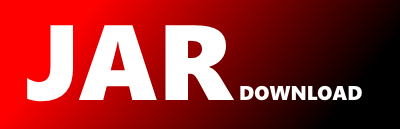
com.pulumi.aws.chatbot.inputs.SlackChannelConfigurationState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.chatbot.inputs;
import com.pulumi.aws.chatbot.inputs.SlackChannelConfigurationTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SlackChannelConfigurationState extends com.pulumi.resources.ResourceArgs {
public static final SlackChannelConfigurationState Empty = new SlackChannelConfigurationState();
/**
* ARN of the Slack channel configuration.
*
*/
@Import(name="chatConfigurationArn")
private @Nullable Output chatConfigurationArn;
/**
* @return ARN of the Slack channel configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy