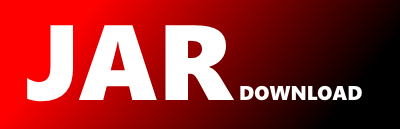
com.pulumi.aws.autoscaling.inputs.GroupMixedInstancesPolicyInstancesDistributionArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.autoscaling.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GroupMixedInstancesPolicyInstancesDistributionArgs extends com.pulumi.resources.ResourceArgs {
public static final GroupMixedInstancesPolicyInstancesDistributionArgs Empty = new GroupMixedInstancesPolicyInstancesDistributionArgs();
/**
* Strategy to use when launching on-demand instances. Valid values: `prioritized`, `lowest-price`. Default: `prioritized`.
*
*/
@Import(name="onDemandAllocationStrategy")
private @Nullable Output onDemandAllocationStrategy;
/**
* @return Strategy to use when launching on-demand instances. Valid values: `prioritized`, `lowest-price`. Default: `prioritized`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy