary and secondary self managed Active Directory domain controllers. Two IP addresses must be provided. If there isn't a secondary domain controller, use the IP address of the primary domain controller for both entries in the list. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
@Export(name="domainDnsIps", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> domainDnsIps;
/**
* @return The IPv4 DNS IP addresses of your primary and secondary self managed Active Directory domain controllers. Two IP addresses must be provided. If there isn't a secondary domain controller, use the IP address of the primary domain controller for both entries in the list. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
public Output>> domainDnsIps() {
return Codegen.optional(this.domainDnsIps);
}
/**
* The fully qualified domain name (FQDN) of the self managed Active Directory domain. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
@Export(name="domainFqdn", refs={String.class}, tree="[0]")
private Output domainFqdn;
/**
* @return The fully qualified domain name (FQDN) of the self managed Active Directory domain. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
public Output domainFqdn() {
return this.domainFqdn;
}
/**
* The name of the IAM role to be used when making API calls to the Directory Service. Conflicts with `domain_fqdn`, `domain_ou`, `domain_auth_secret_arn` and a `domain_dns_ips`.
*
*/
@Export(name="domainIamRoleName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> domainIamRoleName;
/**
* @return The name of the IAM role to be used when making API calls to the Directory Service. Conflicts with `domain_fqdn`, `domain_ou`, `domain_auth_secret_arn` and a `domain_dns_ips`.
*
*/
public Output> domainIamRoleName() {
return Codegen.optional(this.domainIamRoleName);
}
/**
* The self managed Active Directory organizational unit for your DB instance to join. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
@Export(name="domainOu", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> domainOu;
/**
* @return The self managed Active Directory organizational unit for your DB instance to join. Conflicts with `domain` and `domain_iam_role_name`.
*
*/
public Output> domainOu() {
return Codegen.optional(this.domainOu);
}
/**
* Set of log types to enable for exporting to CloudWatch logs. If omitted, no logs will be exported. For supported values, see the EnableCloudwatchLogsExports.member.N parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html).
*
*/
@Export(name="enabledCloudwatchLogsExports", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> enabledCloudwatchLogsExports;
/**
* @return Set of log types to enable for exporting to CloudWatch logs. If omitted, no logs will be exported. For supported values, see the EnableCloudwatchLogsExports.member.N parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html).
*
*/
public Output>> enabledCloudwatchLogsExports() {
return Codegen.optional(this.enabledCloudwatchLogsExports);
}
/**
* The connection endpoint in `address:port` format.
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return The connection endpoint in `address:port` format.
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* The database engine to use. For supported values, see the Engine parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html). Note that for Amazon Aurora instances the engine must match the DB cluster's engine'. For information on the difference between the available Aurora MySQL engines see [Comparison between Aurora MySQL 1 and Aurora MySQL 2](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/AuroraMySQL.Updates.20180206.html) in the Amazon RDS User Guide.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output engine;
/**
* @return The database engine to use. For supported values, see the Engine parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html). Note that for Amazon Aurora instances the engine must match the DB cluster's engine'. For information on the difference between the available Aurora MySQL engines see [Comparison between Aurora MySQL 1 and Aurora MySQL 2](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/AuroraMySQL.Updates.20180206.html) in the Amazon RDS User Guide.
*
*/
public Output engine() {
return this.engine;
}
/**
* The life cycle type for this DB instance. This setting applies only to RDS for MySQL and RDS for PostgreSQL. Valid values are `open-source-rds-extended-support`, `open-source-rds-extended-support-disabled`. Default value is `open-source-rds-extended-support`. [Using Amazon RDS Extended Support]: https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/extended-support.html
*
*/
@Export(name="engineLifecycleSupport", refs={String.class}, tree="[0]")
private Output engineLifecycleSupport;
/**
* @return The life cycle type for this DB instance. This setting applies only to RDS for MySQL and RDS for PostgreSQL. Valid values are `open-source-rds-extended-support`, `open-source-rds-extended-support-disabled`. Default value is `open-source-rds-extended-support`. [Using Amazon RDS Extended Support]: https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/extended-support.html
*
*/
public Output engineLifecycleSupport() {
return this.engineLifecycleSupport;
}
/**
* The engine version to use. If `auto_minor_version_upgrade` is enabled, you can provide a prefix of the version such as `8.0` (for `8.0.36`). The actual engine version used is returned in the attribute `engine_version_actual`, see Attribute Reference below. For supported values, see the EngineVersion parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html). Note that for Amazon Aurora instances the engine version must match the DB cluster's engine version'.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return The engine version to use. If `auto_minor_version_upgrade` is enabled, you can provide a prefix of the version such as `8.0` (for `8.0.36`). The actual engine version used is returned in the attribute `engine_version_actual`, see Attribute Reference below. For supported values, see the EngineVersion parameter in [API action CreateDBInstance](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html). Note that for Amazon Aurora instances the engine version must match the DB cluster's engine version'.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
/**
* The running version of the database.
*
*/
@Export(name="engineVersionActual", refs={String.class}, tree="[0]")
private Output engineVersionActual;
/**
* @return The running version of the database.
*
*/
public Output engineVersionActual() {
return this.engineVersionActual;
}
/**
* The name of your final DB snapshot
* when this DB instance is deleted. Must be provided if `skip_final_snapshot` is
* set to `false`. The value must begin with a letter, only contain alphanumeric characters and hyphens, and not end with a hyphen or contain two consecutive hyphens. Must not be provided when deleting a read replica.
*
*/
@Export(name="finalSnapshotIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> finalSnapshotIdentifier;
/**
* @return The name of your final DB snapshot
* when this DB instance is deleted. Must be provided if `skip_final_snapshot` is
* set to `false`. The value must begin with a letter, only contain alphanumeric characters and hyphens, and not end with a hyphen or contain two consecutive hyphens. Must not be provided when deleting a read replica.
*
*/
public Output> finalSnapshotIdentifier() {
return Codegen.optional(this.finalSnapshotIdentifier);
}
/**
* Specifies the ID that Amazon Route 53 assigns when you create a hosted zone.
*
*/
@Export(name="hostedZoneId", refs={String.class}, tree="[0]")
private Output hostedZoneId;
/**
* @return Specifies the ID that Amazon Route 53 assigns when you create a hosted zone.
*
*/
public Output hostedZoneId() {
return this.hostedZoneId;
}
/**
* Specifies whether mappings of AWS Identity and Access Management (IAM) accounts to database
* accounts is enabled.
*
*/
@Export(name="iamDatabaseAuthenticationEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> iamDatabaseAuthenticationEnabled;
/**
* @return Specifies whether mappings of AWS Identity and Access Management (IAM) accounts to database
* accounts is enabled.
*
*/
public Output> iamDatabaseAuthenticationEnabled() {
return Codegen.optional(this.iamDatabaseAuthenticationEnabled);
}
/**
* The name of the RDS instance, if omitted, this provider will assign a random, unique identifier. Required if `restore_to_point_in_time` is specified.
*
*/
@Export(name="identifier", refs={String.class}, tree="[0]")
private Output identifier;
/**
* @return The name of the RDS instance, if omitted, this provider will assign a random, unique identifier. Required if `restore_to_point_in_time` is specified.
*
*/
public Output identifier() {
return this.identifier;
}
/**
* Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
@Export(name="identifierPrefix", refs={String.class}, tree="[0]")
private Output identifierPrefix;
/**
* @return Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
public Output identifierPrefix() {
return this.identifierPrefix;
}
/**
* The instance type of the RDS instance.
*
*/
@Export(name="instanceClass", refs={String.class}, tree="[0]")
private Output instanceClass;
/**
* @return The instance type of the RDS instance.
*
*/
public Output instanceClass() {
return this.instanceClass;
}
/**
* The amount of provisioned IOPS. Setting this implies a
* storage_type of "io1" or "io2". Can only be set when `storage_type` is `"io1"`, `"io2` or `"gp3"`.
* Cannot be specified for gp3 storage if the `allocated_storage` value is below a per-`engine` threshold.
* See the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#gp3-storage) for details.
*
*/
@Export(name="iops", refs={Integer.class}, tree="[0]")
private Output iops;
/**
* @return The amount of provisioned IOPS. Setting this implies a
* storage_type of "io1" or "io2". Can only be set when `storage_type` is `"io1"`, `"io2` or `"gp3"`.
* Cannot be specified for gp3 storage if the `allocated_storage` value is below a per-`engine` threshold.
* See the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#gp3-storage) for details.
*
*/
public Output iops() {
return this.iops;
}
/**
* The ARN for the KMS encryption key. If creating an
* encrypted replica, set this to the destination KMS ARN.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return The ARN for the KMS encryption key. If creating an
* encrypted replica, set this to the destination KMS ARN.
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
/**
* The latest time, in UTC [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8), to which a database can be restored with point-in-time restore.
*
*/
@Export(name="latestRestorableTime", refs={String.class}, tree="[0]")
private Output latestRestorableTime;
/**
* @return The latest time, in UTC [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8), to which a database can be restored with point-in-time restore.
*
*/
public Output latestRestorableTime() {
return this.latestRestorableTime;
}
/**
* License model information for this DB instance. Valid values for this field are as follows:
* * RDS for MariaDB: `general-public-license`
* * RDS for Microsoft SQL Server: `license-included`
* * RDS for MySQL: `general-public-license`
* * RDS for Oracle: `bring-your-own-license | license-included`
* * RDS for PostgreSQL: `postgresql-license`
*
*/
@Export(name="licenseModel", refs={String.class}, tree="[0]")
private Output licenseModel;
/**
* @return License model information for this DB instance. Valid values for this field are as follows:
* * RDS for MariaDB: `general-public-license`
* * RDS for Microsoft SQL Server: `license-included`
* * RDS for MySQL: `general-public-license`
* * RDS for Oracle: `bring-your-own-license | license-included`
* * RDS for PostgreSQL: `postgresql-license`
*
*/
public Output licenseModel() {
return this.licenseModel;
}
/**
* Specifies the listener connection endpoint for SQL Server Always On. See endpoint below.
*
*/
@Export(name="listenerEndpoints", refs={List.class,InstanceListenerEndpoint.class}, tree="[0,1]")
private Output> listenerEndpoints;
/**
* @return Specifies the listener connection endpoint for SQL Server Always On. See endpoint below.
*
*/
public Output> listenerEndpoints() {
return this.listenerEndpoints;
}
/**
* The window to perform maintenance in.
* Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00". See [RDS
* Maintenance Window
* docs](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_UpgradeDBInstance.Maintenance.html#AdjustingTheMaintenanceWindow)
* for more information.
*
*/
@Export(name="maintenanceWindow", refs={String.class}, tree="[0]")
private Output maintenanceWindow;
/**
* @return The window to perform maintenance in.
* Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00". See [RDS
* Maintenance Window
* docs](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_UpgradeDBInstance.Maintenance.html#AdjustingTheMaintenanceWindow)
* for more information.
*
*/
public Output maintenanceWindow() {
return this.maintenanceWindow;
}
/**
* Set to true to allow RDS to manage the master user password in Secrets Manager. Cannot be set if `password` is provided.
*
*/
@Export(name="manageMasterUserPassword", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> manageMasterUserPassword;
/**
* @return Set to true to allow RDS to manage the master user password in Secrets Manager. Cannot be set if `password` is provided.
*
*/
public Output> manageMasterUserPassword() {
return Codegen.optional(this.manageMasterUserPassword);
}
/**
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN. If not specified, the default KMS key for your Amazon Web Services account is used.
*
*/
@Export(name="masterUserSecretKmsKeyId", refs={String.class}, tree="[0]")
private Output masterUserSecretKmsKeyId;
/**
* @return The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN. If not specified, the default KMS key for your Amazon Web Services account is used.
*
*/
public Output masterUserSecretKmsKeyId() {
return this.masterUserSecretKmsKeyId;
}
/**
* A block that specifies the master user secret. Only available when `manage_master_user_password` is set to true. Documented below.
*
*/
@Export(name="masterUserSecrets", refs={List.class,InstanceMasterUserSecret.class}, tree="[0,1]")
private Output> masterUserSecrets;
/**
* @return A block that specifies the master user secret. Only available when `manage_master_user_password` is set to true. Documented below.
*
*/
public Output> masterUserSecrets() {
return this.masterUserSecrets;
}
/**
* When configured, the upper limit to which Amazon RDS can automatically scale the storage of the DB instance. Configuring this will automatically ignore differences to `allocated_storage`. Must be greater than or equal to `allocated_storage` or `0` to disable Storage Autoscaling.
*
*/
@Export(name="maxAllocatedStorage", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxAllocatedStorage;
/**
* @return When configured, the upper limit to which Amazon RDS can automatically scale the storage of the DB instance. Configuring this will automatically ignore differences to `allocated_storage`. Must be greater than or equal to `allocated_storage` or `0` to disable Storage Autoscaling.
*
*/
public Output> maxAllocatedStorage() {
return Codegen.optional(this.maxAllocatedStorage);
}
/**
* The interval, in seconds, between points
* when Enhanced Monitoring metrics are collected for the DB instance. To disable
* collecting Enhanced Monitoring metrics, specify 0. The default is 0. Valid
* Values: 0, 1, 5, 10, 15, 30, 60.
*
*/
@Export(name="monitoringInterval", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> monitoringInterval;
/**
* @return The interval, in seconds, between points
* when Enhanced Monitoring metrics are collected for the DB instance. To disable
* collecting Enhanced Monitoring metrics, specify 0. The default is 0. Valid
* Values: 0, 1, 5, 10, 15, 30, 60.
*
*/
public Output> monitoringInterval() {
return Codegen.optional(this.monitoringInterval);
}
/**
* The ARN for the IAM role that permits RDS
* to send enhanced monitoring metrics to CloudWatch Logs. You can find more
* information on the [AWS
* Documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_Monitoring.html)
* what IAM permissions are needed to allow Enhanced Monitoring for RDS Instances.
*
*/
@Export(name="monitoringRoleArn", refs={String.class}, tree="[0]")
private Output monitoringRoleArn;
/**
* @return The ARN for the IAM role that permits RDS
* to send enhanced monitoring metrics to CloudWatch Logs. You can find more
* information on the [AWS
* Documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_Monitoring.html)
* what IAM permissions are needed to allow Enhanced Monitoring for RDS Instances.
*
*/
public Output monitoringRoleArn() {
return this.monitoringRoleArn;
}
/**
* Specifies if the RDS instance is multi-AZ
*
*/
@Export(name="multiAz", refs={Boolean.class}, tree="[0]")
private Output multiAz;
/**
* @return Specifies if the RDS instance is multi-AZ
*
*/
public Output multiAz() {
return this.multiAz;
}
/**
* @deprecated
* This property has been deprecated. Please use 'dbName' instead.
*
*/
@Deprecated /* This property has been deprecated. Please use 'dbName' instead. */
@Export(name="name", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> name;
public Output> name() {
return Codegen.optional(this.name);
}
/**
* The national character set is used in the NCHAR, NVARCHAR2, and NCLOB data types for Oracle instances. This can't be changed. See [Oracle Character Sets
* Supported in Amazon RDS](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.OracleCharacterSets.html).
*
*/
@Export(name="ncharCharacterSetName", refs={String.class}, tree="[0]")
private Output ncharCharacterSetName;
/**
* @return The national character set is used in the NCHAR, NVARCHAR2, and NCLOB data types for Oracle instances. This can't be changed. See [Oracle Character Sets
* Supported in Amazon RDS](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Appendix.OracleCharacterSets.html).
*
*/
public Output ncharCharacterSetName() {
return this.ncharCharacterSetName;
}
/**
* The network type of the DB instance. Valid values: `IPV4`, `DUAL`.
*
*/
@Export(name="networkType", refs={String.class}, tree="[0]")
private Output networkType;
/**
* @return The network type of the DB instance. Valid values: `IPV4`, `DUAL`.
*
*/
public Output networkType() {
return this.networkType;
}
/**
* Name of the DB option group to associate.
*
*/
@Export(name="optionGroupName", refs={String.class}, tree="[0]")
private Output optionGroupName;
/**
* @return Name of the DB option group to associate.
*
*/
public Output optionGroupName() {
return this.optionGroupName;
}
/**
* Name of the DB parameter group to associate.
*
*/
@Export(name="parameterGroupName", refs={String.class}, tree="[0]")
private Output parameterGroupName;
/**
* @return Name of the DB parameter group to associate.
*
*/
public Output parameterGroupName() {
return this.parameterGroupName;
}
/**
* (Required unless `manage_master_user_password` is set to true or unless a `snapshot_identifier` or `replicate_source_db`
* is provided or `manage_master_user_password` is set.) Password for the master DB user. Note that this may show up in
* logs, and it will be stored in the state file. Cannot be set if `manage_master_user_password` is set to `true`.
*
*/
@Export(name="password", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> password;
/**
* @return (Required unless `manage_master_user_password` is set to true or unless a `snapshot_identifier` or `replicate_source_db`
* is provided or `manage_master_user_password` is set.) Password for the master DB user. Note that this may show up in
* logs, and it will be stored in the state file. Cannot be set if `manage_master_user_password` is set to `true`.
*
*/
public Output> password() {
return Codegen.optional(this.password);
}
/**
* Specifies whether Performance Insights are enabled. Defaults to false.
*
*/
@Export(name="performanceInsightsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> performanceInsightsEnabled;
/**
* @return Specifies whether Performance Insights are enabled. Defaults to false.
*
*/
public Output> performanceInsightsEnabled() {
return Codegen.optional(this.performanceInsightsEnabled);
}
/**
* The ARN for the KMS key to encrypt Performance Insights data. When specifying `performance_insights_kms_key_id`, `performance_insights_enabled` needs to be set to true. Once KMS key is set, it can never be changed.
*
*/
@Export(name="performanceInsightsKmsKeyId", refs={String.class}, tree="[0]")
private Output performanceInsightsKmsKeyId;
/**
* @return The ARN for the KMS key to encrypt Performance Insights data. When specifying `performance_insights_kms_key_id`, `performance_insights_enabled` needs to be set to true. Once KMS key is set, it can never be changed.
*
*/
public Output performanceInsightsKmsKeyId() {
return this.performanceInsightsKmsKeyId;
}
/**
* Amount of time in days to retain Performance Insights data. Valid values are `7`, `731` (2 years) or a multiple of `31`. When specifying `performance_insights_retention_period`, `performance_insights_enabled` needs to be set to true. Defaults to '7'.
*
*/
@Export(name="performanceInsightsRetentionPeriod", refs={Integer.class}, tree="[0]")
private Output performanceInsightsRetentionPeriod;
/**
* @return Amount of time in days to retain Performance Insights data. Valid values are `7`, `731` (2 years) or a multiple of `31`. When specifying `performance_insights_retention_period`, `performance_insights_enabled` needs to be set to true. Defaults to '7'.
*
*/
public Output performanceInsightsRetentionPeriod() {
return this.performanceInsightsRetentionPeriod;
}
/**
* The port on which the DB accepts connections.
*
*/
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output port;
/**
* @return The port on which the DB accepts connections.
*
*/
public Output port() {
return this.port;
}
/**
* Bool to control if instance is publicly
* accessible. Default is `false`.
*
*/
@Export(name="publiclyAccessible", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> publiclyAccessible;
/**
* @return Bool to control if instance is publicly
* accessible. Default is `false`.
*
*/
public Output> publiclyAccessible() {
return Codegen.optional(this.publiclyAccessible);
}
/**
* Specifies whether the replica is in either `mounted` or `open-read-only` mode. This attribute
* is only supported by Oracle instances. Oracle replicas operate in `open-read-only` mode unless otherwise specified. See [Working with Oracle Read Replicas](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/oracle-read-replicas.html) for more information.
*
*/
@Export(name="replicaMode", refs={String.class}, tree="[0]")
private Output replicaMode;
/**
* @return Specifies whether the replica is in either `mounted` or `open-read-only` mode. This attribute
* is only supported by Oracle instances. Oracle replicas operate in `open-read-only` mode unless otherwise specified. See [Working with Oracle Read Replicas](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/oracle-read-replicas.html) for more information.
*
*/
public Output replicaMode() {
return this.replicaMode;
}
@Export(name="replicas", refs={List.class,String.class}, tree="[0,1]")
private Output> replicas;
public Output> replicas() {
return this.replicas;
}
/**
* Specifies that this resource is a Replicate
* database, and to use this value as the source database. This correlates to the
* `identifier` of another Amazon RDS Database to replicate (if replicating within
* a single region) or ARN of the Amazon RDS Database to replicate (if replicating
* cross-region). Note that if you are
* creating a cross-region replica of an encrypted database you will also need to
* specify a `kms_key_id`. See [DB Instance Replication][instance-replication] and [Working with
* PostgreSQL and MySQL Read Replicas](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_ReadRepl.html)
* for more information on using Replication.
*
*/
@Export(name="replicateSourceDb", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> replicateSourceDb;
/**
* @return Specifies that this resource is a Replicate
* database, and to use this value as the source database. This correlates to the
* `identifier` of another Amazon RDS Database to replicate (if replicating within
* a single region) or ARN of the Amazon RDS Database to replicate (if replicating
* cross-region). Note that if you are
* creating a cross-region replica of an encrypted database you will also need to
* specify a `kms_key_id`. See [DB Instance Replication][instance-replication] and [Working with
* PostgreSQL and MySQL Read Replicas](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_ReadRepl.html)
* for more information on using Replication.
*
*/
public Output> replicateSourceDb() {
return Codegen.optional(this.replicateSourceDb);
}
/**
* The RDS Resource ID of this instance.
*
*/
@Export(name="resourceId", refs={String.class}, tree="[0]")
private Output resourceId;
/**
* @return The RDS Resource ID of this instance.
*
*/
public Output resourceId() {
return this.resourceId;
}
/**
* A configuration block for restoring a DB instance to an arbitrary point in time. Requires the `identifier` argument to be set with the name of the new DB instance to be created. See Restore To Point In Time below for details.
*
*/
@Export(name="restoreToPointInTime", refs={InstanceRestoreToPointInTime.class}, tree="[0]")
private Output* @Nullable */ InstanceRestoreToPointInTime> restoreToPointInTime;
/**
* @return A configuration block for restoring a DB instance to an arbitrary point in time. Requires the `identifier` argument to be set with the name of the new DB instance to be created. See Restore To Point In Time below for details.
*
*/
public Output> restoreToPointInTime() {
return Codegen.optional(this.restoreToPointInTime);
}
/**
* Restore from a Percona Xtrabackup in S3. See [Importing Data into an Amazon RDS MySQL DB Instance](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/MySQL.Procedural.Importing.html)
*
*/
@Export(name="s3Import", refs={InstanceS3Import.class}, tree="[0]")
private Output* @Nullable */ InstanceS3Import> s3Import;
/**
* @return Restore from a Percona Xtrabackup in S3. See [Importing Data into an Amazon RDS MySQL DB Instance](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/MySQL.Procedural.Importing.html)
*
*/
public Output> s3Import() {
return Codegen.optional(this.s3Import);
}
/**
* Determines whether a final DB snapshot is
* created before the DB instance is deleted. If true is specified, no DBSnapshot
* is created. If false is specified, a DB snapshot is created before the DB
* instance is deleted, using the value from `final_snapshot_identifier`. Default
* is `false`.
*
*/
@Export(name="skipFinalSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipFinalSnapshot;
/**
* @return Determines whether a final DB snapshot is
* created before the DB instance is deleted. If true is specified, no DBSnapshot
* is created. If false is specified, a DB snapshot is created before the DB
* instance is deleted, using the value from `final_snapshot_identifier`. Default
* is `false`.
*
*/
public Output> skipFinalSnapshot() {
return Codegen.optional(this.skipFinalSnapshot);
}
/**
* Specifies whether or not to create this
* database from a snapshot. This correlates to the snapshot ID you'd find in the
* RDS console, e.g: rds:production-2015-06-26-06-05.
*
*/
@Export(name="snapshotIdentifier", refs={String.class}, tree="[0]")
private Output snapshotIdentifier;
/**
* @return Specifies whether or not to create this
* database from a snapshot. This correlates to the snapshot ID you'd find in the
* RDS console, e.g: rds:production-2015-06-26-06-05.
*
*/
public Output snapshotIdentifier() {
return this.snapshotIdentifier;
}
/**
* The RDS instance status.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The RDS instance status.
*
*/
public Output status() {
return this.status;
}
/**
* Specifies whether the DB instance is
* encrypted. Note that if you are creating a cross-region read replica this field
* is ignored and you should instead declare `kms_key_id` with a valid ARN. The
* default is `false` if not specified.
*
*/
@Export(name="storageEncrypted", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> storageEncrypted;
/**
* @return Specifies whether the DB instance is
* encrypted. Note that if you are creating a cross-region read replica this field
* is ignored and you should instead declare `kms_key_id` with a valid ARN. The
* default is `false` if not specified.
*
*/
public Output> storageEncrypted() {
return Codegen.optional(this.storageEncrypted);
}
/**
* The storage throughput value for the DB instance. Can only be set when `storage_type` is `"gp3"`. Cannot be specified if the `allocated_storage` value is below a per-`engine` threshold. See the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#gp3-storage) for details.
*
*/
@Export(name="storageThroughput", refs={Integer.class}, tree="[0]")
private Output storageThroughput;
/**
* @return The storage throughput value for the DB instance. Can only be set when `storage_type` is `"gp3"`. Cannot be specified if the `allocated_storage` value is below a per-`engine` threshold. See the [RDS User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Storage.html#gp3-storage) for details.
*
*/
public Output storageThroughput() {
return this.storageThroughput;
}
/**
* One of "standard" (magnetic), "gp2" (general
* purpose SSD), "gp3" (general purpose SSD that needs `iops` independently)
* "io1" (provisioned IOPS SSD) or "io2" (block express storage provisioned IOPS
* SSD). The default is "io1" if `iops` is specified, "gp2" if not.
*
*/
@Export(name="storageType", refs={String.class}, tree="[0]")
private Output storageType;
/**
* @return One of "standard" (magnetic), "gp2" (general
* purpose SSD), "gp3" (general purpose SSD that needs `iops` independently)
* "io1" (provisioned IOPS SSD) or "io2" (block express storage provisioned IOPS
* SSD). The default is "io1" if `iops` is specified, "gp2" if not.
*
*/
public Output storageType() {
return this.storageType;
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy