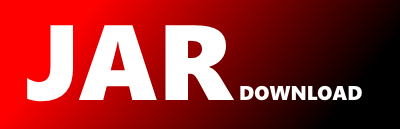
com.pulumi.aws.efs.inputs.MountTargetState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.efs.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MountTargetState extends com.pulumi.resources.ResourceArgs {
public static final MountTargetState Empty = new MountTargetState();
/**
* The unique and consistent identifier of the Availability Zone (AZ) that the mount target resides in.
*
*/
@Import(name="availabilityZoneId")
private @Nullable Output availabilityZoneId;
/**
* @return The unique and consistent identifier of the Availability Zone (AZ) that the mount target resides in.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy