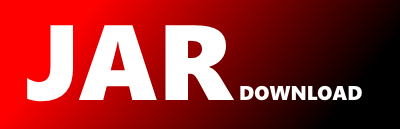
com.pulumi.aws.directoryservice.TrustArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directoryservice;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TrustArgs extends com.pulumi.resources.ResourceArgs {
public static final TrustArgs Empty = new TrustArgs();
/**
* Set of IPv4 addresses for the DNS server associated with the remote Directory.
* Can contain between 1 and 4 values.
*
*/
@Import(name="conditionalForwarderIpAddrs")
private @Nullable Output> conditionalForwarderIpAddrs;
/**
* @return Set of IPv4 addresses for the DNS server associated with the remote Directory.
* Can contain between 1 and 4 values.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy