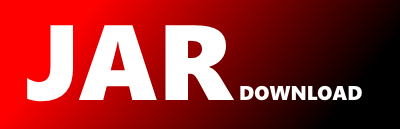
com.pulumi.aws.cfg.DeliveryChannelArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cfg;
import com.pulumi.aws.cfg.inputs.DeliveryChannelSnapshotDeliveryPropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DeliveryChannelArgs extends com.pulumi.resources.ResourceArgs {
public static final DeliveryChannelArgs Empty = new DeliveryChannelArgs();
/**
* The name of the delivery channel. Defaults to `default`. Changing it recreates the resource.
*
*/
@Import(name="name")
private @Nullable Output name;
/**
* @return The name of the delivery channel. Defaults to `default`. Changing it recreates the resource.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy